Design and implement a class to mimic a list of printers. Each printer should have a status with values as either "busy" or "free". The class should have the following functionalities:
Please write in C++


// iostream in C++ is the standard library which implement stream based input/output capabilities
#include <iostream>
// String is C standard library Which contain micro definition, Constant and declaration of function, types that are used not only for string handling but also for various memory handling function
#include <string>
using namespace std;
int getNumPrinters() //This is to get the number of printers in the system
{
int num_printers;
cout<<"Please enter the numner of printers:"<<endl; // Here we are taking the user input for number of printers
cin>>num_printers;
return num_printers; //return the number of printers
}
//This function returns the number of free printers
int getNumFreePrinters(int num_of_printers,int status[])
{
int num_free_printers=0; //Initially the count of free printers is 0.
for(int i=0;i<num_of_printers;i++)
{
if(status[i]==0) num_free_printers++; //if printer is free increment the count of free printers
}
return num_free_printers; //return the free printers count
}
void usePrinter(int index,int num_of_printers,int status[])
{
if(status[index]==0) cout<<"Printer at index "<<index<<" is Free to use \n" ; //if printer is free then we use it
else cout<<"Printer at index "<<index<<" is already Busy \n"; //error message if already in use
for(int i=0;i<num_of_printers;i++)
{
if(status[index]==0) status[index]=1; //if printer is free we make it busy for the given index
}
}
void freePrinter(int index,int num_of_printers,int status[])
{
if(status[index]==1) cout<<"Making Printer at index "<<index<<" Free to use\n"; //if busy we make it free
else cout<<"Printer at index "<<index<<" is already Free to use \n"; //if free then show error message
for(int i=0;i<num_of_printers;i++)
{
if(status[index]==1) status[index]=0; //if printer is busy we make it free for the given index
}
}
int main()
{
int num_of_printers; //variable for number of printers
int num_of_free_printers;
int index;
num_of_printers=getNumPrinters(); // Here we reading the number of printers
cout<<num_of_printers<<endl;
int status[num_of_printers]; // Here we are creating array to store status free or busy
for(int i=0;i<num_of_printers;i++)
{
status[i]=0; //initially all the printers are free
}
num_of_free_printers=getNumFreePrinters(num_of_printers,status); //calling the function to read number of free printers
cout<<"\nNumber of free printers is/are : "<<num_of_free_printers<<endl;
cout<<"Enter the index of the printer to use"<<endl; // Here we read index for which the printer is to be used
cin>>index;
cout<<"You have enterd the index:"<<index<<endl;
usePrinter(index,num_of_printers,status); // Here we are calling usePrinter function
cout<<"\nEnter the index of the printer to use"<<endl; //Here we read index for which the printer is to be used
cin>>index;
cout<<"You have enterd the index:"<<index<<endl;
usePrinter(index,num_of_printers,status); //calling usePrinter function
num_of_free_printers=getNumFreePrinters(num_of_printers,status); // here we are calling the function to read number of free printers
cout<<"\nNumber of free printers is/are : "<<num_of_free_printers<<endl;
cout<<"\nEnter the index of the printer to Free"<<endl; // Here we read index for which the printer to free cin>>index;
cout<<"You have enterd the index:"<<index<<endl;
freePrinter(index,num_of_printers,status); // Here we are calling freePrinter function
cout<<"\nEnter the index of the printer to Free"<<endl; // here we read index for which the printer to free
cin>>index;
cout<<"You have enterd the index:"<<index<<endl;
freePrinter(index,num_of_printers,status); // here we are calling freePrinter function
num_of_free_printers=getNumFreePrinters(num_of_printers,status); //here we are calling the function to read number of free printers
cout<<"\nNumber of free printers is/are : "<<num_of_free_printers<<endl;
}
Step by step
Solved in 2 steps

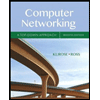
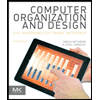
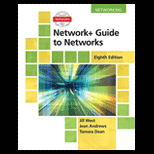
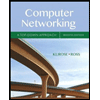
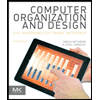
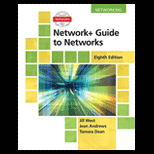
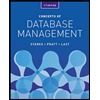
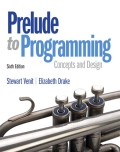
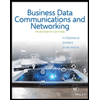