You are expected to complete the skeleton code for a web server by providing the missing code in the sections marked with comments #Fill in start and #Fill in end. Each section may require one or more lines of code.It is not recommended to deviate or remove any skeleton.However, do not you skeleto code,You never need to modify code outside the start and end fill areas, do so at your own peril. Using the skeleton code provided, you will develop a web server that handles one HTTP request at a time. Your web server should: Accept and parse the HTTP request Get the requested file from the server’s file system Create an HTTP response message consisting of the requested file preceded by header lines Send the response directly to the client. For the header ‘Server’ feel free to use one of your own choice/making. Ensure you include all relevant header lines. If you forget any required headers, GradeScope will tell you. If the requested file is not present on the server, the server should send an HTTP “404 Not Found” error message back to the client. i.e. a valid request should serve an HTML file to the client, and an invalid request should serve a “404 Not Found” error message. There are clients (browsers) that will not present HTML content unless encoded HTTP headers are submitted (such as Content-Type, Server Connection) with the message from the web server. IMPORTANT HTTP status codes “200 OK” and “404 Not Found” are required to be part of the Web Server in order to receive full credit on this assignment. Do not send line by line. Prepare your entire transmission and send as one event. That means, send should be called only once for transmission. # import socket module from socket import * # In order to terminate the program import sys def webServer(port=13331): serverSocket = socket(AF_INET, SOCK_STREAM) #Prepare a server socket serverSocket.bind(("", port)) #Fill in start #Fill in end while True: #Establish the connection print('Ready to serve...') connectionSocket, addr = #Fill in start -are you accepting connections? #Fill in end try: message = #Fill in start -a client is sending you a message #Fill in end filename = message.split()[1] #opens the client requested file. #Plenty of guidance online on how to open and read a file in python. How should you read it though if you plan on sending it through a socket? f = open(filename[1:], #fill in start #fill in end) #fill in end #This variable can store the headers you want to send for any valid or invalid request. What header should be sent for a response that is ok? #Fill in start #Content-Type is an example on how to send a header as bytes. There are more! outputdata = b"Content-Type: text/html; charset=UTF-8\r\n" #Note that a complete header must end with a blank line, creating the four-byte sequence "\r\n\r\n" Refer to https://w3.cs.jmu.edu/kirkpams/OpenCSF/Books/csf/html/TCPSockets.html #Fill in end for i in f: #for line in file #Fill in start - append your html file contents #Fill in end #Send the content of the requested file to the client (don't forget the headers you created)! # Fill in start # Fill in end connectionSocket.close() #closing the connection socket except Exception as e: # Send response message for invalid request due to the file not being found (404) # Remember the format you used in the try: block! #Fill in start #Fill in end #Close client socket #Fill in start #Fill in end #Commenting out the below, as its technically not required and some students have moved it erroneously in the While loop. DO NOT DO THAT OR YOURE GONNA HAVE A BAD TIME. #serverSocket.close() #sys.exit() # Terminate the program after sending the corresponding data if __name__ == "__main__": webServer(13331)
You are expected to complete the skeleton code for a web server by providing the missing code in the sections marked with comments #Fill in start and #Fill in end.
Each section may require one or more lines of code.It is not recommended to deviate or remove any skeleton.However, do not you skeleto code,You never need to modify code outside the start and end fill areas,
do so at your own peril.
- Using the skeleton code provided, you will develop a web server that handles one HTTP request at a time.
- Your web server should:
- Accept and parse the HTTP request
- Get the requested file from the server’s file system
- Create an HTTP response message consisting of the requested file preceded by header lines
- Send the response directly to the client.
- For the header ‘Server’ feel free to use one of your own choice/making.
- Ensure you include all relevant header lines. If you forget any required headers, GradeScope will tell you.
- If the requested file is not present on the server, the server should send an
HTTP “404 Not Found” error message back to the client. - i.e. a valid request should serve an HTML file to the client, and an invalid request should serve a “404 Not Found” error message.
