Design and implement a class named CanadianAddress. The class contains: • The four private String type data fields named streetAddress, city, province, and postalCode that represent a Canadian address. (Note: You must use the given data field names.) • A no-arg constructor that creates a CanadianAddress object with null values for all the data fields. (Hint: Data fields have default values, null for String type.) • A constructor that constructs a CanadianAddress object with specified streetAddress, city, province, and postalCode. You must use the following header for this constructor with the exact parameter names: CanadianAddress (String street Address, String city, String province, String postalCode) • The getter and setter methods for all the four data fields. • A method named printAddress() that prints out the address. Check the sample run for expected format. Note that this method should print out the address content only (two nes). • A method named equalAddresses(CanadianAddress address) that returns true if address has the same content with this object and returns false otherwise. Write a test class named TestCanadianAddress that performs the followings in the given order: • Creates two CanadianAddress objects, using new CanadianAddress() and new CanadianAddress("923 Robie St.", "Halifax", "Nova Scotia", "B3H 3C3"). Name the reference variable to the first address as testAddress and the second as SMUAddress. • Print out the two addresses. Check the sample run for expected format. • Check if testAddress has the same content with SMUAddress and print out the result. Check the sample run for expected format. • Invoke the setter methods to update the testAddress to "923 Robie St.", "Halifax", "Nova Scotia", "B3H 3C3" for the four data fields respectively. • Print out the testAddress. Check the sample run for expected format. • Check if testAddress has the same content with SMUAddress and print out the result. Check the sample run for expected format.
Design and implement a class named CanadianAddress. The class contains: • The four private String type data fields named streetAddress, city, province, and postalCode that represent a Canadian address. (Note: You must use the given data field names.) • A no-arg constructor that creates a CanadianAddress object with null values for all the data fields. (Hint: Data fields have default values, null for String type.) • A constructor that constructs a CanadianAddress object with specified streetAddress, city, province, and postalCode. You must use the following header for this constructor with the exact parameter names: CanadianAddress (String street Address, String city, String province, String postalCode) • The getter and setter methods for all the four data fields. • A method named printAddress() that prints out the address. Check the sample run for expected format. Note that this method should print out the address content only (two nes). • A method named equalAddresses(CanadianAddress address) that returns true if address has the same content with this object and returns false otherwise. Write a test class named TestCanadianAddress that performs the followings in the given order: • Creates two CanadianAddress objects, using new CanadianAddress() and new CanadianAddress("923 Robie St.", "Halifax", "Nova Scotia", "B3H 3C3"). Name the reference variable to the first address as testAddress and the second as SMUAddress. • Print out the two addresses. Check the sample run for expected format. • Check if testAddress has the same content with SMUAddress and print out the result. Check the sample run for expected format. • Invoke the setter methods to update the testAddress to "923 Robie St.", "Halifax", "Nova Scotia", "B3H 3C3" for the four data fields respectively. • Print out the testAddress. Check the sample run for expected format. • Check if testAddress has the same content with SMUAddress and print out the result. Check the sample run for expected format.
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter11: More Object-oriented Programming Concepts
Section: Chapter Questions
Problem 3GZ
