Explain the code and how it works:
Explain the code and how it works:
#include <iostream>
#include <cmath>
using namespace std;
double to_kilograms(double n)
{
return n * 0.453592;
}
double to_pounds(double n)
{
return n * 2.20462;
}
double to_kilograms_ref(double& n)
{
return n * 0.453592;
}
double to_pounds_ref(double& n)
{
return n * 2.20462;
}
double to_kilograms_ptr(double* n)
{
return *n * 0.453592;
}
double to_pounds_ptr(double* n)
{
return *n * 2.20462;
}
void display_menu()
{
cout << "1.Kilograms to Pounds (pass by value)\n2. Pounds to Kilograms (pass by value)\n3. Kilograms to Pounds (pass by reference)\n4. Pounds to Kilograms (pass by reference)\n5. Kilograms to Pounds (using pointers)\n6. Pounds to Kilograms (using pointers)\nEnter choice\n\n";
}
void convert()
{
int ch;
double w;
cout << "Enter a menu option: ";
cin >> ch;
cout << "Enter weight: ";
cin >> w;
if (ch == 1)
cout << "weight in kg is " << to_kilograms(w);
else if (ch == 2)
cout << "weight in pound is " << to_pounds(w);
else if (ch == 3)
cout << "weight in kg is " << to_kilograms_ref(w);
else if (ch == 4)
cout << "weight in pound is " << to_pounds_ref(w);
else if (ch == 5)
cout << "weight in kg is " << to_kilograms_ptr(&w);
else if (ch == 6)
cout << "weight in pound is " << to_pounds_ptr(&w);
else
cout << "wrong input";
}
int main() {
cout << "Convert Temperatures\n\n";
display_menu();
char again = 'y';
while (again == 'y')
{
convert();
cout << "\n\nConvert another weight? (y/n): ";
cin >> again;
cout << endl;
}
cout << "Bye!\n";
}

ANSWER :
Execution starts from main() function.
- First we enter into main() function then we print Convert Temperatures.
- After that we enter into next line there is display_menu()
- So, that we directly enter into display_menu() method and we execute the statements.
- Here we passes the values to the Kilograms to Pounds (pass by value), which we give the values to the function.
- And remaining all statements in the display_menu() are executed.
Step by step
Solved in 2 steps

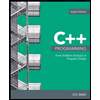
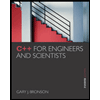
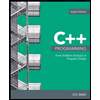
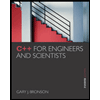