For the Java code below, you will demonstrate the ability to use and call multiple methods in your program. Using the code below, create the following methods: 1. A method that converts the temperature from Fahrenheit to Celsius. The method should be passed a parameter - Fahrenheit and should return a result - Celsius. 2. A method to output the results of your temperature conversion. The method should be passed 2 parameters - temperature in Fahrenheit and the temperature in Celsius. Finally, place an edit in your code to ensure that the user only enters a numeric value. Your output should appear as follows: Enter the Fahrenheit temperature you want to convert: 212 This converts to 100 Celsius Do you want to convert another temperature – Y or N? Y Enter the Fahrenheit temperature you want to convert: 100 This converts to 37.8 Celsius Do you want to convert another temperature – Y or N? N The program has ended
For the Java code below, you will demonstrate the ability to use and call multiple methods in your program. Using the code below, create the following methods:
1. A method that converts the temperature from Fahrenheit to Celsius. The method should be passed a parameter - Fahrenheit and should return a result - Celsius.
2. A method to output the results of your temperature conversion. The method should be passed 2 parameters - temperature in Fahrenheit and the temperature in Celsius.
Finally, place an edit in your code to ensure that the user only enters a numeric value.
Your output should appear as follows:
- Enter the Fahrenheit temperature you want to convert:
- 212
- This converts to 100 Celsius
- Do you want to convert another temperature – Y or N?
- Y
- Enter the Fahrenheit temperature you want to convert:
- 100
- This converts to 37.8 Celsius
- Do you want to convert another temperature – Y or N?
- N
- The program has ended
import java.text.DecimalFormat;
import java.util.Scanner;
public class CS1210
{
public static void main(String[] args)
{
Scanner input = new Scanner(System.in);
DecimalFormat df = new DecimalFormat("#.#");
char choice;
do
{
System.out.print("Enter the Fahrenheit temperature you want to convert: ");
double fahrenheit = input.nextDouble();
double celsius = ((fahrenheit - 32.0) / 1.8);
System.out.println("This converts to " + df.format(celsius) + " Celsius");
System.out.println("Do you want to convert another temperature – Y or N?");
choice=input.next().charAt(0);
}
while (choice == 'Y');
System.out.println("The program has ended");
}
}
![1 // This Java code converts Fahrenheit to Celcius.
2
30 import java.text.DecimalFormat;
4 import java.util.Scanner;
6 public class CS1210
7
8 {
public static void main(String[] args)
{
Scanner input
10
C611
12
13
14
15
16
17
= new Scanner(System.in);
= new DecimalFormat("#.#");
DecimalFormat df :
char choice;
do
{
System.out.print("Enter the Fahrenheit temperature you want to convert: ");
18
double fahrenheit
input.nextDouble();
19
20
21
22
23
24
25
26
27
double celsius =
((fahrenheit - 32.0) / 1.8);
System.out.println("This converts to
+ df.format(celsius) +
Celsius");
Y or N?");
System.out.println("Do you want to convert another temperature
choice=input.next().charAt(0);
}
'Y');
while (choice
System.out.println("The program has ended");
==
28
29
30
}
31 }
32
A Problems @ Javadoc E Declaration E Console X
CS1210 [Java Application] C:\Users|Victor\AppData\Local\Temp\eoiBDB9.tmp\plugins\org.eclipse.justj.openjdk.hotspot.jre.minimal
Enter the Fahrenheit temperature you want to convert:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F467da151-d8cf-4743-8497-8e02aaa91ea3%2Fb507b500-b4e0-4744-bc1e-4731f1edfbe1%2Fqcnk416h_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

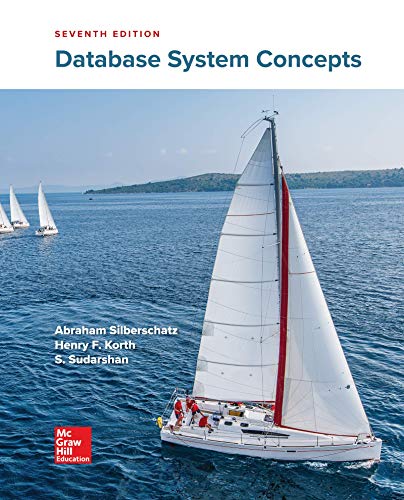
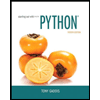
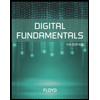
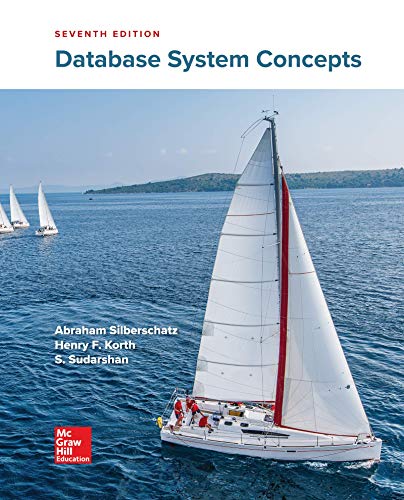
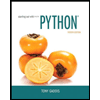
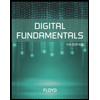
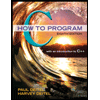
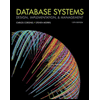
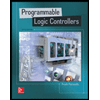