Draw a UML diagram of the following classes? The classes of this program represent an elevator. Press of button 1, 2, or 3 brings you to that Floor. public class Elevator { Floor floor; Context context; State closedState; State openState; State state; public Elevator() { floor = new Floor(1); context = new Context(); closedState = new ClosedState(); openState = new OpenState(); state = openState; state.doAction(context); } public static void main(String[] args) { Elevator elevator = new Elevator(); elevator.press2(); elevator.press1(); elevator.press3(); elevator.press3(); elevator.press1(); } public void move(Floor floor, int requestedFloor) { String door = context.getState().toString(); String closed = "Doors are closed"; System.out.println("\n"+ requestedFloor + " pressed"); if(!door.equals(closed)) { state = closedState; closeDoors(); } if (requestedFloor == floor.getFloor()) { System.out.print("Nothing happens"); }else if (requestedFloor < floor.getFloor()) { floor.setFloor(requestedFloor); System.out.printf("Going down...\n*ding* The elevator arrives at Floor %d", floor.getFloor()); state = openState; openDoors(); }else { floor.setFloor(requestedFloor); System.out.printf("Going up...\n*ding* The elevator arrives at Floor %d", floor.getFloor()); state = openState; openDoors(); } } private void press1() { move(floor,1); } private void press2() { move(floor,2); } private void press3() { move(floor,3); } private void openDoors() { state.doAction(context); System.out.print(context.getState().toString()); } private void closeDoors() { state.doAction(context); System.out.println(context.getState().toString()); } } public class ClosedState implements State { @Override public void doAction(Context context) { context.setState(this); } public String toString(){ return "Doors are closed"; } } public class Context { private State state; public Context() { state = null; } public void setState(State state){ this.state = state; } public State getState(){ return state; } } public class Floor { private int floor; public Floor(int num){ this.floor = num; } public int getFloor() { return floor; } public void setFloor(int num) { this.floor = num; } } public class OpenState implements State { @Override public void doAction(Context context) { context.setState(this); } public String toString(){ return "Doors are open"; } } public interface State { void doAction(Context context); }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Draw a UML diagram of the following classes?
The classes of this program represent an elevator. Press of button 1, 2, or 3 brings you to that Floor.
public class Elevator {
Floor floor;
Context context;
State closedState;
State openState;
State state;
public Elevator() {
floor = new Floor(1);
context = new Context();
closedState = new ClosedState();
openState = new OpenState();
state = openState;
state.doAction(context);
}
public static void main(String[] args) {
Elevator elevator = new Elevator();
elevator.press2();
elevator.press1();
elevator.press3();
elevator.press3();
elevator.press1();
}
public void move(Floor floor, int requestedFloor) {
String door = context.getState().toString();
String closed = "Doors are closed";
System.out.println("\n"+ requestedFloor + " pressed");
if(!door.equals(closed)) {
state = closedState;
closeDoors();
}
if (requestedFloor == floor.getFloor()) {
System.out.print("Nothing happens");
}else if (requestedFloor < floor.getFloor()) {
floor.setFloor(requestedFloor);
System.out.printf("Going down...\n*ding* The elevator arrives at Floor %d", floor.getFloor());
state = openState;
openDoors();
}else {
floor.setFloor(requestedFloor);
System.out.printf("Going up...\n*ding* The elevator arrives at Floor %d", floor.getFloor());
state = openState;
openDoors();
}
}
private void press1() { move(floor,1); }
private void press2() { move(floor,2); }
private void press3() { move(floor,3); }
private void openDoors() {
state.doAction(context);
System.out.print(context.getState().toString());
}
private void closeDoors() {
state.doAction(context);
System.out.println(context.getState().toString());
}
}
public class ClosedState implements State {
@Override
public void doAction(Context context) { context.setState(this); }
public String toString(){
return "Doors are closed";
}
}
public class Context {
private State state;
public Context() { state = null; }
public void setState(State state){
this.state = state;
}
public State getState(){
return state;
}
}
public class Floor {
private int floor;
public Floor(int num){ this.floor = num; }
public int getFloor() { return floor; }
public void setFloor(int num) { this.floor = num; }
}
public class OpenState implements State {
@Override
public void doAction(Context context) { context.setState(this); }
public String toString(){ return "Doors are open"; }
}
public interface State {
void doAction(Context context);
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

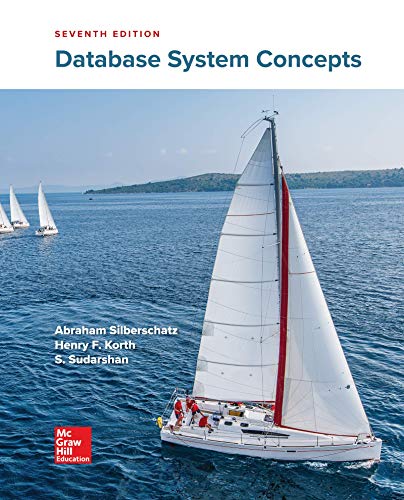
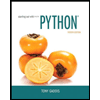
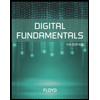
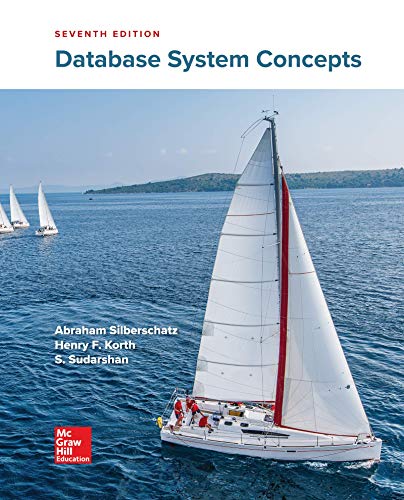
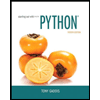
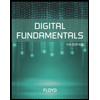
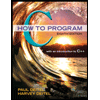
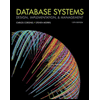
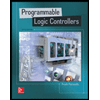