Related questions
Question
Write the code in java and please dont plagarise and dont copy from other sources or use anything. Do what in the question says. Thank you.
![Here is the sample run:
The testAddress is created as:
null, null,
null null, Canada
The SMUAddress is created as:
923 Robie St., Halifax,
Nova Scotia B3H 3C3, Canada
The testAddress is equal to SMUAddress? false
The testAddress is updated as:
923 Robie St., Halifax,
Nova Scotia B3H 3C3, Canada
The testAddress is equal to SMUAddress? true
Hint: Write both classes in a single source code file. The test class with main() method
should be declared as primary class (i.e., public class). You will have the following
template:
public class TestCanadianAddress{
public static void main (String[] args) {
// Test the CanadianAddress class as instructed
}
}
class CanadianAddress{ // not public
// Design the class as instructed
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F83cebb0c-db38-4f0e-a0d3-5fd209e24564%2F82b8a53a-0029-4817-aa0f-537c9dfd88c9%2F52o40s_processed.png&w=3840&q=75)
Transcribed Image Text:Here is the sample run:
The testAddress is created as:
null, null,
null null, Canada
The SMUAddress is created as:
923 Robie St., Halifax,
Nova Scotia B3H 3C3, Canada
The testAddress is equal to SMUAddress? false
The testAddress is updated as:
923 Robie St., Halifax,
Nova Scotia B3H 3C3, Canada
The testAddress is equal to SMUAddress? true
Hint: Write both classes in a single source code file. The test class with main() method
should be declared as primary class (i.e., public class). You will have the following
template:
public class TestCanadianAddress{
public static void main (String[] args) {
// Test the CanadianAddress class as instructed
}
}
class CanadianAddress{ // not public
// Design the class as instructed
}
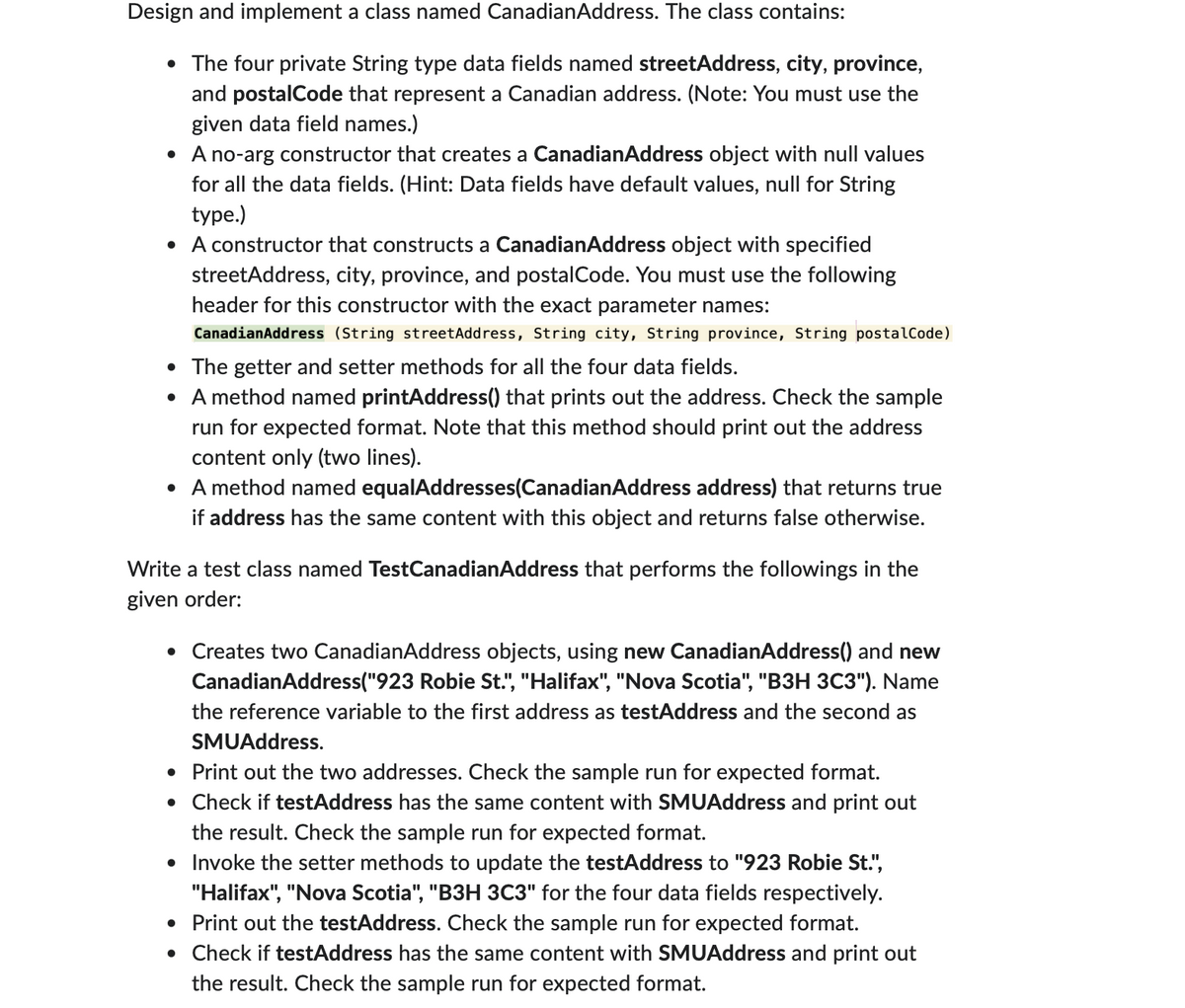
Transcribed Image Text:Design and implement a class named CanadianAddress. The class contains:
• The four private String type data fields named streetAddress, city, province,
and postalCode that represent a Canadian address. (Note: You must use the
given data field names.)
• A no-arg constructor that creates a CanadianAddress object with null values
for all the data fields. (Hint: Data fields have default values, null for String
type.)
• A constructor that constructs a CanadianAddress object with specified
streetAddress, city, province, and postalCode. You must use the following
header for this constructor with the exact parameter names:
CanadianAddress (String streetAddress, String city, String province, String postalCode)
• The getter and setter methods for all the four data fields.
• A method named printAddress() that prints out the address. Check the sample
run for expected format. Note that this method should print out the address
content only (two lines).
• A method named equalAddresses(Canadian Address address) that returns true
if address has the same content with this object and returns false otherwise.
Write a test class named TestCanadianAddress that performs the followings in the
given order:
• Creates two CanadianAddress objects, using new CanadianAddress() and new
CanadianAddress("923 Robie St.", "Halifax", "Nova Scotia", "B3H 3C3"). Name
the reference variable to the first address as testAddress and the second as
SMUAddress.
• Print out the two addresses. Check the sample run for expected format.
• Check if testAddress has the same content with SMUAddress and print out
the result. Check the sample run for expected format.
• Invoke the setter methods to update the testAddress to "923 Robie St.",
"Halifax", "Nova Scotia", "B3H 3C3" for the four data fields respectively.
• Print out the testAddress. Check the sample run for expected format.
• Check if testAddress has the same content with SMUAddress and print out
the result. Check the sample run for expected format.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
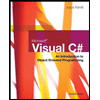
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
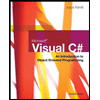
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,