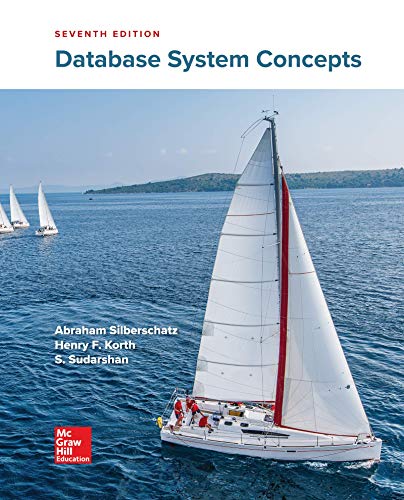
Implement a Doubly linked list to store a set of Integer numbers (no duplicate)
• Instance variable
• Constructor
• Accessor and Update methods
2. Define DLinkedList Class
a. Instance Variables:
# Node header
# Node trailer
# int size
b. Constructor
c. Methods
# int getSize() //Return the number of nodes of the list.
# int getSize() //Return the number of elements of the list.
# boolean isEmpty() //Return true if the list is empty, and false otherwise.
# E getFirst() //Return the value of the first node of the list.
# E getLast()/ /Return the value of the Last node of the list.
# addFirst(E e) //Add a new node to the front of the list.
# addLast(E e) //Add a new node to the end of the list.
# Node remove(Node n) //remove a node which has the reference n from the list
# Node removeFirst() //Remove the first node of the list, and return the removed node or null if failed.
# Node removeLast() //Remove the last node of the list, and return the removed node or null if failed.
# addBetween(E e, Node predecessor, Node successor) //Add a new node between the predecessor and successor.
# Node search(E key) //Search and return a node according to a given key
# Node update(E key, E e) //update the value of a given k to a new value.
# display() //Display all nodes of the list by traversing the linked list.
# addAfter(E e, E key) // Add a new node e after a given key of the list.
# addBefore(E e, E key) // Add a new node e before a given key of the list.
# Node removeAt(E key) //Remove node based on a given key, return the removed node or null if failed

Step by stepSolved in 3 steps with 6 images

- Tree Define a class called TreeNode containing three data fields: element, left and right. The element is a generic type. Create constructors, setters and getters as appropriate. Define a class called BinaryTree containing two data fields: root and numberElement. Create constructors, setters and getters as appropriate. Define a method balanceCheck to check if a tree is balanced. A tree being balanced means that the balance factor is -1, 0, or 1.arrow_forwardImplement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 2.) Define SLinkedList Classa. Instance Variables: # Node head # Node tail # int sizeb. Constructorc. Methods # int getSize() //Return the number of nodes of the list. # boolean isEmpty() //Return true if the list is empty, and false otherwise. # int getFirst() //Return the value of the first node of the list. # int getLast()/ /Return the value of the Last node of the list. # Node getHead()/ /Return the head # setHead(Node h)//Set the head # Node getTail()/ /Return the tail # setTail(Node t)//Set the tail # addFirst(E e) //add a new element to the front of the list # addLast(E e) // add new element to the end of the list # E removeFirst()//Return the value of the first node of the list # display()/ /print out values of all the nodes of the list # Node search(E key)//check if a given…arrow_forwardAssume you have a class SLNode representing a node in a singly-linked list and a variable called list referencing the first element on a list of integers, as shown below: public class SLNode { private E data; private SLNode next; public SLNode( E e){ data = e; next = null; } public SLNode getNext() { return next; } public void setNext( SLNoden){ next = n; } } SLNode list; Write a fragment of Java code that would append a new node with data value 21 at the end of the list. Assume that you don't know if the list has any elements in it or not (i.e., it may be empty). Do not write a complete method, but just show a necessary fragment of code.arrow_forward
