• follow_trail(filename, treasure_map, start_row, start_col): Takes as inputs a string correspond- ing to a filename, a list of lists corresponding to a treasure map, and two non-negative integers representing a row and column index. Follows the trail in the given treasure map, starting at the given row and column index. Following the trail means to look at each character of the trail and perform the appropriate operation for that character: 'v', A: Continues following the trail by moving to the character to the right, left, below or above the current trail character, respectively. - '.': Creates a new map file, with 'new_' prepended to the current map filename, and stores the same treasure map but with an X at the current position. Then, returns a tuple of three elements: the first being -1, and the latter two being the current row and column index. - *': Returns a tuple of three elements: the first being the current map number plus one, and the latter two being the current row and column index. - 'T': Returns a tuple of three elements: the first being the current map number minus one, and the latter two being the current row and column index. A digit between 0 and 9 (inclusive): Returns a tuple of three elements: the first being said digit between 0 and 9, and the latter two being 0. You can assume that the treasure map given as input will be a valid matrix and contain only the characters above. However, it's possible that there could be other issues with the treasure map. For example, a trail you follow might lead off the grid. In such case, raise an AssertionError with an appropriate error message. There are other cases of invalid treasure maps that you should also consider and raise an AssertionError for. We will leave these other cases up to you to discover. In other words, your function should never raise any exception other than AssertionError no matter what input is given for the treasure map argument (as long as it is a valid matrix and contains only the characters above). Show an example in your docstring for each different AssertionError that could be raised. These examples are in addition to the three examples that your function must normally have.
• follow_trail(filename, treasure_map, start_row, start_col): Takes as inputs a string correspond- ing to a filename, a list of lists corresponding to a treasure map, and two non-negative integers representing a row and column index. Follows the trail in the given treasure map, starting at the given row and column index. Following the trail means to look at each character of the trail and perform the appropriate operation for that character: 'v', A: Continues following the trail by moving to the character to the right, left, below or above the current trail character, respectively. - '.': Creates a new map file, with 'new_' prepended to the current map filename, and stores the same treasure map but with an X at the current position. Then, returns a tuple of three elements: the first being -1, and the latter two being the current row and column index. - *': Returns a tuple of three elements: the first being the current map number plus one, and the latter two being the current row and column index. - 'T': Returns a tuple of three elements: the first being the current map number minus one, and the latter two being the current row and column index. A digit between 0 and 9 (inclusive): Returns a tuple of three elements: the first being said digit between 0 and 9, and the latter two being 0. You can assume that the treasure map given as input will be a valid matrix and contain only the characters above. However, it's possible that there could be other issues with the treasure map. For example, a trail you follow might lead off the grid. In such case, raise an AssertionError with an appropriate error message. There are other cases of invalid treasure maps that you should also consider and raise an AssertionError for. We will leave these other cases up to you to discover. In other words, your function should never raise any exception other than AssertionError no matter what input is given for the treasure map argument (as long as it is a valid matrix and contains only the characters above). Show an example in your docstring for each different AssertionError that could be raised. These examples are in addition to the three examples that your function must normally have.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
