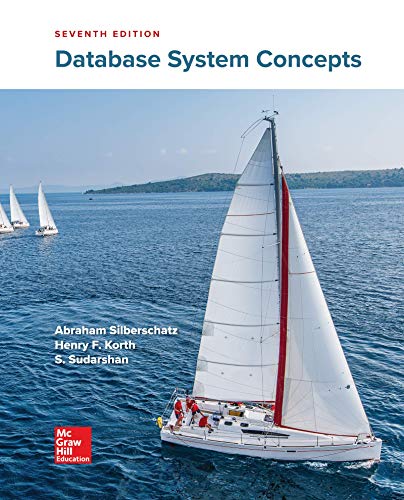
.......Python Code.............
Python file:
# Write a function that takes an open hockey file and returns a list of tuples,
# where each tuple refers to a team name and that team's maximum number of wins in any year.
from typing import TextIO
def teams_and_most_wins(f: TextIO) -> list[tuple[str, int]]:
# Return a list of tuples where each tuple stores a team name and
# that team's maximum number of wins in any year.
# DO CODE HERE ... Use this
'''
f is an open hockey file.
Each year has 4 digits and each number of wins has 2 digits.
Every team should appear in exactly one of the tuples.
'''
.......File.............
Each line of a hockey file contains a four-digit year, a team name, and a two-digit number of wins.
Here is one such file, hockey.txt:
>>>
2003maple leafs45
2020maple leafs35
1995senators18
1996maple leafs30
2000senators48
2012canadiens48
>>>
The first line of the file, for example, says that in 2003 (year),
the maple leafs (team name) had 45 wins.
.......Sample and Result.............
Here is a sample call of the function on hockey.txt:
>>> teams_and_most_wins(open('hockey.txt'))
[('maple leafs', 45), ('senators', 48), ('canadiens', 48)]

Step by stepSolved in 4 steps with 3 images

- The function interleave_lists in python takes two parameters, L1 and L2, both lists. Notice that the lists may have different lengths. The function accumulates a new list by appending alternating items from L1 and L2 until one list has been exhausted. The remaining items from the other list are then appended to the end of the new list, and the new list is returned. For example, if L1 = ["hop", "skip", "jump", "rest"] and L2 = ["up", "down"], then the function would return the list: ["hop", "up", "skip", "down", "jump", "rest"]. HINT: Python has a built-in function min() which is helpful here. Initialize accumulator variable newlist to be an empty list Set min_length = min(len(L1), len(L2)), the smaller of the two list lengths Use a for loop to iterate k over range(min_length) to do the first part of this function's work. On each iteration, append to newlist the item from index k in L1, and then append the item from index k in L2 (two appends on each iteration). AFTER the loop…arrow_forwardWrite a function that will accept a list of integers and return the sum of the contents of the list(……if the list contains 1,2,3 it will return 6…..)arrow_forwardPython Write a function that takes as a parameter a list of strings and returns a list containing the lengths of each of the strings. That is, if the input parameter is ["apple pie", "brownies","chocolate","dulce de leche","eclairs"], your function should return [9, 8, 9, 14, 7]. The file you submit should include a main() function that demonstrates that your function works. The list that is the input argument can be a hard-coded one like the one shown above or you could ask the user to input a string and split on the appropriate delimiter, creating a list. Hint: This problem can be approached as an "accumulator", where we accumulate lists:arrow_forward
- The function list_combination takes two lists as arguments, list_one and list_two. Return from the function the combination of both lists - that is, a single list such that it alternately takes elements from both lists starting from list_one. You can assume that both list_one and list_two will be of equal lengths. As an example if list_one = [1,3,5] and if list_two = [2,4,6]. The function must return [1,2,3,4,5,6].arrow_forwardpython LAB: Subtracting list elements from max When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Write a program that adjusts a list of values by subtracting each value from the maximum value in the list. The input begins with an integer indicating the number of integers that follow.arrow_forwardWrite a function called find_duplicates which accepts one list as a parameter. This function needs to sort through the list passed to it and find values that are duplicated in the list. The