Fix the Java code below, make sure it can make the same outputs as the sample: import java.util.ArrayList; import java.util.Scanner; class Main { //Returns index of name if found otherwise -1 public static int findName(ArrayList arr, String name) { for(int i = 0; i < arr.size(); i++) if(arr.get(i).equals(name)) return i; return -1;//Name not found } //Deletes a name from ArrayList public static void deleteName(ArrayList arr, String name) { int index = findName(arr, name);//Get index of name to be deleted for(int i = index; i < arr.size() - 1; i++){ arr.set(i, arr.get(i + 1)); } arr.remove(arr.size() - 1);//Remove last element } public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.print("Enter number of students(upto 10): "); int n = scan.nextInt(); ArrayList arr = new ArrayList<>(n); for (int i = 0; i < n; i++) { System.out.print("Enter name of student " + (i+1) + ": "); arr.add(scan.next()); } System.out.print("Enter name of student to delete: "); String name = scan.next(); while(findName(arr, name) == -1)//Keep asking user for a valid name to delete { System.out.print("Name not found. Try a new name: "); name = scan.next(); } deleteName(arr, name);//Delete name from array System.out.println("Revised list of students: " + arr);//Print revised list } } Sample outputs in the picture:
Fix the Java code below, make sure it can make the same outputs as the sample:
import java.util.ArrayList;
import java.util.Scanner;
class Main
{
//Returns index of name if found otherwise -1
public static int findName(ArrayList<String> arr, String name)
{
for(int i = 0; i < arr.size(); i++)
if(arr.get(i).equals(name))
return i;
return -1;//Name not found
}
//Deletes a name from ArrayList
public static void deleteName(ArrayList<String> arr, String name)
{
int index = findName(arr, name);//Get index of name to be deleted
for(int i = index; i < arr.size() - 1; i++){
arr.set(i, arr.get(i + 1));
}
arr.remove(arr.size() - 1);//Remove last element
}
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
System.out.print("Enter number of students(upto 10): ");
int n = scan.nextInt();
ArrayList<String> arr = new ArrayList<>(n);
for (int i = 0; i < n; i++) {
System.out.print("Enter name of student " + (i+1) + ": ");
arr.add(scan.next());
}
System.out.print("Enter name of student to delete: ");
String name = scan.next();
while(findName(arr, name) == -1)//Keep asking user for a valid name to delete
{
System.out.print("Name not found. Try a new name: ");
name = scan.next();
}
deleteName(arr, name);//Delete name from array
System.out.println("Revised list of students: " + arr);//Print revised list
}
}
Sample outputs in the picture:
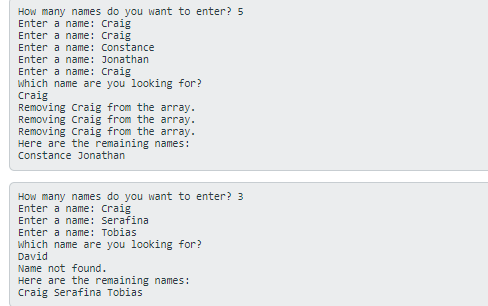

Step by step
Solved in 2 steps with 1 images

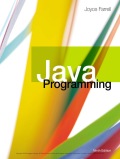
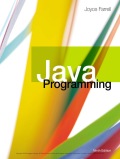