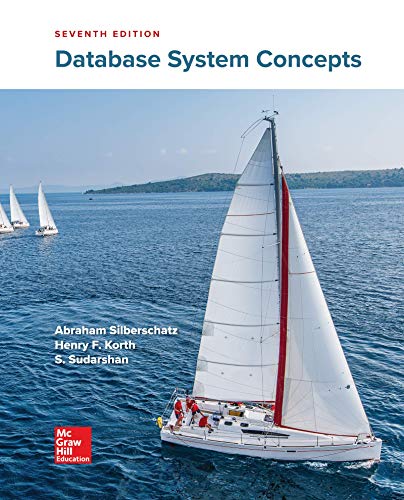
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
The output should be a text file with the final position and the facing direction (no GUI required)
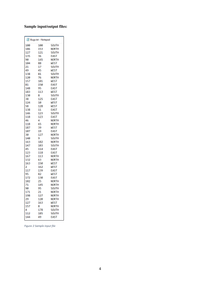
Transcribed Image Text:Sample input/output files:
Bugs.txt - Notepad
100
100
SOUTH
186
153
NORTH
127
121
SOUTH
135
36
EAST
90
104
145
NORTH
88
WEST
21
49
17
SOUTH
45
WEST
138
81
SOUTH
120
76
NORTH
157
101
WEST
81
150
EAST
148
95
EAST
183
113
WEST
130
8
SOUTH
38
125
EAST
124
10
WEST
58
128
WEST
138
11
EAST
146
123
SOUTH
118
46
118
123
EAST
4
NORTH
65
NORTH
187
39
WEST
107
19
EAST
30
127
NORTH
140
SOUTH
163
147
182
NORTH
103
SOUTH
45
114
EAST
123
118
EAST
167
113
NORTH
132
63
NORTH
163
150
WEST
2
117
95
162
WEST
139
EAST
82
WEST
172
130
EAST
102
25
NORTH
71
90
145
NORTH
95
SOUTH
171
21
NORTH
198
127
NORTH
29
120
NORTH
127
163
WEST
157
8
NORTH
4
178
SOUTH
112
185
SOUTH
144
49
EAST
Figure 2 Sample input file
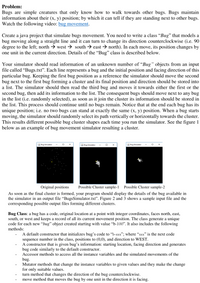
Transcribed Image Text:Problem:
Bugs are simple creatures that only know how to walk towards other bugs. Bugs maintain
information about their (x, y) position; by which it can tell if they are standing next to other bugs.
Watch the following video: bug movement.
Create a java project that simulate bugs movement. You need to write a class “Bug" that models a
bug moving along a straight line and it can turn to change its direction counterclockwise (i.e. 90
degree to the left; north → west → south → east → north). In each move, its position changes by
one unit in the current direction. Details of the "Bug" class is described below.
Your simulator should read information of an unknown number of "Bug" objects from an input
file called “Bugs.txt". Each line represents a bug and the initial position and facing direction of this
particular bug. Keeping the first bug position as a reference the simulator should move the second
bug next to the first bug forming a cluster and its final position and direction should be stored into
a list. The simulator should then read the third bug and moves it towards either the first or the
second bug, then add its information to the list. The consequent bugs should move next to any bug
in the list (i.e. randomly selected), as soon as it join the cluster its information should be stored in
the list. This process should continue until no bugs remain. Notice that at the end each bug has its
unique position; i.e. no two bugs can stand at exactly the same (x, y) position. When a bug starts
moving, the simulator should randomly select its path vertically or horizontally towards the cluster.
This results different possible bug cluster shapes each time you run the simulator. See the figure 1
below as an example of bug movement simulator resulting a cluster.
Bug Simulator
Bug Simulator
O X
Bug Simulator
Original positions
Possible Cluster sample-1 Possible Cluster sample-2
As soon as the final cluster is formed, your program should display the details of the bug available in
the simulator in an output file “BugsSimulator.txt". Figure 2 and 3 shows a sample input file and the
corresponding possible output files forming different clusters.
Bug Class: a bug has a code, original location at a point with integer coordinates, faces north, east,
south, or west and keeps a record of all its current movement position. The class generate a unique
code for each new “bug" object created starting with value "b-I00". It also includes the following
methods:
A default constructor that initializes bug's code to "b-xxx"; where "xxx" is the next code
sequence number in the class, positions to (0,0), and direction to WEST.
A constructor that is given bug's information: starting location, facing direction and generates
bug code similarly to the default constructor.
Accessor methods to access all the instance variables and the simulated movements of the
לל
XXX
bug.
