Finish this program from the code posted below! Note: There should be two files Main.py and Contact.py You will implement the edit_contact function. In the function, do the following: Ask the user to enter the name of the contact they want to edit. If the contact exists, in a loop, give them the following choices Remove one of the phone numbers from that Contact. Add a phone number to that Contact. Change that Contact's email address. Change that Contact's name (if they do this, you will have to remove the key/value pair from the dictionary and re-add it, since the key is the contact’s name. Use the dictionary's pop method for this!) Stop editing the Contact Once the user is finished making changes to the Contact, the function should return. Code: from Contact import Contact import pickle def load_contacts(): """ Unpickle the data on mydata.dat and save it to a dictionary Return an empty dictionary if the file doesn't exist """ try: with open("mydata.dat", 'rb') as file: return pickle.load(file) except FileNotFoundError: return {} def save_contacts(contacts): """ Serialize and save the data in the 'contacts' dictionary """ with open("mydata.dat", 'wb') as file: pickle.dump(contacts, file) def add(contacts): """ Ask the user to add a contact to the 'contacts' dictionary Do not allow duplicate names """ name = input("Name: ") if name in contacts: print("An entry already exists for that contact!") return email = input("Email: ") entry = Contact(name, email) # Add phone numbers to the new Contact object until the user decides to stop while True: next_num = input("Enter a phone number (or -1 to stop): ") if next_num == "-1": break entry.add_number(next_num) # Add the new Contact object to the dictionary contacts[name] = entry def look_up(contacts): """ Print the information related to the given name (if it exists in the dictionary) """ name = input("Enter a name: ") if name in contacts: print(contacts[name]) else: print("There is no contact with that name") def delete(contacts): """ Delete the contact associated with the name the user enters (if it exists in the dictionary) """ name = input("Enter a name to remove from your list of contacts: ") if name in contacts: print("Are you sure you want to delete the following contact? ") print(contacts[name]) choice = input("'y' or 'n': ") if choice == 'y': del contacts[name] else: print("Contact saved in dictionary") else: print("There is no contact with that name") def main(): contacts = load_contacts() ### Ask the user what to do next each loop iteration while True: choice = int(input("\nEnter 1) to add a contact, 2) to lookup a contact, " "3) to delete a contact, 4) to edit a contact, 5) to quit: ")) if choice == 1: add(contacts) elif choice == 2: look_up(contacts) elif choice == 3: delete(contacts) elif choice == 5: break save_contacts(contacts) main() class Contact: def __init__(self, name, email): self._name = name self._email = email self._phone_numbers = [] def add_number(self, phone_number): """ Add 'phone_number' to the internal list """ if phone_number in self._phone_numbers: print("That number is already in the list!") else: self._phone_numbers.append(phone_number) def remove_number(self, phone_number): if phone_number in self._phone_numbers: self._phone_numbers.remove(phone_number) @property def phone_numbers(self): return self._phone_numbers @property def name(self): return self._name @property def email(self): return self._email @email.setter def email(self, email): self._email = email @name.setter def name(self, name): self._name = name def __str__(self): return_string = "Name: " + self._name + "\n" return_string += "Numbers: " + "\n" for num in self._phone_numbers: return_string += "\t" + num + "\n" return_string += "Email: " + self._email return return_string
Finish this program from the code posted below! Note: There should be two files Main.py and Contact.py
You will implement the edit_contact function. In the function, do the following:
-
- Ask the user to enter the name of the contact they want to edit.
- If the contact exists, in a loop, give them the following choices
- Remove one of the phone numbers from that Contact.
- Add a phone number to that Contact.
- Change that Contact's email address.
- Change that Contact's name (if they do this, you will have to remove the key/value pair from the dictionary and re-add it, since the key is the contact’s name. Use the dictionary's pop method for this!)
- Stop editing the Contact
- Once the user is finished making changes to the Contact, the function should return.
Code:
from Contact import Contact
import pickle
def load_contacts():
""" Unpickle the data on mydata.dat and save it to a dictionary
Return an empty dictionary if the file doesn't exist """
try:
with open("mydata.dat", 'rb') as file:
return pickle.load(file)
except FileNotFoundError:
return {}
def save_contacts(contacts):
""" Serialize and save the data in the 'contacts' dictionary """
with open("mydata.dat", 'wb') as file:
pickle.dump(contacts, file)
def add(contacts):
""" Ask the user to add a contact to the 'contacts' dictionary
Do not allow duplicate names """
name = input("Name: ")
if name in contacts:
print("An entry already exists for that contact!")
return
email = input("Email: ")
entry = Contact(name, email)
# Add phone numbers to the new Contact object until the user decides to stop
while True:
next_num = input("Enter a phone number (or -1 to stop): ")
if next_num == "-1":
break
entry.add_number(next_num)
# Add the new Contact object to the dictionary
contacts[name] = entry
def look_up(contacts):
""" Print the information related to the given name (if it exists in the dictionary) """
name = input("Enter a name: ")
if name in contacts:
print(contacts[name])
else:
print("There is no contact with that name")
def delete(contacts):
""" Delete the contact associated with the name the user enters (if it exists in the dictionary) """
name = input("Enter a name to remove from your list of contacts: ")
if name in contacts:
print("Are you sure you want to delete the following contact? ")
print(contacts[name])
choice = input("'y' or 'n': ")
if choice == 'y':
del contacts[name]
else:
print("Contact saved in dictionary")
else:
print("There is no contact with that name")
def main():
contacts = load_contacts()
### Ask the user what to do next each loop iteration
while True:
choice = int(input("\nEnter 1) to add a contact, 2) to lookup a contact, "
"3) to delete a contact, 4) to edit a contact, 5) to quit: "))
if choice == 1:
add(contacts)
elif choice == 2:
look_up(contacts)
elif choice == 3:
delete(contacts)
elif choice == 5:
break
save_contacts(contacts)
main()
class Contact:
def __init__(self, name, email):
self._name = name
self._email = email
self._phone_numbers = []
def add_number(self, phone_number):
""" Add 'phone_number' to the internal list """
if phone_number in self._phone_numbers:
print("That number is already in the list!")
else:
self._phone_numbers.append(phone_number)
def remove_number(self, phone_number):
if phone_number in self._phone_numbers:
self._phone_numbers.remove(phone_number)
@property
def phone_numbers(self):
return self._phone_numbers
@property
def name(self):
return self._name
@property
def email(self):
return self._email
@email.setter
def email(self, email):
self._email = email
@name.setter
def name(self, name):
self._name = name
def __str__(self):
return_string = "Name: " + self._name + "\n"
return_string += "Numbers: " + "\n"
for num in self._phone_numbers:
return_string += "\t" + num + "\n"
return_string += "Email: " + self._email
return return_string

Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 7 images

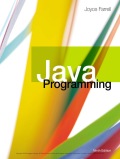
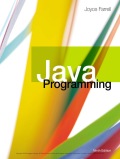