•For the following algorithm, explain what it computes, state what the input size for analysis is, state what basic operations should be counted for analyzing it, state exactly how many operations are executed as a function of the input size, and state the efficiency class or classes to which it belongs. The built-in C++ swap function is extremely efficient, and you should count it as performing exactly two basic operations. 01 void foo(vector& array) 02 { size_t size - array.size(); for (size_t pass_indx = 0; pass_indx < size - 1; pass_indx++) { size_t min_position = pass_indx; for (size_t compare_indx = pass_indx + 1; compare_indx < size; compare_indx++) { if (array.at(compare_indx) < array.at (min_position)) { min_position - compare_indx; } } 03 04 05 06 07 08 09 10 11 12 13 if (min_position != pass_indx) { swap (array.at(pass_indx), array.at (min_position)); 17 18 19
•For the following algorithm, explain what it computes, state what the input size for analysis is, state what basic operations should be counted for analyzing it, state exactly how many operations are executed as a function of the input size, and state the efficiency class or classes to which it belongs. The built-in C++ swap function is extremely efficient, and you should count it as performing exactly two basic operations. 01 void foo(vector& array) 02 { size_t size - array.size(); for (size_t pass_indx = 0; pass_indx < size - 1; pass_indx++) { size_t min_position = pass_indx; for (size_t compare_indx = pass_indx + 1; compare_indx < size; compare_indx++) { if (array.at(compare_indx) < array.at (min_position)) { min_position - compare_indx; } } 03 04 05 06 07 08 09 10 11 12 13 if (min_position != pass_indx) { swap (array.at(pass_indx), array.at (min_position)); 17 18 19
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter16: Searching, Sorting And Vector Type
Section: Chapter Questions
Problem 16SA
Related questions
Question
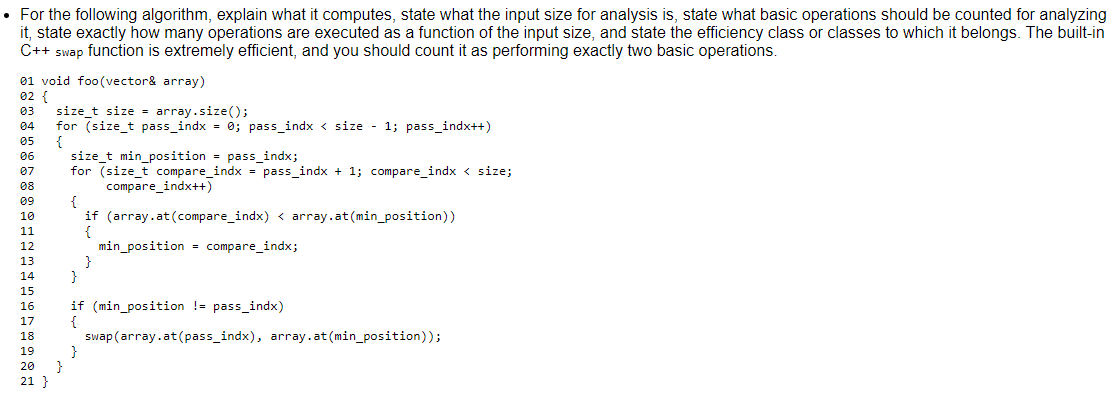
Transcribed Image Text:• For the following algorithm, explain what it computes, state what the input size for analysis is, state what basic operations should be counted for analyzing
it, state exactly how many operations are executed as a function of the input size, and state the efficiency class or classes to which it belongs. The built-in
C++ swap function is extremely efficient, and you should count it as performing exactly two basic operations.
01 void foo(vector& array)
02 {
size_t size = array.size();
for (size_t pass_indx = 0; pass_indx < size - 1; pass_indx++)
{
size_t min_position = pass_indx;
for (size_t compare_indx = pass_indx + 1; compare_indx < size;
compare_indx++)
{
if (array.at (compare_indx) < array.at(min_position))
{
min_position = compare_indx;
}
}
03
04
05
06
07
08
09
10
11
12
13
14
15
16
if (min_position !- pass_indx)
17
swap (array.at(pass_indx), array.at (min_position));
18
19
}
21 }
20
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
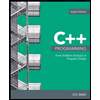
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
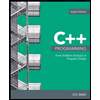
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning