Experimenting with AES-128 symmetric key cipher.using python PART 2: you will try to use "brute force" to decrypt an encrypted message, where you only have the first 96-bits of the 128-bit secret key (last 4 bytes of the secret key are missing!) The program should be called "findk", and should deal with two files that contain the encrypted text as a stream of bytes and the first 96-bits of the key, respectively. The program should print out on standard output the missing 4 bytes of the key in hexadecimal, the decrypted message and the time it took to find the correct key. o To test this part, here is the encrypted file (ciphertext2.dat), and the first 96-bits of the key (partial-key.dat) Hint: the original message starts with the word "Salam". Answer you provided . from Crypto.Cipher import AES def revpad(s): """ This function is to remove padding. parameters: s:str string to be reverse padded """ k = ord(s[-1]) temp = 0 # temporary variable to check padding for i in range(1, k): # for loop to check padding if (s[-i] != s[-1]): # comparision of bytes with the last Byte temp = 1 if (temp == 0): return (s[:-k]) # Reverse padded string else: return ("Wrong padding") def decrypt(ct, key): """ This function is for decrypting ciphertext in ECB mode parameters: ct: str ciphertext to be decrypted key: str key for ECB mode encryption whose lenght is always a multiple of 16 in this case """ plaintxt = AES.new(key, AES.MODE_ECB) pt = plaintxt.decrypt(ct) return revpad(pt) # After decrypting padding should be removed if __name__ == "__main__": ciphertext = open("ciphertext2.dat", "r").read() partial_key = open("partial-key.dat", "r") for i in range(256): for j in range(256): for k in range(256): for l in range(256): remaining_key = chr(i) + chr(j) + chr(k) + chr(l) key = partial_key + remaining_key decrypted_text = decrypt(ciphertext, key) if (decrypted_text[:5] == "Salam"): print(decrypted_text) key = partial_key + remaining_key TypeError: unsupported operand type(s) for +: '_io.TextIOWrapper' and 'str' why the error? , please can you correct the code to give the output = should print out on standard output the missing 4 bytes of the key in hexadecimal, the decrypted message and the time it took to find the correct key. thank you in advance.
Experimenting with AES-128 symmetric key cipher.using python
PART 2: you will try to use "brute force" to decrypt an encrypted message, where you only have the first 96-bits of the 128-bit secret key (last 4 bytes of the secret key are missing!) The
o To test this part, here is the encrypted file (ciphertext2.dat), and the first 96-bits of the key (partial-key.dat) Hint: the original message starts with the word "Salam".
Answer you provided .
from Crypto.Cipher import AES
def revpad(s):
"""
This function is to remove padding.
parameters:
s:str
string to be reverse padded
"""
k = ord(s[-1])
temp = 0 # temporary variable to check padding
for i in range(1, k): # for loop to check padding
if (s[-i] != s[-1]): # comparision of bytes with the last Byte
temp = 1
if (temp == 0):
return (s[:-k]) # Reverse padded string
else:
return ("Wrong padding")
def decrypt(ct, key):
"""
This function is for decrypting ciphertext in ECB mode
parameters:
ct: str
ciphertext to be decrypted
key: str
key for ECB mode encryption whose lenght is always a multiple of 16 in this case
"""
plaintxt = AES.new(key, AES.MODE_ECB)
pt = plaintxt.decrypt(ct)
return revpad(pt) # After decrypting padding should be removed
if __name__ == "__main__":
ciphertext = open("ciphertext2.dat", "r").read()
partial_key = open("partial-key.dat", "r")
for i in range(256):
for j in range(256):
for k in range(256):
for l in range(256):
remaining_key = chr(i) + chr(j) + chr(k) + chr(l)
key = partial_key + remaining_key
decrypted_text = decrypt(ciphertext, key)
if (decrypted_text[:5] == "Salam"):
print(decrypted_text)
key = partial_key + remaining_key
TypeError: unsupported operand type(s) for +: '_io.TextIOWrapper' and 'str'
why the error? ,
please can you correct the code to give the output = should print out on standard output the missing 4 bytes of the key in hexadecimal, the decrypted message and the time it took to find the correct key.
thank you in advance.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

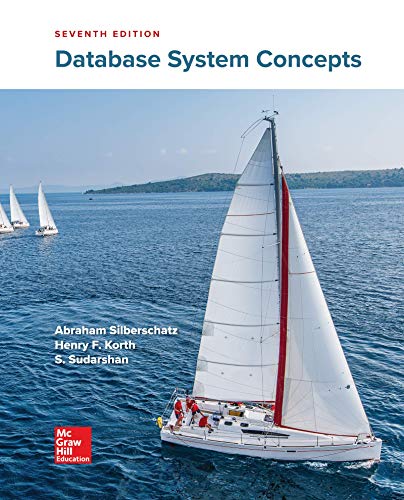
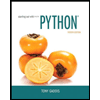
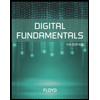
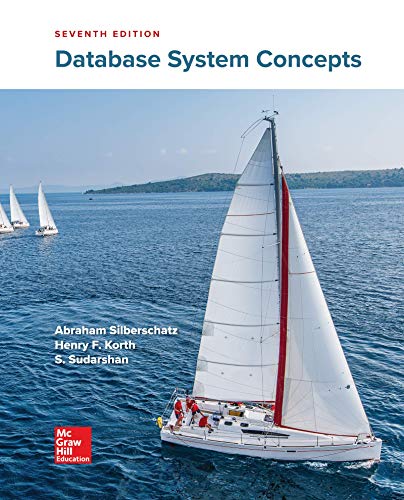
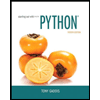
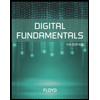
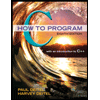
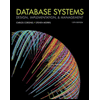
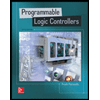