For a parking management system, use the Observer pattern to implement this interaction using Java and implement the three classes mentioned below. Implement a ParkingObserver that has the role of the Observer. The Observable classes (Subjects) will be the ParkingLots. It would be helpful if the notify method in the subjects (and update method in the observers) took a parameter, perhaps an object of type ParkingEvent, which could have fields including the lot, timeIn, timeOut (if relevant), and permit. The ParkingObserver should register with each ParkingLot and receive the messages. Once a car enters (in an entry-scan only lot) or leaves (in an entry-scan and exit-scan lot), then the ParkingObserver will be updated, and then can register the charge with the parking system via the TransactionManager’s park() method. Based on these requirements you should implement three actor classes in this interaction using java: TransactionManager, ParkingObserver and ParkingLot. 1. The TransactionManager knows nothing of the other two classes but it has a park() method that could take a ParkingEvent. 2. The ParkingObserver class will have a reference to the TransactionManager instance (so that it can call park()) and it will have an update() method that could take a ParkingEvent. 3. Each ParkingLot will have a reference to a ParkingObserver, or list of ParkingObservers (they could all have the same instance or there could be one instance for each ParkingLot). When someone enters or exits the parking lot, the ParkingLot instance will call the update() method of ParkingObserver. Include small description to your code design.
For a parking management system, use the Observer pattern to implement this interaction using Java and implement the three classes mentioned below.
Implement a ParkingObserver that has the role of the Observer. The Observable classes (Subjects) will be the ParkingLots. It would be helpful if the notify method in the subjects (and update method in the observers) took a parameter, perhaps an object of type ParkingEvent, which could have fields including the lot, timeIn, timeOut (if relevant), and permit. The ParkingObserver should register with each ParkingLot and receive the messages. Once a car enters (in an entry-scan only lot) or leaves (in an entry-scan and exit-scan lot), then the ParkingObserver will be updated, and then can register the charge with the parking system via the TransactionManager’s park() method.
Based on these requirements you should implement three actor classes in this interaction using java: TransactionManager, ParkingObserver and ParkingLot.
1. The TransactionManager knows nothing of the other two classes but it has a park() method that could take a ParkingEvent.
2. The ParkingObserver class will have a reference to the TransactionManager instance (so that it can call park()) and it will have an update() method that could take a ParkingEvent.
3. Each ParkingLot will have a reference to a ParkingObserver, or list of ParkingObservers (they could all have the same instance or there could be one instance for each ParkingLot). When someone enters or exits the parking lot, the ParkingLot instance will call the update() method of ParkingObserver.
Include small description to your code design.
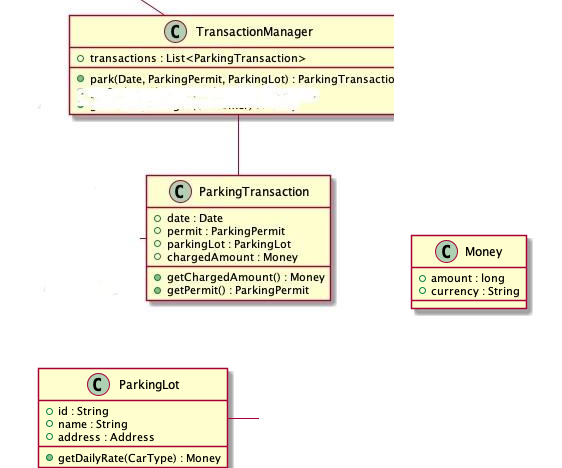

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

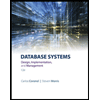
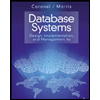
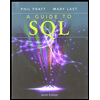
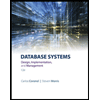
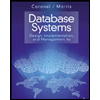
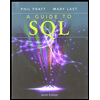
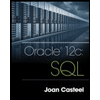