Doubly Linked List (C Language, without headers) This assignment asks you to sort the letters in an input file and print the sorted letters to an output file (or standard output) which will be the solution. Your program, called codesolve, will take the following command line arguments: % codesolve [-o output_file_name] input_file_name Read the letters in from the input file and convert them to upper case if they are not already in uppercase. If the output_file_name is given with the -o option, the program will output the sorted letters to the given output file; otherwise, the output shall be to standard output. In addition to parsing and processing the command line arguments, your program needs to do the following: You need to construct a doubly linked list as you read from input. Each node in the list will link to the one in front of it and the one behind it if they exist. They will have NULL for the previous pointer if they are first in the list. They will have NULL for the next pointer if they are last in the list. You can look up doubly linked lists in your Data Structure textbook. Initially the list is empty. The program reads from the input file one letter at a time and converts it to upper case. Create a node with the letter and 2 pointers to nodes, previous and next. Initially the previous and next pointers should be NULL. As long as you continue reading letters, if the letter is not already in the list, create a new node and place the node into the list in the proper Alphabetical order. If there is a node in front of it, the previous pointer should point to it. If there is a node after it then the next pointer should point to it. All duplicate letters are ignored. An end of file would indicate the end of input from the input file. Once the program has read all the input, the program then performs a traversal of the doubly linked list first to last to print one letter at a time to the output file or stdout. The output would be all letters on the same line. Before the program ends, it must reclaim the list! You can do this by going through the list and freeing all nodes. This can be done in either direction. It is required that you use getopt for processing the command line and use malloc or calloc and free functions for dynamically allocating and deallocating nodes. Directions: Makefile named Makefile. Typing make at the command line should create executable named codesolve. Make file should include a clean target which removed .o files There should be no warnings during compile on ocelot. Program should have consistent indentation. Consistent indentation with curly braces lined up in either of the two standard formats. They can be lined up right above each other or the opening curly brace can be on the right end of the line above with the block begins. Program should have good comments throughout the body and describing each function. Code should be readable with good variable and function names. No use of break or continue statements except in a switch. Program must exit with a return code of 0 on success and an error code in other cases. The program used getopt to parse the command line. Whenever an error occurs on the command line the user is given the usage statement and an appropriate error message if needed. A node structure is included. It should include the letter, and the previous and next pointers to other nodes. The program must dynamically allocate the memory for the nodes. The program must free the nodes after the output is completed. If the -o option is used the output will go to a file named as specified without changing the filename at all otherwise it will go to stdout. Execution of the program will end with an EOF from an input file. codesolve infile codesolve -o outfile infile
Doubly Linked List (C Language, without headers)
This assignment asks you to sort the letters in an input file and print the sorted letters to an output file (or standard output) which will be the solution. Your program, called codesolve, will take the following command line arguments:
% codesolve [-o output_file_name] input_file_name
Read the letters in from the input file and convert them to upper case if they are not already in uppercase. If the output_file_name is given with the -o option, the program will output the sorted letters to the given output file; otherwise, the output shall be to standard output.
In addition to parsing and processing the command line arguments, your program needs to do the following:
- You need to construct a doubly linked list as you read from input. Each node in the list will link to the one in front of it and the one behind it if they exist. They will have NULL for the previous pointer if they are first in the list. They will have NULL for the next pointer if they are last in the list. You can look up doubly linked lists in your Data Structure textbook.
- Initially the list is empty. The program reads from the input file one letter at a time and converts it to upper case. Create a node with the letter and 2 pointers to nodes, previous and next. Initially the previous and next pointers should be NULL.
- As long as you continue reading letters, if the letter is not already in the list, create a new node and place the node into the list in the proper Alphabetical order. If there is a node in front of it, the previous pointer should point to it. If there is a node after it then the next pointer should point to it. All duplicate letters are ignored.
- An end of file would indicate the end of input from the input file.
- Once the program has read all the input, the program then performs a traversal of the doubly linked list first to last to print one letter at a time to the output file or stdout. The output would be all letters on the same line.
- Before the program ends, it must reclaim the list! You can do this by going through the list and freeing all nodes. This can be done in either direction.
- It is required that you use getopt for processing the command line and use malloc or calloc and free functions for dynamically allocating and deallocating nodes.
Directions:
Makefile named Makefile.
Typing make at the command line should create executable named codesolve.
Make file should include a clean target which removed .o files
There should be no warnings during compile on ocelot.
Program should have consistent indentation.

Step by step
Solved in 4 steps with 8 images

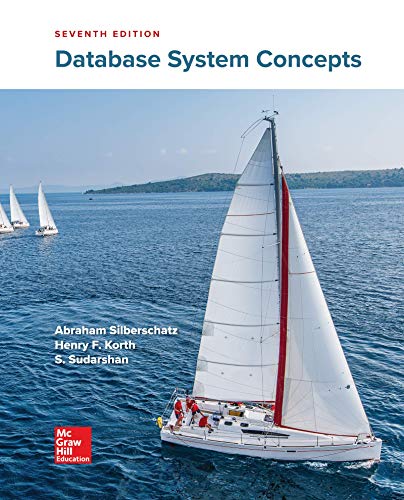
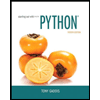
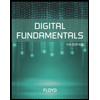
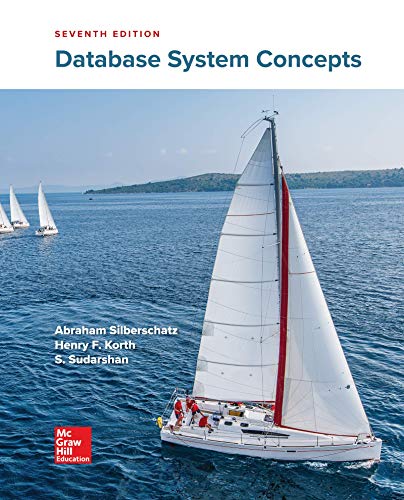
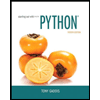
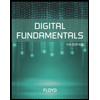
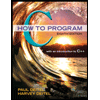
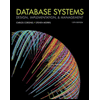
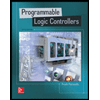