Description of the Problem In this assignment, you are required to a new grading system which, includes the menu system to allow the lecturer to input the students' marks for different subjects. The system is able to calculate the grade and CGPA for the students. Store and persist the students' details in the file (students.txt) for further processing. Retrieve the student records from the file whenever the user wants to manipulate the student records such as adding an additional new subject and mark to the system. You have to use the list or dictionary or both in storing the student records in the memory. The student file structure is shown as follows: [Student Id],[Name]. [Subject! Mark], [Subject2 Mark], [Subject3 Mark] Example S20001, Ow Yi Han, CSC1101 86, csc1102 75, csc1103 60 The above example illustrates that the student id is S20001 with the name "Peter" and has three subjects (CSC1101, CSC1102, csc1103) with different marks.
Description of the Problem In this assignment, you are required to a new grading system which, includes the menu system to allow the lecturer to input the students' marks for different subjects. The system is able to calculate the grade and CGPA for the students. Store and persist the students' details in the file (students.txt) for further processing. Retrieve the student records from the file whenever the user wants to manipulate the student records such as adding an additional new subject and mark to the system. You have to use the list or dictionary or both in storing the student records in the memory. The student file structure is shown as follows: [Student Id],[Name]. [Subject! Mark], [Subject2 Mark], [Subject3 Mark] Example S20001, Ow Yi Han, CSC1101 86, csc1102 75, csc1103 60 The above example illustrates that the student id is S20001 with the name "Peter" and has three subjects (CSC1101, CSC1102, csc1103) with different marks.
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter7: File Handling And Applications
Section: Chapter Questions
Problem 1GZ
Related questions
Question
100%
python
text files
student.txt = P21013088,Kylie Tan Zen Dong,CSC1101 86,CSC1102 75
P21013177,Tan Soo Hong,CSC1101 76,CSC1103 76,CSC1104 92,CSC1105 88
P21013200,Peh Jia Xuan,CSC1101 88,CSC1102 80,CSC1103 90,CSC1106 75
subjects.txt = CSC1101,Computer Fundamental
CSC1102,Computer Architecture
CSC1103,Fundamentals of Databases
CSC1104,Networking
CSC1105,Programming with Python
CSC1106,Design Thinking
CSC1107,Human Computer Interaction
CSC1108,Programming with R
CSC1109,Systems Analysis and Design
CSC1110,Machine Learning
CSC1111,Data Structure and Algorithms
CSC1112,Big Data Architecture
CSC1113,Fundamentals of Data Science
![Part 4: Editing Result
If the user selects option 4, prompt the user to enter the student id. If the id is found in the
database, display the full details of the student including the listing of the subjects with marks.
For example:
Enter user id: P21013088
Student Name: [Kylie Tan Zen Dong]
1) CSC1101-Computer Fundamental (86 %)
2) CSC1102-Computer Architecture (75%)
3) Exit
Enter subject option: 2
Enter new mark for CSC1102: 78
Save the record? [y/n]:
Prompt the user to enter the option and user is required to enter a new mark for the selected
subject and save the file upon the save confirmation.
Part 5: Deleting Result
If the user selects option 3, prompt the user to enter the student id. If the id is found in the
database, display the full details of the students and request for the deletion confirmation. The
student record should be deleted permanently from the file upon y/Y being entered by the user.
For example:
Enter user id: $20001
Student Name: [Ow Yi Han]
Subject Code
CSC1101
csc1102
Subject Name
Computer Fundamental
Computer Architecture
Mark
86
75
Grade GPA
A
A-
4.00
3.67
CGPA: 3.84
Delete this record? [y/n]:
Structure your solution using functions that accept parameters and return values where appropriate. Use
whitespace and indentation properly. Limit lines to 80 characters. Give meaningful names to
functions/variables, and follow Python's naming standards. Localize variables. Put descriptive
comments at the start of your program and each function. Since this program has longer functions, also
put brief comments inside functions on complex sections of code.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1ef30170-7f46-40c4-a1ee-7333021f101b%2Fd262d38a-c676-4f1e-ac54-829de1bbb7d0%2Fvpvrnyq_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Part 4: Editing Result
If the user selects option 4, prompt the user to enter the student id. If the id is found in the
database, display the full details of the student including the listing of the subjects with marks.
For example:
Enter user id: P21013088
Student Name: [Kylie Tan Zen Dong]
1) CSC1101-Computer Fundamental (86 %)
2) CSC1102-Computer Architecture (75%)
3) Exit
Enter subject option: 2
Enter new mark for CSC1102: 78
Save the record? [y/n]:
Prompt the user to enter the option and user is required to enter a new mark for the selected
subject and save the file upon the save confirmation.
Part 5: Deleting Result
If the user selects option 3, prompt the user to enter the student id. If the id is found in the
database, display the full details of the students and request for the deletion confirmation. The
student record should be deleted permanently from the file upon y/Y being entered by the user.
For example:
Enter user id: $20001
Student Name: [Ow Yi Han]
Subject Code
CSC1101
csc1102
Subject Name
Computer Fundamental
Computer Architecture
Mark
86
75
Grade GPA
A
A-
4.00
3.67
CGPA: 3.84
Delete this record? [y/n]:
Structure your solution using functions that accept parameters and return values where appropriate. Use
whitespace and indentation properly. Limit lines to 80 characters. Give meaningful names to
functions/variables, and follow Python's naming standards. Localize variables. Put descriptive
comments at the start of your program and each function. Since this program has longer functions, also
put brief comments inside functions on complex sections of code.
