Hello, I am having trouble with this homework question for my c++ course. **It is saying to use the int main provided - I have included it in the snip along with the correct output snip. 1. Implement a class IntArr using dynamic memory. a. data members: capacity: maximum number of elements in the array size: current number of elements in the array array: a pointer to a dynamic array of integers b. constructors: default constructor: capacity and size are 0, array pointer is nullptr user constructor: create a dynamic array of the specified size c. overloaded operators: subscript operator: return an element or exits if illegal index d. “the big three”: copy constructor: construct an IntArr object using deep copy assignment overload operator: deep copy from one object to another destructor: destroys an object without creating a memory leak e. grow function: “grow” the array to twice its capacity f. push_back function: add a new integer to the end of the array g. pop_back function: remove the last element in the array h. getSize function: return the current size of the array (not capacity) i. Use the provided main function (next page) and output example Notes a. grow function replaces the existing array with a new array b. subscript operator should be a const member function c. ensure that deep copy is used where applicable d. push_back and pop_back functions require the grow function e. push_back and pop_back functions should update array size (not capacity)
Hello, I am having trouble with this homework question for my c++ course.
**It is saying to use the int main provided - I have included it in the snip along with the correct output snip.
1. Implement a class IntArr using dynamic memory.
a. data members:
capacity: maximum number of elements in the array
size: current number of elements in the array
array: a pointer to a dynamic array of integers
b. constructors:
default constructor: capacity and size are 0, array pointer is nullptr
user constructor: create a dynamic array of the specified size
c. overloaded operators:
subscript operator: return an element or exits if illegal index
d. “the big three”:
copy constructor: construct an IntArr object using deep copy
assignment overload operator: deep copy from one object to another
destructor: destroys an object without creating a memory leak
e. grow function: “grow” the array to twice its capacity
f. push_back function: add a new integer to the end of the array
g. pop_back function: remove the last element in the array
h. getSize function: return the current size of the array (not capacity)
i. Use the provided main function (next page) and output example
Notes
a. grow function replaces the existing array with a new array
b. subscript operator should be a const member function
c. ensure that deep copy is used where applicable
d. push_back and pop_back functions require the grow function
e. push_back and pop_back functions should update array size (not capacity)
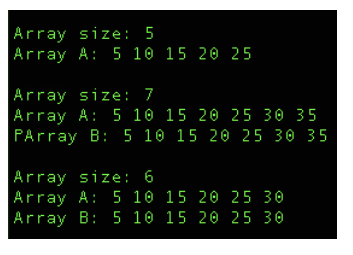
![int main() {
cout << endl;
IntArr a{5};
for(int i=0; i<5; i++) { a.push_back((1+1)*5); }
cout « "Array size: " « a.getSize() « endl;
cout « "Array A: ";
for(int i=0; i<a.getSize(); i++) { cout << a[i] << " "; }
cout << endl « endl;
a. push_back(30);
a.push_back(35);
cout « "Array size: " « a.getSize() « endl;
<<
IntArr b = a;
cout <« "Array A: ";
for(int i=0; i<a.getSize(); i++) { cout <« a[i] << " "; }
cout << "\nPArray B: ";
for(int i=0; i<b.getSize(); i++) { cout « b[i] « " "; }
cout <« endl « endl;
a. pop_back();
cout <« "Array size: " <« a.getSize() <« endl;
b = a;
cout « "Array A: ";
for(int i=0; i<a.getSize(); i++)
cout <« ali] «" "; }
cout <« "\nArray B: ";
for(int i=0; i<b.getSize(); i++){ cout « b[i] « " "; }
cout « endl « endl;
cout << endl;
return 8;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F86bfc21f-7304-4d6a-8ae3-de059a5180e7%2F96f2a1f2-c501-4007-a196-7dc66f6f45e6%2Fe209t0l_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

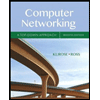
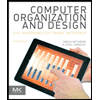
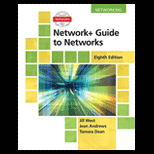
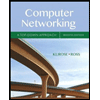
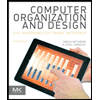
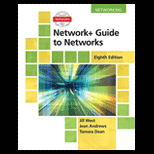
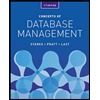
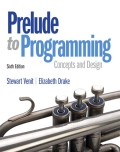
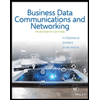