Mutator methods that change the instance variables to given values and they make the change
for only suitable values.
turn method that changes the direction of the bug counterclockwise.
move method that moves the bug by one unit in the direction it is facing.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- The name of the image file assigned to a picture box control is stored in thecontrol’s________ property.arrow_forwardPlease help me with this. I am not understanding how and what to do?arrow_forwardThe Accumulator Step 1: Start Raptor and save your document as Lab 5-2Accumulator. The .rap file extension will be added automatically. Step 2: The next loop to code is the pseudocode from Step 10, Lab 5.1. This loop will take in a number and accumulate the total. The complete pseudocode is below: Declare Integer counter Declare Integer total = 0 Declare Integer number For counter = 1 to 5 Display "Enter a number: " Input number Set total = total + number End For Display "The total is total: ", total Step 3: Click the Loop symbol and add it between the Start and the End symbol. Above the Loop symbol, add three assignment statements. Set a variable named counter to 1, a variable named total to 0, and a variable named number to 0. Step 4: Double click the Diamond symbol and add the condition that will execute the loop through 5 iterations. Step 5: Add an input statement if the loop is NO. This statement will ask the user to enter a number. Step…arrow_forward
- C++ You are given the root node of a binary search tree (BST) and a value to insert into the tree. Return the root node of the BST after the insertion. It is guaranteed that the new value does not exist in the original BST. Example 1: diagram for example 1 attached Input: root = [4,2,7,1,3], val = 5 Output: [4,2,7,1,3,5] Explanation: Another accepted tree is: Example 2: Input: root = [40,20,60,10,30,50,70], val = 25 Output: [40,20,60,10,30,50,70,null,null,25] Example 3: Input: root = [4,2,7,1,3,null,null,null,null,null,null], val = 5 Output: [4,2,7,1,3,5] Constraints: The number of nodes in the tree will be in the range [0, 104]. -108 <= Node.val <= 108 All the values Node.val are unique. -108 <= val <= 108 It's guaranteed that val does not exist in the original BST.arrow_forwardWhat does the Form1.cs file contain?arrow_forwardGUI 2. JMenu code is provided in question (a) Enhance this program to have 3 menus File, Format, and Format List. (b) Modify File menu to have a menu item Read as below Using JMenus File Format Format List Read... Exit Input ? When you hit Read..., a dialog pops up asking you to type in text: Sample Text Enter a text I like challenges Dr. Lin's JMenus File Format OK Font Color Background Color Font Cancel If you type "I like challenges", then text "Sample Text" will be changed to "I like challenges". Pick your own text and also change to a different color like Red. X (c) Enhance the Format menu so that you can change both the font color and background color as follows. There are 4 choices for Background: White, Cyan, Magenta, and Yellow. The following shows the enhanced Format menu without displaying the new Format List Menu. ●White Ⓒ Cyan O Magenta Sample Text Using JMenus File Format Format List Xx I like challenges File Format Dr. Lin's JMenus Sample Text Figure 3 Select Background…arrow_forward
- When a file is named with a .csv extension, does that mean that the data within the file is in CSV format? YesNoarrow_forwardYour application will demonstrate the use of a loop to determine the smallest value entered by the user. Incorporate the following requirements into your application: The program will consist of one file - the main application class Name the class appropriately and name the file Program.cs (the default when you create the application) Include documentation at the top of the file that includes Your name Date of development Assignment (e.g., CIS214 Performance Assessment - Smallest Number) Description of the class The main application class must meet the following requirements Print a line that states "Your Name - Week 2 PA Smallest Number" Ask the user how many integers they will enter Loop to get the specified number of integers from the user Print the value of the smallest integer entered by the userarrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
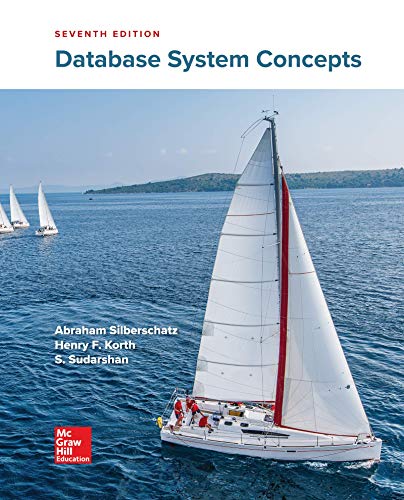
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
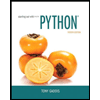
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
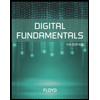
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
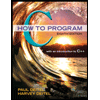
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
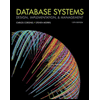
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
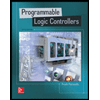
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education