There are clients (browsers) that will not present HTML content unless encoded HTTP headers are submitted (such as Content-Type, Server Connection) with the message from the web server.
IMPORTANT
HTTP status codes “200 OK” and “404 Not Found” are required to be part of
the Web Server in order to receive full credit on this assignment.
Do not send line by line. Prepare your entire transmission and send as one
event. That means, send should be called only once for transmission.
# import socket module
from socket import *
# In order to terminate the program
import sys
def webServer(port=13331):
serverSocket = socket(AF_INET, SOCK_STREAM)
#Prepare a server socket
serverSocket.bind(("", port))
#Fill in start
#Fill in end
while True:
#Establish the connection
print('Ready to serve...')
connectionSocket, addr = #Fill in start -are you accepting connections? #Fill in end
try:
message = #Fill in start -a client is sending you a message #Fill in end
filename = message.split()[1]
#opens the client requested file.
#Plenty of guidance online on how to open and read a file in python. How should you read it though if you plan on sending it through a socket?
f = open(filename[1:], #fill in start #fill in end)
#fill in end
#This variable can store the headers you want to send for any valid or invalid request. What header should be sent for a response that is ok?
#Fill in start
#Content-Type is an example on how to send a header as bytes. There are more!
outputdata = b"Content-Type: text/html; charset=UTF-8\r\n"
#Note that a complete header must end with a blank line, creating the four-byte sequence "\r\n\r\n" Refer to https://w3.cs.jmu.edu/kirkpams/OpenCSF/Books/csf/html/TCPSockets.html
#Fill in end
for i in f: #for line in file
#Fill in start - append your html file contents #Fill in end
#Send the content of the requested file to the client (don't forget the headers you created)!
# Fill in start
# Fill in end
connectionSocket.close() #closing the connection socket
except Exception as e:
# Send response message for invalid request due to the file not being found (404)
# Remember the format you used in the try: block!
#Fill in start
#Fill in end
#Close client socket
#Fill in start
#Fill in end
#Commenting out the below, as its technically not required and some students have moved it erroneously in the While loop. DO NOT DO THAT OR YOURE GONNA HAVE A BAD TIME.
#serverSocket.close()
#sys.exit() # Terminate the program after sending the corresponding data
if __name__ == "__main__":
webServer(13331)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

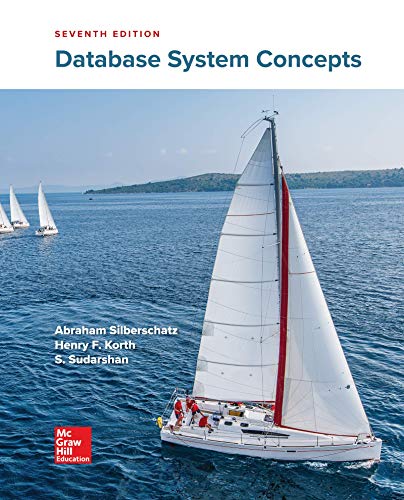
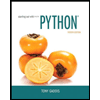
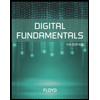
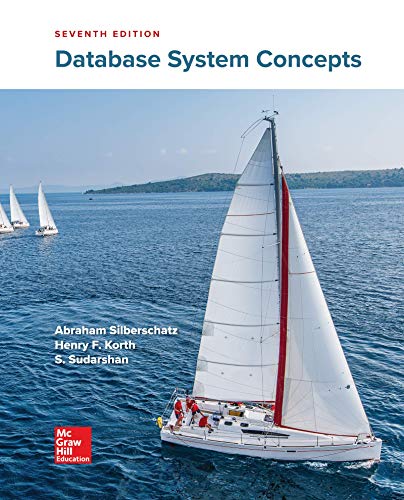
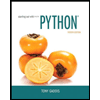
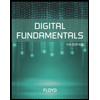
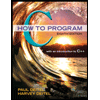
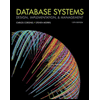
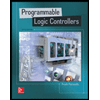