For this exercise, you will be given a project file digital_pet containing two (2) classes, the DigiPet class and the DigiPetTester class. Every DigiPet has five fields: - name - lifespan (in cycles) – max age - age (in cycles) – current age - mood (2 = joyful, 1 = happy, 0 = neutral, -1 = sad, -2 = angry) - size – current size of pet (size > 1) • A DigiPet has a default constructor, overloaded constructor, and some various methods which allow one to interact with it or modify its state. • Poke or pet increases or decreases its mood. • Feed or exercise increases or decreases its size by a specified amount. Every time you interact with the digipet (poke, pet, feed, exercise), its age increases. When the age reaches the lifespan, it dies (or becomes a zombie). You need to modify and customize the DigiPet class as follows: - Implement the overloaded constructor: Initialize all five fields. Use the new values (passed as parameters) along with the same default values that the default constructor uses. - Implement poke(): Decrement mood by 1. Check and make sure mood stays larger than or equal to -2. If it is less than -2, set mood to -2. Call the makeOlder() method to make the pet older by one cycle. (Note that the makeOlder() method is already implemented for you; you simply need to call it.) - Implement pet): Increment mood by 1. Check and make sure mood stays smaller than or equal to 2. If it is larger than 2, set mood to 2. Call the makeOlder() method to make the pet older by one cycle. - Implement feed(int amount): Increases the pet's size by the amount (passed as a parameter to the method). Call the makeOlder() method to make the pet older by one cycle. - Implement exercise(int amount): Decreases the pet's size by the amount. If the resulting size is smaller than 1, print a message "No more energy!" and set the size to 1. Call the makeOlder() method to make the pet older by one cycle. - Modify showPet() (optinal): Customize it any way you like but with the following requirements: It must show the name, age, and lifespan. It must depict the size and mood in some way (be creative).
For this exercise, you will be given a project file digital_pet containing two (2) classes, the DigiPet class and the DigiPetTester class. Every DigiPet has five fields: - name - lifespan (in cycles) – max age - age (in cycles) – current age - mood (2 = joyful, 1 = happy, 0 = neutral, -1 = sad, -2 = angry) - size – current size of pet (size > 1) • A DigiPet has a default constructor, overloaded constructor, and some various methods which allow one to interact with it or modify its state. • Poke or pet increases or decreases its mood. • Feed or exercise increases or decreases its size by a specified amount. Every time you interact with the digipet (poke, pet, feed, exercise), its age increases. When the age reaches the lifespan, it dies (or becomes a zombie). You need to modify and customize the DigiPet class as follows: - Implement the overloaded constructor: Initialize all five fields. Use the new values (passed as parameters) along with the same default values that the default constructor uses. - Implement poke(): Decrement mood by 1. Check and make sure mood stays larger than or equal to -2. If it is less than -2, set mood to -2. Call the makeOlder() method to make the pet older by one cycle. (Note that the makeOlder() method is already implemented for you; you simply need to call it.) - Implement pet): Increment mood by 1. Check and make sure mood stays smaller than or equal to 2. If it is larger than 2, set mood to 2. Call the makeOlder() method to make the pet older by one cycle. - Implement feed(int amount): Increases the pet's size by the amount (passed as a parameter to the method). Call the makeOlder() method to make the pet older by one cycle. - Implement exercise(int amount): Decreases the pet's size by the amount. If the resulting size is smaller than 1, print a message "No more energy!" and set the size to 1. Call the makeOlder() method to make the pet older by one cycle. - Modify showPet() (optinal): Customize it any way you like but with the following requirements: It must show the name, age, and lifespan. It must depict the size and mood in some way (be creative).
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
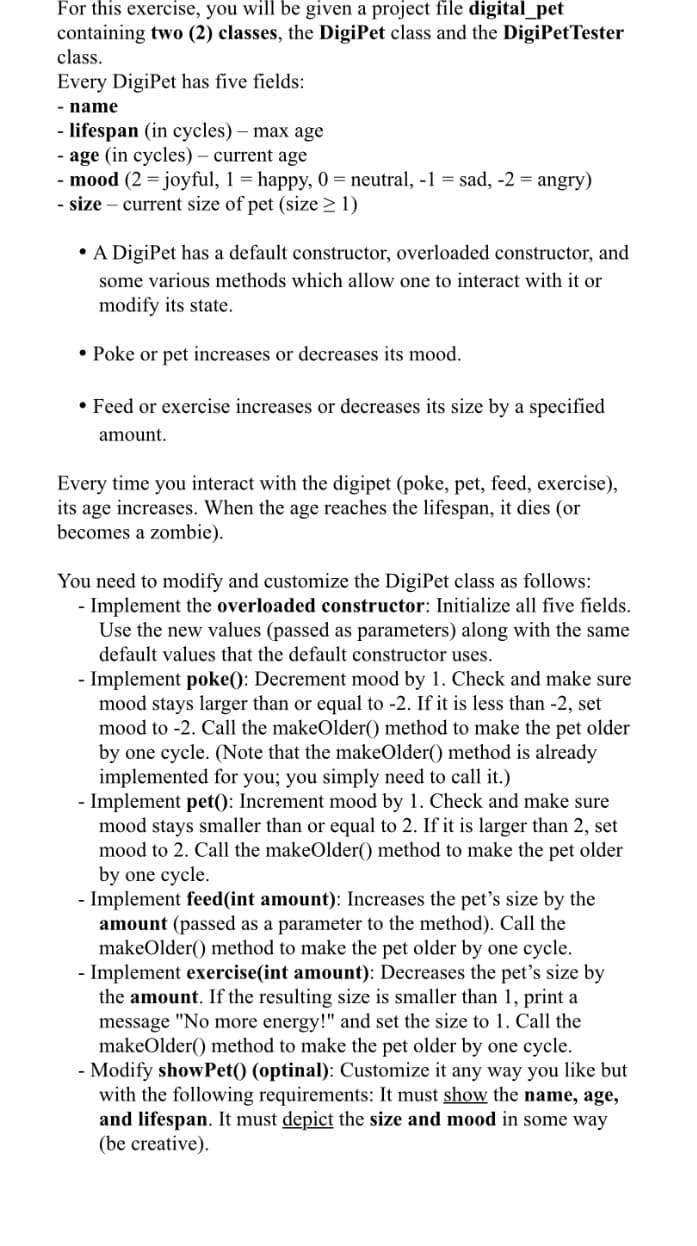
Transcribed Image Text:For this exercise, you will be given a project file digital_pet
containing two (2) classes, the DigiPet class and the DigiPetTester
class.
Every DigiPet has five fields:
- name
- lifespan (in cycles) – max age
- age (in cycles) – current age
- mood (2 = joyful, 1 = happy, 0 = neutral, -1 = sad, -2 = angry)
- size – current size of pet (size > 1)
• A DigiPet has a default constructor, overloaded constructor, and
some various methods which allow one to interact with it or
modify its state.
• Poke or pet increases or decreases its mood.
• Feed or exercise increases or decreases its size by a specified
amount.
Every time you interact with the digipet (poke, pet, feed, exercise),
its age increases. When the age reaches the lifespan, it dies (or
becomes a zombie).
You need to modify and customize the DigiPet class as follows:
- Implement the overloaded constructor: Initialize all five fields.
Use the new values (passed as parameters) along with the same
default values that the default constructor uses.
- Implement poke(): Decrement mood by 1. Check and make sure
mood stays larger than or equal to -2. If it is less than -2, set
mood to -2. Call the makeOlder() method to make the pet older
by one cycle. (Note that the makeOlder() method is already
implemented for you; you simply need to call it.)
- Implement pet): Increment mood by 1. Check and make sure
mood stays smaller than or equal to 2. If it is larger than 2, set
mood to 2. Call the makeOlder() method to make the pet older
by one cycle.
- Implement feed(int amount): Increases the pet's size by the
amount (passed as a parameter to the method). Call the
makeOlder() method to make the pet older by one cycle.
- Implement exercise(int amount): Decreases the pet's size by
the amount. If the resulting size is smaller than 1, print a
message "No more energy!" and set the size to 1. Call the
makeOlder() method to make the pet older by one cycle.
- Modify showPet() (optinal): Customize it any way you like but
with the following requirements: It must show the name, age,
and lifespan. It must depict the size and mood in some way
(be creative).

Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
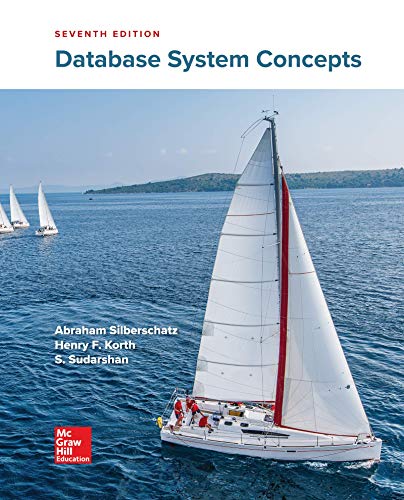
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
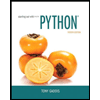
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
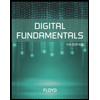
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
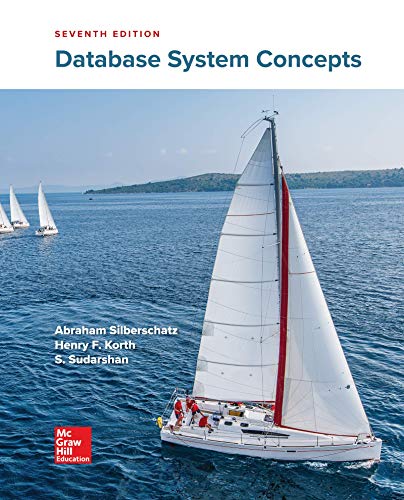
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
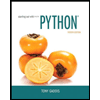
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
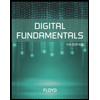
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
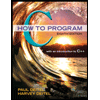
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
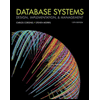
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
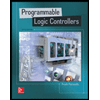
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education