For some reason I have 2 programs that aren't running. This is the second one, please let me know if everything is correct. (And I have tried changing class to main but that doesn't work) My code: class Program12two { //PSEUDOCODE //Write a program that creates a small (6-10) array of ints //Display your array elements, all on one line, using a foreach loop //In a try block, prompt the user to enter an index for the array and attempt to print the element with that index //Follow the try block with two catch blocks: //one that detects an index out of bounds //another that catches other bad inputs public static void main(String[] args) { Scanner in = new Scanner(System.in); int[] arr = {12, 15, 24, 5, 9, 16}; for (int num: arr) { System.out.print(num + " "); } System.out.println(); try { System.out.print("Enter any index of your array "); int index = Integer.parseInt(in.next()); int num = arr[index]; System.out.println("Element at index " + index + " is " + num); } catch (IndexOutOfBoundsException e) { System.out.println("Error. Array index was out of bounds"); } catch (Exception e) { System.out.println("Bad input. Try again"); } in.close(); } }
For some reason I have 2 programs that aren't running. This is the second one, please let me know if everything is correct. (And I have tried changing class to main but that doesn't work)
My code:
class Program12two {
//PSEUDOCODE
//Write a
//Display your array elements, all on one line, using a foreach loop
//In a try block, prompt the user to enter an index for the array and attempt to print the element with that index
//Follow the try block with two catch blocks:
//one that detects an index out of bounds
//another that catches other bad inputs
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int[] arr = {12, 15, 24, 5, 9, 16};
for (int num: arr) {
System.out.print(num + " ");
}
System.out.println();
try {
System.out.print("Enter any index of your array ");
int index = Integer.parseInt(in.next());
int num = arr[index];
System.out.println("Element at index " + index + " is " + num);
} catch (IndexOutOfBoundsException e) {
System.out.println("Error. Array index was out of bounds");
} catch (Exception e) {
System.out.println("Bad input. Try again");
}
in.close();
}
}

Step by step
Solved in 2 steps with 1 images

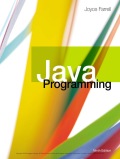
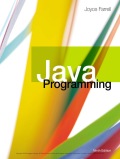