load_treasure_map(filename): Takes a string as input corresponding to a filename. Opens the treasure map at that filename and loads the treasure map into a list of lists, then returns said list of lists. You can assume that the file will exist. Note that the treasure map could have any number of rows and columns. If there is any issue with the format of the file (e.g., it is not a matrix, or contains any invalid characters), then raise an AssertionError with an appropriate error message. Note that an 'X' in a file is valid. Note: In the example below, we show each row of the resulting list on its own line, but when you use doctest, you must put all the rows of the list into one line of code, as doctest checks for any improper whitespace. |>>> load_treasure_map('map0.txt') [['>', [ ['v', ['v' ['v', write_treasure_map(treasure_map, filename): Takes as inputs a list of lists corresponding to a treasure map and a string corresponding to a filename. Writes the treasure map to a file at the given filename, with a newline after each row of the map. Does not return anything. >>> my_map = load_treasure_map('map0.txt') |>>> write_treasure_map(my_map, 'new_map.txt') |>>> my_map2 = load_treasure_map('new_map.txt') |>>> my_map == my_map2 True
load_treasure_map(filename): Takes a string as input corresponding to a filename. Opens the treasure map at that filename and loads the treasure map into a list of lists, then returns said list of lists. You can assume that the file will exist. Note that the treasure map could have any number of rows and columns. If there is any issue with the format of the file (e.g., it is not a matrix, or contains any invalid characters), then raise an AssertionError with an appropriate error message. Note that an 'X' in a file is valid. Note: In the example below, we show each row of the resulting list on its own line, but when you use doctest, you must put all the rows of the list into one line of code, as doctest checks for any improper whitespace. |>>> load_treasure_map('map0.txt') [['>', [ ['v', ['v' ['v', write_treasure_map(treasure_map, filename): Takes as inputs a list of lists corresponding to a treasure map and a string corresponding to a filename. Writes the treasure map to a file at the given filename, with a newline after each row of the map. Does not return anything. >>> my_map = load_treasure_map('map0.txt') |>>> write_treasure_map(my_map, 'new_map.txt') |>>> my_map2 = load_treasure_map('new_map.txt') |>>> my_map == my_map2 True
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
write two functions:
load_treasure_map(filename): Takes a string as input corresponding to a filename. Opens the treasure map at that filename and loads the treasure map into a list of lists, then returns said list of lists. You can assume that the file will exist. Note that the treasure map could have any number of rows and columns
write_treasure_map(treasure_map, filename): Takes as inputs a list of lists corresponding to a treasure map and a string corresponding to a filename. Writes the treasure map to a file at the given filename, with a newline after each row of the map. Does not return anything.
![• Load_treasure_map(filename): Takes a string as input corresponding to a filename. Opens the
treasure map at that filename and loads the treasure map into a list of lists, then returns said list
of lists. You can assume that the file will exist. Note that the treasure map could have any number
of rows and columns.
If there is any issue with the format of the file (e.g., it is not a matrix, or contains any invalid
characters), then raise an AssertionError with an appropriate error message. Note that an 'X' in
a file is valid.
Note: In the example below, we show each row of the resulting list on its own line, but when you
use doctest, you must put all the rows of the list into one line of code, as doctest checks for any
improper whitespace.
>>> load_treasure_map( 'map0.txt')
[['>',
'v'
['.'
['.',
'],
['v',
['v',
['v',
'1]
• write_treasure_map(treasure_map, filename): Takes as inputs a list of lists corresponding to a
treasure map and a string corresponding to a filename. Writes the treasure map to a file at the
given filename, with a newline after each row of the map. Does not return anything.
>>> my_map = load_treasure_map('map0.txt')
>>> write_treasure_map(my_map, 'new_map.txt')
>>> my_map2 = load_treasure_map('new_map.txt')
>>> my_map == my_map2
True](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9c002e4e-ece1-4dd1-9934-cbb442392244%2F0ae684ab-7186-4c90-8b32-14a95faba309%2Fx91st76_processed.png&w=3840&q=75)
Transcribed Image Text:• Load_treasure_map(filename): Takes a string as input corresponding to a filename. Opens the
treasure map at that filename and loads the treasure map into a list of lists, then returns said list
of lists. You can assume that the file will exist. Note that the treasure map could have any number
of rows and columns.
If there is any issue with the format of the file (e.g., it is not a matrix, or contains any invalid
characters), then raise an AssertionError with an appropriate error message. Note that an 'X' in
a file is valid.
Note: In the example below, we show each row of the resulting list on its own line, but when you
use doctest, you must put all the rows of the list into one line of code, as doctest checks for any
improper whitespace.
>>> load_treasure_map( 'map0.txt')
[['>',
'v'
['.'
['.',
'],
['v',
['v',
['v',
'1]
• write_treasure_map(treasure_map, filename): Takes as inputs a list of lists corresponding to a
treasure map and a string corresponding to a filename. Writes the treasure map to a file at the
given filename, with a newline after each row of the map. Does not return anything.
>>> my_map = load_treasure_map('map0.txt')
>>> write_treasure_map(my_map, 'new_map.txt')
>>> my_map2 = load_treasure_map('new_map.txt')
>>> my_map == my_map2
True
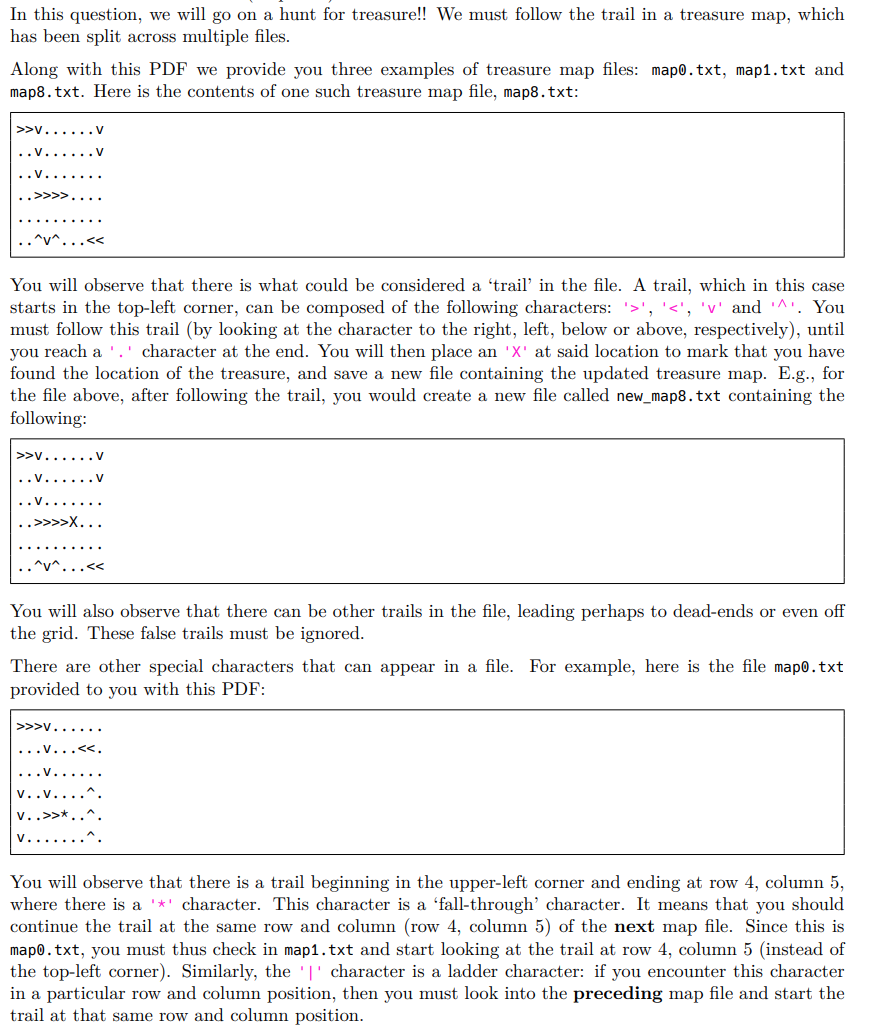
Transcribed Image Text:In this question, we will go on a hunt for treasure!! We must follow the trail in a treasure map, which
has been split across multiple files.
Along with this PDF we provide you three examples of treasure map files: map0.txt, map1.txt and
map8.txt. Here is the contents of one such treasure map file, map8.txt:
>>v......V
^v^.
You will observe that there is what could be considered a 'trail' in the file. A trail, which in this case
starts in the top-left corner, can be composed of the following characters: '>', '<', 'v' and '^'. You
must follow this trail (by looking at the character to the right, left, below or above, respectively), until
you reach a .' character at the end. You will then place an 'X' at said location to mark that you have
found the location of the treasure, and save a new file containing the updated treasure map. E.g., for
the file above, after following the trail, you would create a new file called new_map8.txt containing the
following:
>>v.
..v...... V
..>>>>X..
.^v^
You will also observe that there can be other trails in the file, leading perhaps to dead-ends or even off
the grid. These false trails must be ignored.
There are other special characters that can appear in a file. For example, here is the file map0.txt
provided to you with this PDF:
>>>v......
...v...
v..v.
v..>>*
You will observe that there is a trail beginning in the upper-left corner and ending at row 4, column 5,
where there is a '*' character. This character is a 'fall-through' character. It means that you should
continue the trail at the same row and column (row 4, column 5) of the next map file. Since this is
mapo.txt, you must thus check in map1.txt and start looking at the trail at row 4, column 5 (instead of
the top-left corner). Similarly, the '|' character is a ladder character: if you encounter this character
in a particular row and column position, then you must look into the preceding map file and start the
trail at that same row and column position.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
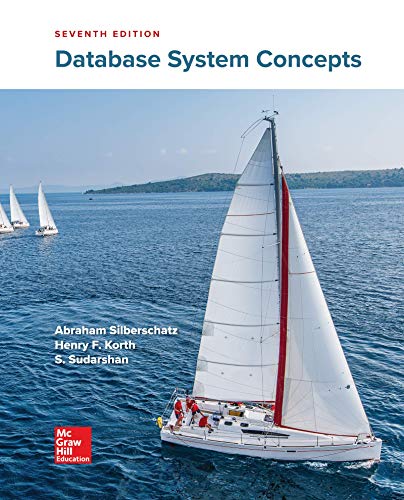
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
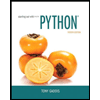
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
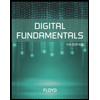
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
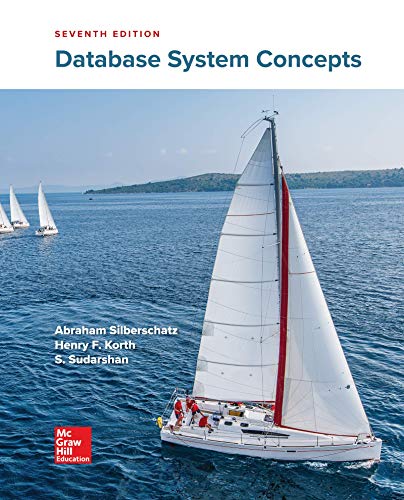
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
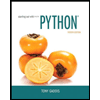
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
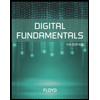
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
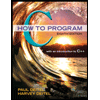
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
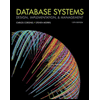
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
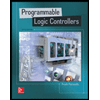
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education