Write a java program as shown in the following UML class diagram:(read the details below) The classes Name and Date are separate classes used as properties in the Employee class. Class Employee is the superclass of the classes SalariedEmployee and HourlyEmployee. The superclass has the attributes name and data of hire and methods to set and get these attributes. It also has 2 abstract methods equals() which check if the objects are same and toString() which convert the properties to a printable string. Also the method read() which used as an input method. The SalariedEmployee subclass has in addition to the attributes of the super-class the attribute annualSalary and set and get methods for it. This class also defines the read() method and grossPay() which in this case returns the salary of the employee. The HourlyEmployee subclass has in addition to the attributes of the super-class, the hoursWorked and hourlyRate attributes. It provides ‘get’ and ‘set’ methods for these attributes. Also it provides the methods equals() and toString(). Finally, it provides an implementation of the method grossPay(), which returns the product of the hourly rate with the hours worked for each employee. After creating this class hierarchy create a Main class with a main function. This function will have an array of type Employee and size 2. Place in the position 0 of this array a SalariedEmployee object and in the position 1 an HourlyEmployee object. Then set some appropriate values for the two objects. Finally create a loop that calls the grossPay() method on both objects of the array and display the returned value
Write a java program as shown in the following UML class diagram:(read the details below) The classes Name and Date are separate classes used as properties in the Employee class. Class Employee is the superclass of the classes SalariedEmployee and HourlyEmployee. The superclass has the attributes name and data of hire and methods to set and get these attributes. It also has 2 abstract methods equals() which check if the objects are same and toString() which convert the properties to a printable string. Also the method read() which used as an input method. The SalariedEmployee subclass has in addition to the attributes of the super-class the attribute annualSalary and set and get methods for it. This class also defines the read() method and grossPay() which in this case returns the salary of the employee. The HourlyEmployee subclass has in addition to the attributes of the super-class, the hoursWorked and hourlyRate attributes. It provides ‘get’ and ‘set’ methods for these attributes. Also it provides the methods equals() and toString(). Finally, it provides an implementation of the method grossPay(), which returns the product of the hourly rate with the hours worked for each employee. After creating this class hierarchy create a Main class with a main function. This function will have an array of type Employee and size 2. Place in the position 0 of this array a SalariedEmployee object and in the position 1 an HourlyEmployee object. Then set some appropriate values for the two objects. Finally create a loop that calls the grossPay() method on both objects of the array and display the returned value
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write a java
- The classes Name and Date are separate classes used as properties in the Employee class.
- Class Employee is the superclass of the classes SalariedEmployee and HourlyEmployee. The superclass has the attributes name and data of hire and methods to set and get these attributes. It also has 2 abstract methods equals() which check if the objects are same and toString() which convert the properties to a printable string. Also the method read() which used as an input method.
- The SalariedEmployee subclass has in addition to the attributes of the super-class the attribute annualSalary and set and get methods for it. This class also defines the read() method and grossPay() which in this case returns the salary of the employee.
- The HourlyEmployee subclass has in addition to the attributes of the super-class, the hoursWorked and hourlyRate attributes. It provides ‘get’ and ‘set’ methods for these attributes. Also it provides the methods equals() and toString(). Finally, it provides an implementation of the method grossPay(), which returns the product of the hourly rate with the hours worked for each employee.
After creating this class hierarchy create a Main class with a main function. This function will have an array of type Employee and size 2. Place in the position 0 of this array a SalariedEmployee object and in the position 1 an HourlyEmployee object. Then set some appropriate values for the two objects. Finally create a loop that calls the grossPay() method on both objects of the array and display the returned value.
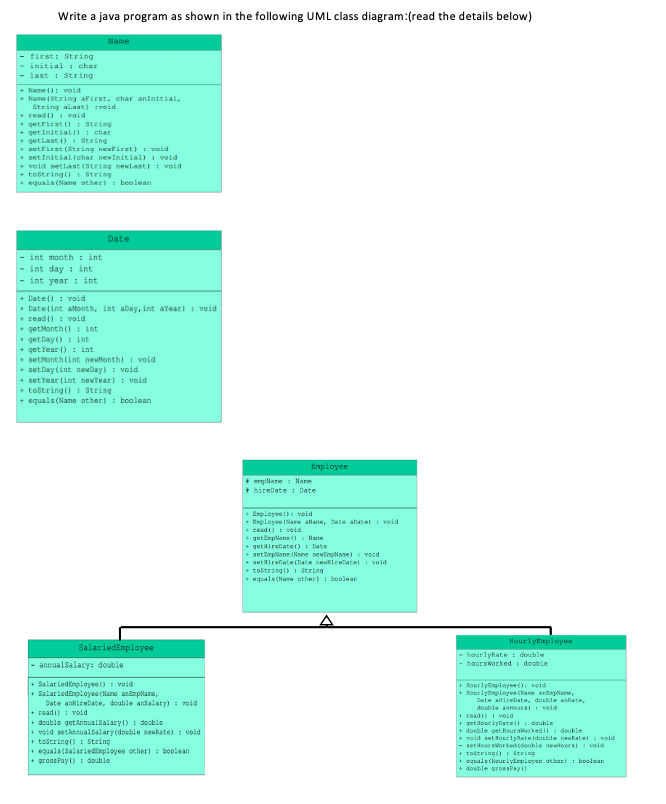
Transcribed Image Text:Write a java program as shown in the following UML class diagram:(read the details below)
Name
first: String
initial : char
- last i String
- Name ): void
• Name (String aPirst, char aninitial,
String alaat) iveid
• read () : void
• getrirst () : String
+ get Initial 0I char
• getlast () : String
setPirat (String newlirat) : void
• setInitial (char newInitial) i vold
+ void settast (String netast : void
+ tostring OI String
. equala (Nane other) boolean
Date
- int month : int
int day : int
- int year : int
+ Date() : void
• Datelint aMonth, int aDay, int aYear) : void
+ read() : void
* getMonth () : int
* getDay () I int
+ getYear () : int
• setMonth (int nevMonth) : vold
* setDay (int newbay) i void
+ setYear (int nevYear) : void
• tostring() : String
+ equals (Nane other) i boolean
Employee
* enplare i Nane
+ hirelate : Date
• Eeployee l : void
- Eeployee (Sanre allane, bate abate) : void
read) : void
getrpllane0 : Hane
- getairelate): Date
• setErplane (Nane newEnpiame) : void
- setairetate (Tate nexirelatel i void
• tostringi I String
*equals (Nane other) i boolean
Hourlykaployee
SalariedEmployee
hourlyRate: double
hoursWorked i double
- annualsalary: double
+ Salariedinployee) : void
+ Salariedinployeo (Nane anmplane,
Date ankirebate, double ansalary) : void
+ readi) : vold
+ double getannualsalary() : double
+ void setknnualsalary(double sewRate) : void
+ tostring() : String
+ equals(Salariedimployee ocher) : boolean
+ grossPay () i double
+ Kourlyaployee0: void
+ Hourlynployee (Nare antapare,
Date alirebate, double anRate,
double anhourSI : vola
+ readt)i veid
+ getilour lylate( : double
+ double getHour slocked ii double
+ vaid settoerlyRateidouble nexRatel: vold
- setHoursvorked (double nevitoursi i vold
+ tostring() : String
equala (lourlyirpleyee other : boolean
+ double gronslay(
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
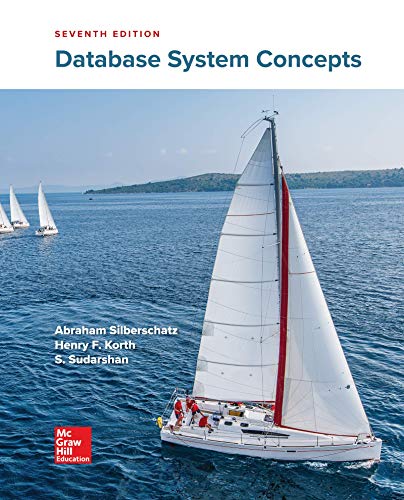
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
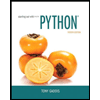
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
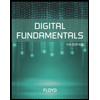
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
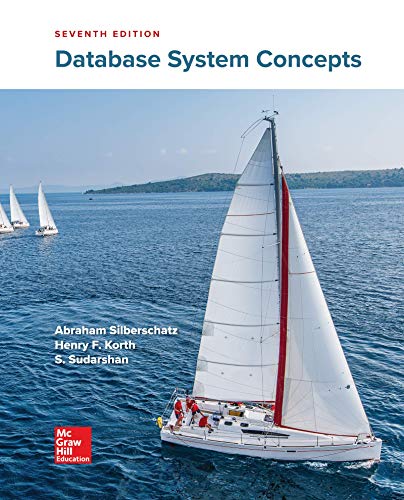
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
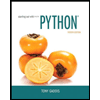
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
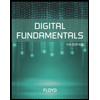
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
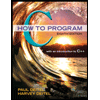
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
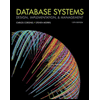
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
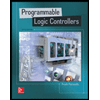
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education