For question 4 in this lab, you wrote a program that prompted the user to enter a comma separated line of integers. The program split this line and obtained a list of integer values. It then processed this list of integer values by comparing each pair in the list and swapping them if they were out of order. In effect, the largest integer in the list was "bubbled" to the end of the list. The following question is based on this. Define a function called bubble_largest_value() that takes a single parameter - a list of integers called integer_list. The function processes this list of integers so that the largest integer in this list is bubbled to the end. This is done by comparing each pair of integers in the list. If the integer at index i is larger than the integer at index i + 1, then the program swaps the two values so that the larger value is at index i+ 1 and the smaller value at index i. Note: • The function does not return a new list. It modifies the list passed to it as an argument. • You can assume that the list passed as an argument to the function contains at least 1 integer item. Some examples of the function being called are shown below. For example: Test integer_list = [5,4,9,2,1,8,-1] bubble_largest_value(integer_list) print("List of integers:", integer_list) integer_list = [1,2,3,4,5,6,7,8,9] bubble_largest_value(integer_list) print("List of integers:", integer_list) Result List of integers: [4, 5, 2, 1, 8, -1, 9] Reset answer List of integers: [1, 2, 3, 4, 5, 6, 7, 8, 9] Answer: (penalty regime: 0, 0, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50 %) 1|def bubble_largest_value(integer_list):
For question 4 in this lab, you wrote a program that prompted the user to enter a comma separated line of integers. The program split this line and obtained a list of integer values. It then processed this list of integer values by comparing each pair in the list and swapping them if they were out of order. In effect, the largest integer in the list was "bubbled" to the end of the list. The following question is based on this. Define a function called bubble_largest_value() that takes a single parameter - a list of integers called integer_list. The function processes this list of integers so that the largest integer in this list is bubbled to the end. This is done by comparing each pair of integers in the list. If the integer at index i is larger than the integer at index i + 1, then the program swaps the two values so that the larger value is at index i+ 1 and the smaller value at index i. Note: • The function does not return a new list. It modifies the list passed to it as an argument. • You can assume that the list passed as an argument to the function contains at least 1 integer item. Some examples of the function being called are shown below. For example: Test integer_list = [5,4,9,2,1,8,-1] bubble_largest_value(integer_list) print("List of integers:", integer_list) integer_list = [1,2,3,4,5,6,7,8,9] bubble_largest_value(integer_list) print("List of integers:", integer_list) Result List of integers: [4, 5, 2, 1, 8, -1, 9] Reset answer List of integers: [1, 2, 3, 4, 5, 6, 7, 8, 9] Answer: (penalty regime: 0, 0, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50 %) 1|def bubble_largest_value(integer_list):
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Python with output screenshot please.
![For question 4 in this lab, you wrote a program that prompted the user to enter a comma separated line of integers.
The program split this line and obtained a list of integer values. It then processed this list of integer values by
comparing each pair in the list and swapping them if they were out of order. In effect, the largest integer in the list was
"bubbled" to the end of the list. The following question is based on this.
Define a function called bubble_largest_value() that takes a single parameter - a list of integers called
integer_list. The function processes this list of integers so that the largest integer in this list is bubbled to the end.
This is done by comparing each pair of integers in the list. If the integer at index i is larger than the integer at index i
+ 1, then the program swaps the two values so that the larger value is at index i+ 1 and the smaller value at index
i.
Note:
• The function does not return a new list. It modifies the list passed to it as an argument.
• You can assume that the list passed as an argument to the function contains at least 1 integer item.
Some examples of the function being called are shown below.
For example:
Test
integer_list = [5,4,9,2,1,8,-1]
bubble_largest_value(integer_list)
print("List of integers:", integer_list)
integer_list = [1,2,3,4,5,6,7,8,9]
bubble_largest_value(integer_list)
print("List of integers:", integer_list)
Result
Reset answer
List of integers: [4, 5, 2, 1, 8, -1, 9]
List of integers: [1, 2, 3, 4, 5, 6, 7, 8, 9]
Answer: (penalty regime: 0, 0, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50 %)
1 def bubble_largest_value(integer_list):](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5c618048-b4b5-4c2a-a8b3-e4d9ef6f9c6a%2F4e10defe-e790-4944-b18a-eec68c63f277%2Fh2upmab_processed.png&w=3840&q=75)
Transcribed Image Text:For question 4 in this lab, you wrote a program that prompted the user to enter a comma separated line of integers.
The program split this line and obtained a list of integer values. It then processed this list of integer values by
comparing each pair in the list and swapping them if they were out of order. In effect, the largest integer in the list was
"bubbled" to the end of the list. The following question is based on this.
Define a function called bubble_largest_value() that takes a single parameter - a list of integers called
integer_list. The function processes this list of integers so that the largest integer in this list is bubbled to the end.
This is done by comparing each pair of integers in the list. If the integer at index i is larger than the integer at index i
+ 1, then the program swaps the two values so that the larger value is at index i+ 1 and the smaller value at index
i.
Note:
• The function does not return a new list. It modifies the list passed to it as an argument.
• You can assume that the list passed as an argument to the function contains at least 1 integer item.
Some examples of the function being called are shown below.
For example:
Test
integer_list = [5,4,9,2,1,8,-1]
bubble_largest_value(integer_list)
print("List of integers:", integer_list)
integer_list = [1,2,3,4,5,6,7,8,9]
bubble_largest_value(integer_list)
print("List of integers:", integer_list)
Result
Reset answer
List of integers: [4, 5, 2, 1, 8, -1, 9]
List of integers: [1, 2, 3, 4, 5, 6, 7, 8, 9]
Answer: (penalty regime: 0, 0, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50 %)
1 def bubble_largest_value(integer_list):
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
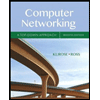
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
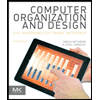
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
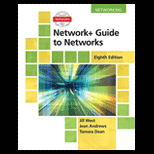
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
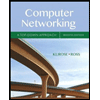
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
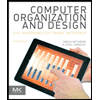
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
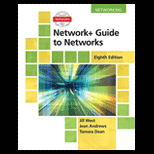
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
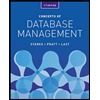
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
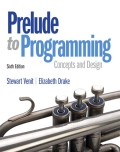
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
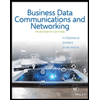
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY