Your task will be to create a simple text-based simulation that inherits from a pre-existing World class. You must use the World.h provided as a starting point for your code. At least one object in your solution should meaningfully inherit from this class. DO NOT MODIFY THE WORLD CLASS in any way. Note that the World class is abstract and there are a few pure virtual functions for you to implement in your object that inherits from the world. Namely, you will need to implement: void PrintGrid() - This function should print the grid (10 by 10 char array) to standard out.
Your task will be to create a simple text-based simulation that inherits from a pre-existing World class.
You must use the World.h provided as a starting point for your code. At least one object in your solution should meaningfully inherit from this class. DO NOT MODIFY THE WORLD CLASS in any way. Note that the World class is abstract and there are a few pure virtual functions for you to implement in your object that inherits from the world. Namely, you will need to implement:
- void PrintGrid() - This function should print the grid (10 by 10 char array) to standard out.
- void UpdateGrid() - This function should update your characters' positions on the grid
- void initGrid() - This function should set your grid up (implement your "starting conditions" here)
The scenarios that you will simulate will be confined to a 10x10 grid (defined in the World class as grid) and be subject to at least 10 distinct rules. In addition, there must be at least 2 different objects or “characters” interacting in your simulation. Each should be defined in their own class. Initially, characters should be spawned in random positions in the grid. Their movement on each day (or round of simulation) should be governed by the rules that you develop. After each day or round of your simulation, an updated grid showing the position of all characters should be printed to the standard output. There should be some clear stopping condition of your simulation. In addition, in at least one of your objects should overload at least two operators in a meaningful way. Ex. You may choose to overload your + operator to determine what will result if two of your characters interact. Your program should not contain any memory leaks. Where appropriate, you should use the const keyword.
Example Scenario:
Life Simulation
Starting Condition: The simulation will start with 10 Humans, age 0 with immunity of 1, (5 female and 5 male) and 1 Virus object randomly placed in the grid. Each round of the program will symbolize a year passed.
Example Rules:
- Every year a Human object can make one random move vertically, horizontally or diagonally in the grid.
- If two Human objects come in contact with one another then if they are of the opposite gender, are unmarried and over age 18 then they will spawn another Human object somewhere in the grid (gender will be randomly assigned).
- Every 10 years another Virus object appears on the grid in a random spot.
- If a Human object comes in contact with a Virus object then if their immunity value is greater than 0.25 then a number between 0 and 1 will be randomly calculated and subtracted from the Human’s immunity value.
- If a Human object comes in contact with a Virus object then if their immunity value is less than 0.25 then they will disappear from the grid.
- If the randomly generated move for any Human is illegal (out of bounds) then no move is made. ...
Stopping Condition: The 10x10 grid has filled up or 1000 years (rounds) have passed.
Checklist (and points)
- Inherit from the World class provided (5 points)
- Implement the 3 pure virtual functions in the World class (6 points - 2 points each)
- Implement 10 distinct rules (30 points - 3 points each)
- Include at least 2 other objects as "characters" in your sim (10 points - 5 for each class)
- Make sure that there is an option for printing each iteration of the sim to standard out (5 points)
- Make sure there is a clear stopping condition (5 points)
- Overload at least two operators in at least one of your objects (4 points - 2 points each)
- No memory leaks if you choose to use pointers in any way (-5 points for memory leaks)
- Where appropriate, use the const keyword. (-1-5 points if missing consts - depends on how many you are missing)
Example of PrintGrid()
void Gameboard::PrintGrid()
{
cout << "World at Day " << day << endl;
cout << " ---+---+---+---+---+---+---+--- " << endl;
for(int i = 0; i < 10; i++) {
cout << "| "
}
for(int j = 0; j < 10; j++) {
cout << grid[i][j] << " | "; }
cout << endl; cout << " ---+---+---+---+---+---+---+--- " << endl;
}
}
#ifndef WORLD_H | |
#define WORLD_H | |
#include <cstdlib> | |
class World { | |
public: | |
World() {}; | |
virtual void PrintGrid() = 0; | |
virtual void UpdateGrid() = 0; | |
protected: | |
int day; | |
char grid[10][10]; | |
virtual void initGrid() = 0; | |
}; | |
#endif /* WORLD_H */ |

Step by step
Solved in 6 steps

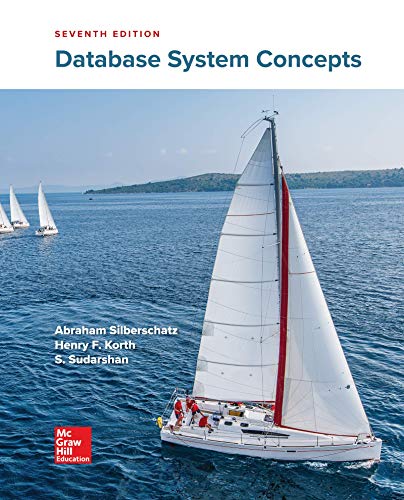
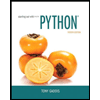
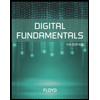
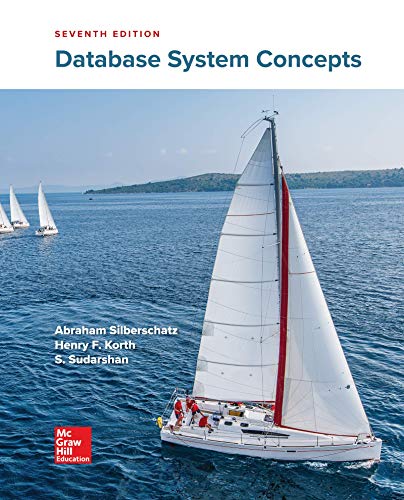
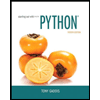
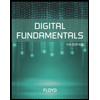
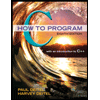
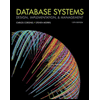
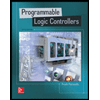