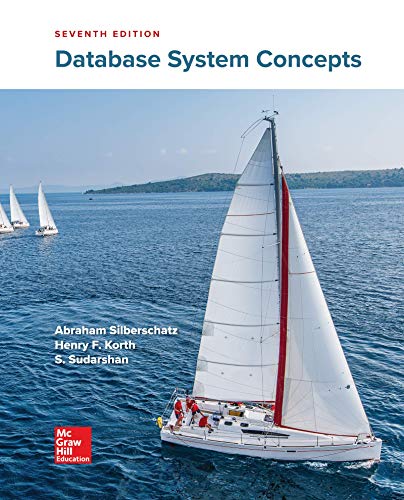
Concept explainers
Complete the program 'Bcast-Reduce-Assignment.c' (Refer to 'Bcast-Reduce-Example.c') to make the program have the output as follows if we run the program using 2 processes.
The input sequence is:
0 1 2 3
The output sequence is:
0 2 6 12
The code below must be used, please
#include <stdio.h>
#include <stdlib.h>
#include <mpi.h>
int main(void)
{
int my_rank, comm_sz;
int i;
int Count = 4;
int Number[4];
int Result[4];
MPI_Init(NULL, NULL);
MPI_Comm_size(MPI_COMM_WORLD, &comm_sz);
MPI_Comm_rank(MPI_COMM_WORLD, &my_rank);
if(my_rank == 0)
{
printf("The input sequence is: \n");
for (i = 0; i < Count; i++)
{
Number[i] = i;
printf("%d ", Number[i]);
}
printf("\n");
}
// Process 0 sends data to all of the processes
MPI_Bcast(Number, Count, MPI_INT, 0, MPI_COMM_WORLD);
for (i = 0; i < Count; i++)
Number[i] += my_rank;
MPI_Reduce( , Result, , MPI_INT, MPI_PROD, 0, MPI_COMM_WORLD);
// Print out the result
if (my_rank == 0)
{
printf("The output sequence is: \n");
printf("\n");
}
MPI_Finalize();
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Instruction: It should be a C++ PROGRAM INCLUDE THE HEADER FILE, MAIN CPP, AND BSTREE.CPP There is a real program developed by a computer company that reads a report ( running text ) and issues warnings on style and partially corrects bad style. You are to write a simplified version of this program with the following features: Statistics A statistical summary with the following information: Total number of words in the report Number of unique words Number of unique words of more than three letters Average word length Average sentence length An index (alphabetical listing) of all the unique words (see next page for a specific format) Style Warnings Issue a warning in the following cases: Word used too often: list each unique word of more than three letters if its usage is more than 5% of the total number of words of more than three letters Sentence length : write a warning message if the average sentence length is greater than 10 Word length : write a warning message if the…arrow_forwardprogram must include a processing loop that allows multiple sets of data to be processed and the program should be terminated when there is no more data to process Develop a program in python that allows the user to enter a start value of 1 to 4, a stop value of 5 to 12 and a multiplier of 2 to 8. The program must display a multiplication table with results using these values. For example, if the user enters a start value of 3, a stop value of 7 and a multiplier value of 3, the table should be displayed as follows: Multiplication Table 3 x 3 = 9 3 x 4 = 12 3 x 5 = 15 3 x 6 = 18 3 x 7 = 21 Run the program with the following values: Start Stop Multiplier 2 10 4 4 12 6arrow_forwardSubject: programming language : c++ Question : Jane has opened a new fitness center with charges of 2500 per month so the cost to become a member of a fitness center is as follow :1.For senior citizens discount is 30 %2. For young one the discount is 15 %3. For adult the discount is 20% Write a menu driven program (using structure c++) thata. Add a new memberb. Display the general information on about the fitness center and its chargesc. Determine the cost of a new membershipd. At any time show the total money made.Use appropriate parameters to pass information on and out of a function.arrow_forward
- Don't give me AI generated answer or plagiarised answer.arrow_forwardC++ Question Hello, Please create the correct code for the attached picture. Please create the code based on the given requirements. Please make sure it is working. Thank you!arrow_forwardInstructions Flowchart, implement in C++ & test the problem outlined in the problem statement. Submit the solution into Canvas. This program obtains a series of letter grades as input from a user. The program should calculate and output the total grade points and the grade point average (note: you may have to track the total number of inputs and the grade points to calculate the grade point average). The algorithm should execute 3 times for testing purposes. Use the appropriate loop for the main structure of the program that will stop accepting letter grades when the user inputs X. Also, choose the appropriate selection statement to convert the letter grade to the correct grade points.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
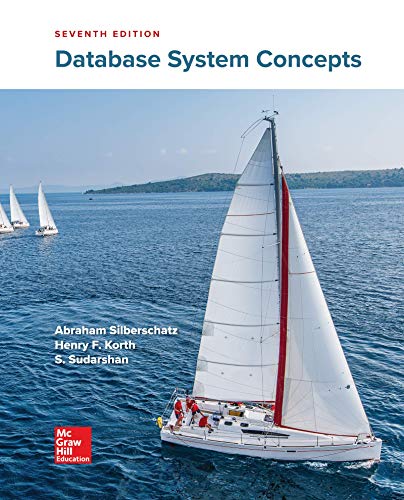
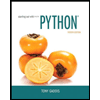
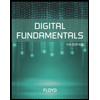
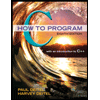
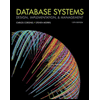
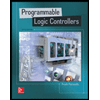