- In Java please help with the following: Sees whether this list is empty.@return True if the list is empty, or false if not. */ public boolean isEmpty(); } // end ListInterface Hint:Node class definition should look something like: public class Node<T> { T element; Node next; Node prev; public Node(T element, Node next, Node prev) { this.element = element;this.next = next;this.prev = prev; } }arrow_forward1 Assume some Node class with info & link fields. Complete this method in class List that returns a reference to the node containing the data item in the argument find This, Assume that find this is in the list public class List { protected Node head; protected int size; fublic Public Node find (char find This)arrow_forwardSorting Create a MyLinkedList class with inner Node class, data fields, and the insert(element) method. Implement a toString method to return all nodes in MyLinkedList. Implement a recursive sorting and a non-recursive sorting method.arrow_forward
- Implement LeafNode and InteriorNode classes for the expression tree as discussed on this page Use this template: Please don't change any function namesAdd any methods if necesssaryTODO: Remove the pass statements and implement the methods. '''class LeafNode:def __init__(self, data):self.data = datadef postfix(self):return str(self)def __str__(self):return str(self.data)def prefix(self):passdef infix(self):passdef value(self):return self.dataclass InteriorNode:def __init__(self, op, left_op, right_op):self.op = opself.left_op = left_opself.right_op = right_opdef postfix(self):return self.left_op.postfix() + " " + self.right_op.postfix() + " " + self.opdef prefix(self):passdef infix(self):passdef value(self):passif __name__ == "__main__":# TODO: (Optional) your test code here.a = LeafNode(4)b = InteriorNode('+', LeafNode(2), LeafNode(3))c = InteriorNode('*', a, b)c = InteriorNode('-', c, b)arrow_forwardRedesign LaptopList class from previous project public class LaptopList { private class LaptopNode //inner class { public String brand; public double price; public LaptopNode next; public LaptopNode(String brand, double price) { // add your code } public String toString() { // add your code } } private LaptopNode head; // head of the linked list public LaptopList(String fname) throws IOException { File file = new File(fname); Scanner scan = new Scanner(file); head = null; while(scan.hasNextLine()) { // scan data // create LaptopNode // call addToHead and addToTail alternatively } } private void addToHead(LaptopNode node) { // add your code } private void addToTail(LaptopNode node) { // add your code } private…arrow_forwardImplement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 3. Define TestSLinkedList Classa. Declare an instance of the Single List class.b. Test all the methods of the Single List class.arrow_forward
- Java programming language I have to create a remove method that removes the element at an index (ind) or space in an array and returns it. Thanks! I have to write the remove method in the code below. i attached the part where i need to write it. public class ourArrayList<T>{ private Node<T> Head = null; private Node<T> Tail = null; private int size = 0; //default constructor public ourArrayList() { Head = Tail = null; size = 0; } public int size() { return size; } public boolean isEmpty() { return (size == 0); } //implement the method add, that adds to the back of the list public void add(T e) { //HW TODO and TEST //create a node and assign e to the data of that node. Node<T> N = new Node<T>();//N.mData is null and N.next is null as well N.setsData(e); //chain the new node to the list //check if the list is empty, then deal with the special case if(this.isEmpty()) { //head and tail refer to N this.Head = this.Tail = N; size++; //return we are done.…arrow_forwardCan you please answer this fast and with correct answer. Thankyouarrow_forward1.Assume some Node class with info link fields . Complete this method in class List that returns a reference to the node containing the data item in the argument findThis, Assume that findThis is in the list public class list { protected Node head ; Protected int size ; Public Node find (char find this) { Node curr = head; while(curr != null) { if(curr.info == findThis) return curr; curr = curr.link; } return -1; } }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
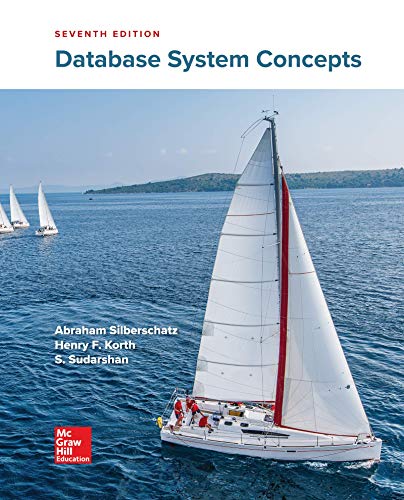
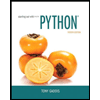
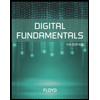
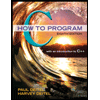
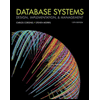
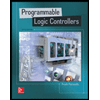