In python please
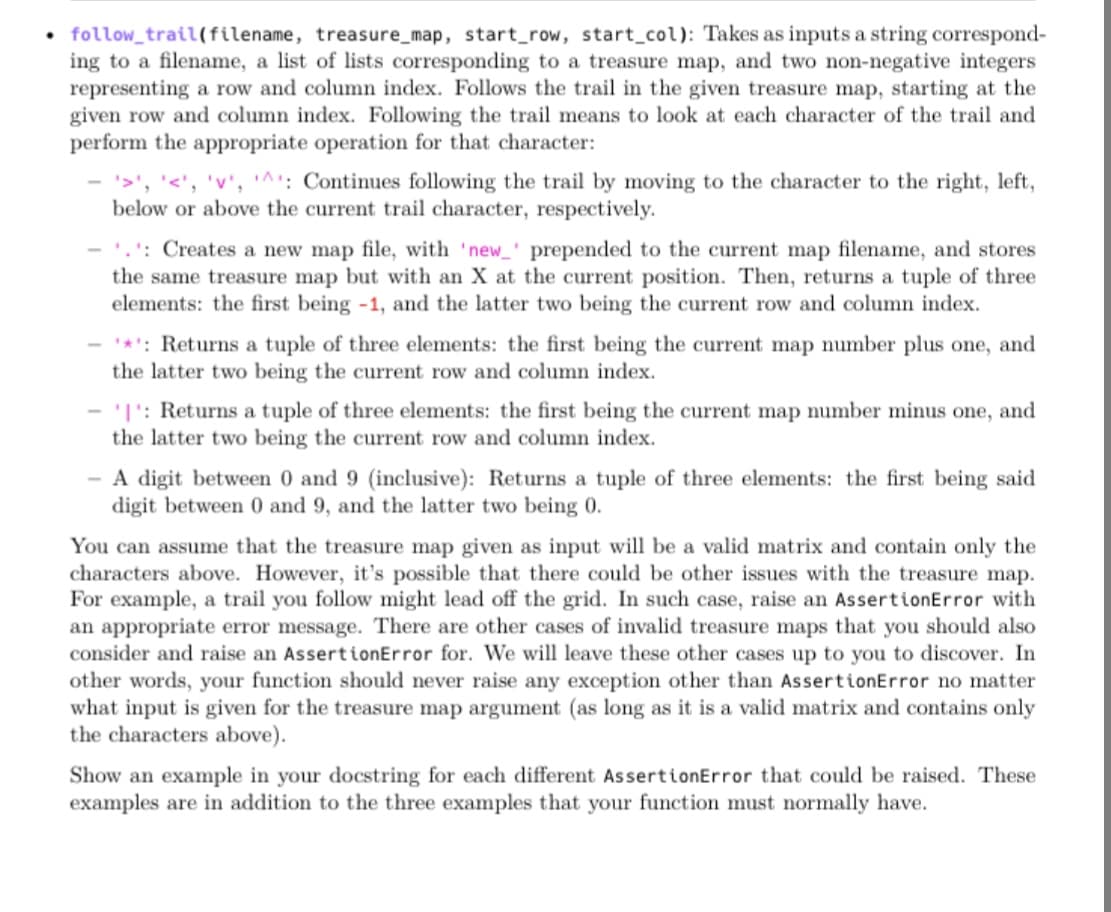
Transcribed Image Text:• follow_trail(filename, treasure_map, start_row, start_col): Takes as inputs a string correspond-
ing to a filename, a list of lists corresponding to a treasure map, and two non-negative integers
representing a row and column index. Follows the trail in the given treasure map, starting at the
given row and column index. Following the trail means to look at each character of the trail and
perform the appropriate operation for that character:
'<', 'v', '^: Continues following the trail by moving to the character to the right, left,
below or above the current trail character, respectively.
- '.': Creates a new map file, with 'new_' prepended to the current map filename, and stores
the same treasure map but with an X at the current position. Then, returns a tuple of three
elements: the first being -1, and the latter two being the current row and column index.
- *': Returns a tuple of three elements: the first being the current map number plus one, and
the latter two being the current row and column index.
'I': Returns a tuple of three elements: the first being the current map number minus one, and
the latter two being the current row and column index.
- A digit between 0 and 9 (inclusive): Returns a tuple of three elements: the first being said
digit between 0 and 9, and the latter two being 0.
You can assume that the treasure map given as input will be a valid matrix and contain only the
characters above. However, it's possible that there could be other issues with the treasure map.
For example, a trail you follow might lead off the grid. In such case, raise an AssertionError with
an appropriate error message. There are other cases of invalid treasure maps that you should also
consider and raise an AssertionError for. We will leave these other cases up to you to discover. In
other words, your function should never raise any exception other than AssertionError no matter
what input is given for the treasure map argument (as long as it is a valid matrix and contains only
the characters above).
Show an example in your docstring for each different AssertionError that could be raised. These
examples are in addition to the three examples that your function must normally have.
![>>> my_map = load_treasure_map('map0.txt')
>>> follow_trail('map0.txt', my_map, 0, 0)
|(1, 4, 5)
>>> my_map = load_treasure_map('map1.txt')
>>> follow_trail('map1.txt', my_map, 4, 5)
(8, 0, 0)
>>> my_map = load_treasure_map( 'map8.txt')
>>> follow_trail('map8.txt', my_map, 0, 0)
(-1, 3, 6)
>>> my_map = load_treasure_map('new_map8.txt')
>>> my_map[3][6]
'X'](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4f00f67d-abe4-4062-b2e6-94501e662452%2F21289242-b0ee-4062-a751-4d77749619de%2Fp33b3he_processed.jpeg&w=3840&q=75)
Transcribed Image Text:>>> my_map = load_treasure_map('map0.txt')
>>> follow_trail('map0.txt', my_map, 0, 0)
|(1, 4, 5)
>>> my_map = load_treasure_map('map1.txt')
>>> follow_trail('map1.txt', my_map, 4, 5)
(8, 0, 0)
>>> my_map = load_treasure_map( 'map8.txt')
>>> follow_trail('map8.txt', my_map, 0, 0)
(-1, 3, 6)
>>> my_map = load_treasure_map('new_map8.txt')
>>> my_map[3][6]
'X'
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
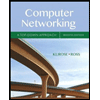
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
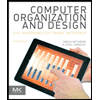
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
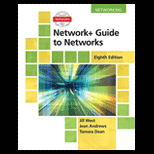
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
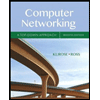
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
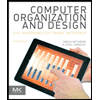
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
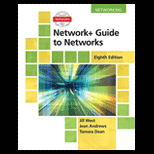
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
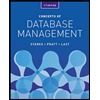
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
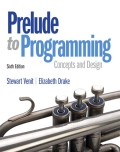
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
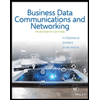
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY