From time to time Yetis who live high up in the Himalayan mountain ranges come down from the mountains to deliver a profound message, in their own language, to the folks in the plains. The folks in the plains look forward to listening to these profound messages. The Yetis' language is called Yetian where the characters, as we know, are represented by 3 digits which cannot be understood by the folks in the plains. Hence, Yetis' come with a translator so that everyone listening to the message can understand it. This time around, Yowie the Yeti came down to deliver the message, but forgot to bring the translator. Mr. Coder in the plains has asked you to help code a translator based on the following rules that he gathered from his previous experiences with the Yetis. Yeti messages always contain three digits for each character, unless a mistake has been made. Hence, the length of a valid Yeti message is always a multiple of three. Each set of three digits translates directly to an English character using the chr() function which translates a decimal number to it's ASCII character equivalent (see the partial ASCII code table below for some codes and their characters). Yeti messages transform to all lowercase English alphabet characters, except for where Yetis intend to have a space between words. A space is represented using the decimal ASCII character code for "Y", i.e., 089, rather than the normal code 032. Hence, all Yeti 3-digit characters fall in the range 097 to 122, or 089 as a space. Write a Python program, in a file called yetian_decoder.py, which asks for a string of digits (the Yetis' message) and converts the message into English by building the message, one character at a time, using the following functions: function isValidYeti that, given the message as a string, returns True if the number of digits in the message is a multiple of three, otherwise it returns False function decodeDigits that, given a 3-character code string, returns the decoded string as the equivalent ASCII character; note that if the character ends up being an uppercase "Y", a space should be returned instead function decodeMsg which, given the complete string of digits, uses repetition to extract three digits at a time, calls the function decodeDigits and, character by character, builds and eventually returns the decoded message function main that allows the user to enter any message as a string of digits and, using the functions mentioned above, checks to see if it is a valid string and, if so, decodes the message and prints the original encoded message, along with the decoded message; if the message is not a valid Yeti string then a message is printed indicating this fact Sample input/output from run 1: Enter the Yeti message: 098101089104097112112121 Yeti Message: 098101089104097112112121 English Translation: be happy Sample input/output from run 2: Enter the Yeti message: 115104111119089107105110100110101115115 Yeti Message: 115104111119089107105110100110101115115 English Translation: show kindness Sample input/output from run 3: Enter the Yeti message: 1081010971141100891021141111090891011141141111141150 The string is not a valid Yeti message. Partial ASCII Character Code Table Dec Chr Dec Chr Dec Chr Dec Chr Dec Chr Dec Chr 48'0' 49'1' 50'2' 54'6' 55'7' 56'8' 60'<' 61'=' 62'>' 66'B' 67'C' 68'D' 72'H' 73'I' 74'J' 78'N' 79'O' 80'P' 84'T' 85'U' 86'V' 90'Z' 91'[' 92'\' 96'`' 97'a' 98'b' 51'3' 52'4' 57'9' 58':' 63'?' 64'@' 69'E' 70'F' 75'K' 76'L' 81'Q' 82'R' 87'W' 88'X' 93']' 94'^' 99'c'100'd'101'e' 102 'f' 103 'g' 104 'h' 105 'i' 106 'j' 107 'k' 108 'l' 109 'm' 110 'n' 111 'o' 112 'p' 113 'q' 114 'r' 115 's' 116 't' 117 'u' 118 'v' 119 'w' 120 'x' 121 'y' 122 'z' Note: In your code you should use only the user-defined functions (as described above), along with loops, decision structures and the built-in functions input(), range(), len(), int(),chr() and print().
THE FOLLOWING PROGRAM MUST BE DONE IN PYTHON.
From time to time Yetis who live high up in the Himalayan mountain ranges come down from the mountains to deliver a profound message, in their own language, to the folks in the plains. The folks in the plains look forward to listening to these profound messages. The Yetis' language is called Yetian where the characters, as we know, are represented by 3 digits which cannot be understood by the folks in the plains. Hence, Yetis' come with a translator so that everyone listening to the message can understand it. This time around, Yowie the Yeti came down to deliver the message, but forgot to bring the translator. Mr. Coder in the plains has asked you to help code a translator based on the following rules that he gathered from his previous experiences with the Yetis.
-
Yeti messages always contain three digits for each character, unless a mistake has been made. Hence, the length of a valid Yeti message is always a multiple of three.
-
Each set of three digits translates directly to an English character using the chr() function which translates a decimal number to it's ASCII character equivalent (see the partial ASCII code table below for some codes and their characters).
-
Yeti messages transform to all lowercase English alphabet characters, except for where Yetis intend to have a space between words. A space is represented using the decimal ASCII character code for "Y", i.e., 089, rather than the normal code 032. Hence, all Yeti 3-digit characters fall in the range 097 to 122, or 089 as a space.
Write a Python program, in a file called yetian_decoder.py, which asks for a string of digits (the Yetis' message) and converts the message into English by building the message, one character at a time, using the following functions:
-
function isValidYeti that, given the message as a string, returns True if the number of digits in the
message is a multiple of three, otherwise it returns False
-
function decodeDigits that, given a 3-character code string, returns the decoded string as the equivalent
ASCII character; note that if the character ends up being an uppercase "Y", a space should be returned
instead
-
function decodeMsg which, given the complete string of digits, uses repetition to extract three digits at a
time, calls the function decodeDigits and, character by character, builds and eventually returns the
decoded message
-
function main that allows the user to enter any message as a string of digits and, using the functions
mentioned above, checks to see if it is a valid string and, if so, decodes the message and prints the original encoded message, along with the decoded message; if the message is not a valid Yeti string then a message is printed indicating this fact
Sample input/output from run 1:
Enter the Yeti message: 098101089104097112112121 Yeti Message: 098101089104097112112121 English Translation: be happy
Sample input/output from run 2:
Enter the Yeti message: 115104111119089107105110100110101115115 Yeti Message: 115104111119089107105110100110101115115 English Translation: show kindnessSample input/output from run 3:
Enter the Yeti message: 1081010971141100891021141111090891011141141111141150 The string is not a valid Yeti message.Partial ASCII Character Code Table
Dec Chr Dec Chr Dec Chr Dec Chr Dec Chr Dec Chr48'0' 49'1' 50'2' 54'6' 55'7' 56'8' 60'<' 61'=' 62'>' 66'B' 67'C' 68'D' 72'H' 73'I' 74'J' 78'N' 79'O' 80'P' 84'T' 85'U' 86'V' 90'Z' 91'[' 92'\' 96'`' 97'a' 98'b'
51'3' 52'4'
57'9' 58':'
63'?' 64'@'
69'E' 70'F'
75'K' 76'L'
81'Q' 82'R'
87'W' 88'X'
93']' 94'^' 99'c'100'd'101'e'
Note: In your code you should use only the user-defined functions (as described above), along with loops, decision structures and the built-in functions input(), range(), len(), int(),chr() and print().

Step by step
Solved in 4 steps with 6 images

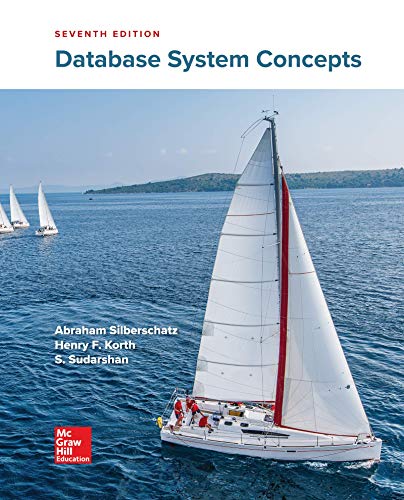
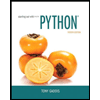
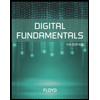
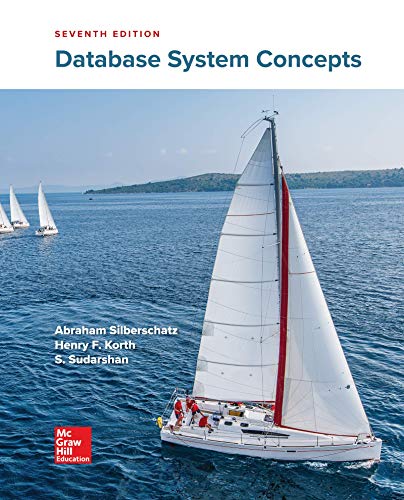
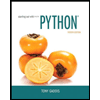
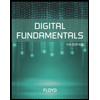
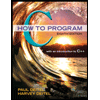
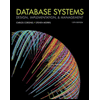
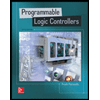