![Description of the Problem
In this assignment, you are required to a new grading system which, includes the menu system
to allow the lecturer to input the students' marks for different subjects. The system is able to
calculate the grade and CGPA for the students. Store and persist the students' details in the file
(students.txt) for further processing. Retrieve the student records from the file whenever
the user wants to manipulate the student records such as adding an additional new subject and
mark to the system. You have to use the list or dictionary or both in storing the student records
in the memory. The student file structure is shown as follows:
[Student Id], [Name].[Subject 1 Mark], [Subject2 Mark], [Subject3 Mark]
Example
S20001, Ow Yi Han, CSC1101 86, CSC1102 75, CSC1103 60
The above example illustrates that the student id is S20001 with the name "Peter" and has
three subjects (CSC1101, CSC1102, CSC1103) with different marks.
Part 1: Create Menu System
Create a simple menu for the user selection. Your program should have a loop and continue to
ask for the option until the exit option is selected.
[Grading System]
1) Add New Mark
(2) Print Results
3) Edit Results
4) Delete Results.
5) Exit
Enter Option:
Part 2: Adding New Mark
If the user selects option 1, prompt the user to enter the student id. If the student id is found in
the students.txt, retrieve the existing records and display them to the user. Then, prompt the
user to enter a new mark for a particular subject. You should retrieve the subject details from
the subjects.txt file. Provide necessary validation for the student id and subject code. All the
entered details should save to the students.txt upon the save confirmation. For example:
Enter user id: P21013088
Student Name: [Kylie Tan Zen Dong].
Subject Code
Subject Name
‒‒‒‒‒‒
CSC1101
CSC1102
Enter subject code: CSC1103
Subject: [Fundamental of Database]
Enter subject mark: 81
Save Record? [y/n]:
Computer Fundamental
Computer Architecture
Enter user id: P21013088
Student Name: [Kylie Tan Zen Dong]
Subject Code
Subject Name
CSC1101
CSC1102
Part 3: Printing Result
If the user selects option 2, prompt the user to enter the student id. Retrieve the student details
and print out the output with GPA for each subject and CGPA. Provide an option for printing
the result to a file (the default file name is student id). Use Table I as a guideline to determine
the grade and GPA values. For example:
Table 1: Grade and GPA
50-54
45-49
40-44
<40
Mark Range Grade
80-100
75-79
70-74
65-69
60-64
55-59
A
A-
Save the result slip to P21013088.txt? [y/n]:
Mark
Computer Fundamental. 86
Computer Architecture 75
86
75
B+
B
B-
C+
C
C-
D
F
GPA
4
3.67
3.33
3.00
2.67
2.33
2.00
1.67
1.33
1.00
Mark
Grade
A
B+
A
Grade GPA
4.00
3.67
CGPA: 3.84](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1ef30170-7f46-40c4-a1ee-7333021f101b%2Fd262d38a-c676-4f1e-ac54-829de1bbb7d0%2F3cy1vy9_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Description of the Problem
In this assignment, you are required to a new grading system which, includes the menu system
to allow the lecturer to input the students' marks for different subjects. The system is able to
calculate the grade and CGPA for the students. Store and persist the students' details in the file
(students.txt) for further processing. Retrieve the student records from the file whenever
the user wants to manipulate the student records such as adding an additional new subject and
mark to the system. You have to use the list or dictionary or both in storing the student records
in the memory. The student file structure is shown as follows:
[Student Id], [Name].[Subject 1 Mark], [Subject2 Mark], [Subject3 Mark]
Example
S20001, Ow Yi Han, CSC1101 86, CSC1102 75, CSC1103 60
The above example illustrates that the student id is S20001 with the name "Peter" and has
three subjects (CSC1101, CSC1102, CSC1103) with different marks.
Part 1: Create Menu System
Create a simple menu for the user selection. Your program should have a loop and continue to
ask for the option until the exit option is selected.
[Grading System]
1) Add New Mark
(2) Print Results
3) Edit Results
4) Delete Results.
5) Exit
Enter Option:
Part 2: Adding New Mark
If the user selects option 1, prompt the user to enter the student id. If the student id is found in
the students.txt, retrieve the existing records and display them to the user. Then, prompt the
user to enter a new mark for a particular subject. You should retrieve the subject details from
the subjects.txt file. Provide necessary validation for the student id and subject code. All the
entered details should save to the students.txt upon the save confirmation. For example:
Enter user id: P21013088
Student Name: [Kylie Tan Zen Dong].
Subject Code
Subject Name
‒‒‒‒‒‒
CSC1101
CSC1102
Enter subject code: CSC1103
Subject: [Fundamental of Database]
Enter subject mark: 81
Save Record? [y/n]:
Computer Fundamental
Computer Architecture
Enter user id: P21013088
Student Name: [Kylie Tan Zen Dong]
Subject Code
Subject Name
CSC1101
CSC1102
Part 3: Printing Result
If the user selects option 2, prompt the user to enter the student id. Retrieve the student details
and print out the output with GPA for each subject and CGPA. Provide an option for printing
the result to a file (the default file name is student id). Use Table I as a guideline to determine
the grade and GPA values. For example:
Table 1: Grade and GPA
50-54
45-49
40-44
<40
Mark Range Grade
80-100
75-79
70-74
65-69
60-64
55-59
A
A-
Save the result slip to P21013088.txt? [y/n]:
Mark
Computer Fundamental. 86
Computer Architecture 75
86
75
B+
B
B-
C+
C
C-
D
F
GPA
4
3.67
3.33
3.00
2.67
2.33
2.00
1.67
1.33
1.00
Mark
Grade
A
B+
A
Grade GPA
4.00
3.67
CGPA: 3.84
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
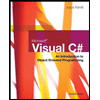
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
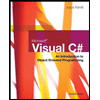
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,