duplicated values should be compiled into another list which the function will return. No matter how many times a word is duplicated, it should only be added once to the duplicates list. NB: Only write the function. Do not call it. For example: Test Result random_words = ("remember","snakes","nappy","rough","dusty","judicious","brainy","shop","light","straw","quickest", "adventurous","yielding","grandiose","replace","fat","wipe","happy","brainy","shop","light","straw", "quickest","adventurous","yielding","grandiose","motion","gaudy","precede","medical","park","flowers", "noiseless","blade","hanging","whistle","event","slip") print(find_duplicates(sorted(random_words))) ['adventurous', 'brainy', 'grandiose', 'light', 'quickest', 'shop', 'straw', 'yielding']…arrow_forward
- The mapped list pattern Our second pattern is the mapped list pattern, described in video 4 3 mapped list pattern. Often we need to write a function that takes a list as a parameter and returns a new list in which each item in the original list is "mapped" to a new item in the result list. For example, the following function takes a list of numbers as a parameter and returns a list of all the numbers squared, e.g. squares ( [1, 3, 7]) returns [1, 9, 49]. def squares (nums): "Returns the squares of the given numbers""" result = [] for num in nums: result.append (num * num) return result Although this is just a special case of the accumulator pattern, it is so common that we give it its own name: the mapped list pattern. Consider the following function: def squares(nums): ""Returns the squares of the given numbers""" result = [] for num in nums: result.append (num * num) return result If the main program calls print(squares ( [5, -3, 2, 7]) what is the state table for the function…arrow_forwardIn Python IDLE: How would I write a function for the problem in the attached image?arrow_forwardWrite a program with two functions: addToList(food): printList(food): Print the entire list food is used to pass a list to the function. Ask the user to input another food item. The main program will create a list: myList["apple", "oralge", "grapes] Call the function printList, using myList Call the function addToList, using myList Call the function printList, using myListarrow_forward
- The function should be written in python language. Write a function called "zero_triples" to return all triples in a list of integers that sum to zero. You can assume the list won't contain any duplicates, and a triple should not use the same number more than once. [In]: zero_triples([1, 2, 4]) [Out]: [] [In]: zero_triples([-3, 1, 4, 2]) [Out]: [[1, 2, -3]] [In]: zero_triples([-9, 1, -3, 2, 4, 5, -4, -1]) [Out]: [[-9, 4, 5], [1, -3, 2], [-3, 4, -1], [5, -4, -1]]arrow_forwardPYTHON Complete the function below, which takes two arguments: data: a list of tweets search_words: a list of search phrases The function should, for each tweet in data, check whether that tweet uses any of the words in the list search_words. If it does, we keep the tweet. If it does not, we ignore the tweet. data = ['ZOOM earnings for Q1 are up 5%', 'Subscriptions at ZOOM have risen to all-time highs, boosting sales', "Got a new Mazda, ZOOM ZOOM Y'ALL!", 'I hate getting up at 8am FOR A STUPID ZOOM MEETING', 'ZOOM execs hint at a decline in earnings following a capital expansion program'] Hint: Consider the example_function below. It takes a list of numbers in numbers and keeps only those that appear in search_numbers. def example_function(numbers, search_numbers): keep = [] for number in numbers: if number in search_numbers(): keep.append(number) return keep def search_words(data, search_words):arrow_forwardNeed help with this short python questionarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
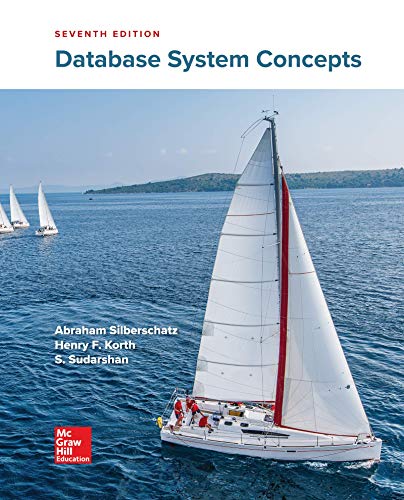
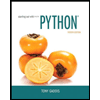
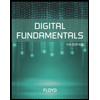
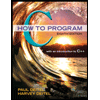
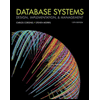
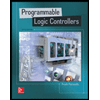