We have a main class that implements a parking charge class of a parking management system. We wanted to generate a junit test class for our main class. Generate a junit test class for our main class ParkingCharge class class Instant{ public int hours; public int minutes; } public class ParkingCharge{ public String permitID; public String lotID; public double amount; public Instant incurredTime = new Instant(); // toString class definition @Override public String toString(){ String permitDetails = "\nPermit ID: "+permitID; String lotDetails = "\nLot ID: "+lotID; String incurredDetails="\nDuration: "+incurredTime.hours+" HH "+incurredTime.minutes+" MM"; // lets say 1 minutes of parking charge is 1 dollar amount = incurredTime.hours*60 + incurredTime.minutes; String amountDetails = "\nParking Charge: "+amount; return permitDetails+lotDetails+incurredDetails+amountDetails; } } Main Class import java.util.Scanner; public class main { public static void main(String [] args){ Scanner sc = new Scanner(System.in); // declared an object of ParkingCharge class ParkingCharge pc = new ParkingCharge(); // taking input for all the fields System.out.print("Enter Permit ID: "); pc.permitID = sc.next(); System.out.print("Enter Lot ID: "); pc.lotID = sc.next(); System.out.print("Enter duration for which vechile is parked: "); System.out.print("Enter hours: "); pc.incurredTime.hours = sc.nextInt(); System.out.print("Enter minutes: "); pc.incurredTime.minutes = sc.nextInt(); // call to toString function to print the details System.out.println(pc.toString()); } } Use the class diagram for reference
We have a main class that implements a parking charge class of a parking management system. We wanted to generate a junit test class for our main class.
Generate a junit test class for our main class
ParkingCharge class
class Instant{
public int hours;
public int minutes;
}
public class ParkingCharge{
public String permitID;
public String lotID;
public double amount;
public Instant incurredTime = new Instant();
// toString class definition
@Override
public String toString(){
String permitDetails = "\nPermit ID: "+permitID;
String lotDetails = "\nLot ID: "+lotID;
String incurredDetails="\nDuration: "+incurredTime.hours+" HH "+incurredTime.minutes+" MM";
// lets say 1 minutes of parking charge is 1 dollar
amount = incurredTime.hours*60 + incurredTime.minutes;
String amountDetails = "\nParking Charge: "+amount;
return permitDetails+lotDetails+incurredDetails+amountDetails;
}
}
Main Class
import java.util.Scanner;
public class main {
public static void main(String [] args){
Scanner sc = new Scanner(System.in);
// declared an object of ParkingCharge class
ParkingCharge pc = new ParkingCharge();
// taking input for all the fields
System.out.print("Enter Permit ID: ");
pc.permitID = sc.next();
System.out.print("Enter Lot ID: ");
pc.lotID = sc.next();
System.out.print("Enter duration for which vechile is parked: ");
System.out.print("Enter hours: ");
pc.incurredTime.hours = sc.nextInt();
System.out.print("Enter minutes: ");
pc.incurredTime.minutes = sc.nextInt();
// call to toString function to print the details
System.out.println(pc.toString());
}
}
Use the class diagram for reference
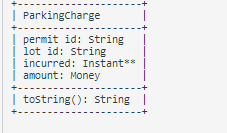

Step by step
Solved in 2 steps with 1 images

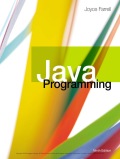
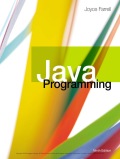