Hi in the below code I would like to remove the blank tokens and how to achieve it. #include #include #include int count_tokens(const char* str) { int count = 0; int invalidToken = 0; // set false for(int i = 0; i <= strlen(str); i++) { if( i == strlen(str) || str[i] == 32){ // if past end of string, or if space if(invalidToken) { invalidToken = 0; // set false continue; } else { count++; // increment count only if token was not invalid continue; } } if( !(str[i] >= 97 && str[i] <= 122) ) { // if not lowercase letter invalidToken = 1; // set true } } return count; } char** split_tokens(const char* str) { char **tokenArr; tokenArr = (char **) malloc(count_tokens(str) * sizeof(char*)); /*use count_tokes() to determine the length*/ int invalidToken = 0; // set false int startIndex = 0; int arrayIndex = 0; for(int i = 0; i <= strlen(str); i++) { if( i == strlen(str) || str[i] == 32){ // if past end of string, or if space if(invalidToken) { invalidToken = 0; // set false startIndex = i + 1; continue; } else { int lengthOfTokenFound = i - startIndex; char *tokenFound; tokenFound = (char*) malloc((lengthOfTokenFound+1)*sizeof(char)); /*+1 for '\0' character */ for(int i = 0; i < lengthOfTokenFound; i++) { tokenFound[i] = str[startIndex+i]; } tokenArr[arrayIndex] = tokenFound; arrayIndex++; startIndex = i + 1; continue; } } if( !(str[i] >= 97 && str[i] <= 122) ) { // if not lowercase letter invalidToken = 1; // set true } } return tokenArr; } void printArrayOfStrings(char ** arr, int length) { printf("["); for(int i = 0; i < length; i++) { printf("'%s'", arr[i]); if(i < length-1) { printf(", "); } } printf("]\n\n"); } void main() { char myStr1[] = "abc EFaG hi"; int count1 = count_tokens(myStr1); printf("String '%s' has %d tokens.\n",myStr1, count1); char** tokArr1 = split_tokens(myStr1); printArrayOfStrings(tokArr1, count1); char myStr2[] = "ab 12 ef hi"; int count2 = count_tokens(myStr2); printf("String '%s' has %d tokens.\n",myStr2, count2); char** tokArr2 = split_tokens(myStr2); printArrayOfStrings(tokArr2, count2); char myStr3[] = "ab12ef+"; int count3 = count_tokens(myStr3); printf("String '%s' has %d tokens.\n",myStr3, count3); char** tokArr3 = split_tokens(myStr3); printArrayOfStrings(tokArr3, count3); }
Hi in the below code I would like to remove the blank tokens and how to achieve it. #include #include #include int count_tokens(const char* str) { int count = 0; int invalidToken = 0; // set false for(int i = 0; i <= strlen(str); i++) { if( i == strlen(str) || str[i] == 32){ // if past end of string, or if space if(invalidToken) { invalidToken = 0; // set false continue; } else { count++; // increment count only if token was not invalid continue; } } if( !(str[i] >= 97 && str[i] <= 122) ) { // if not lowercase letter invalidToken = 1; // set true } } return count; } char** split_tokens(const char* str) { char **tokenArr; tokenArr = (char **) malloc(count_tokens(str) * sizeof(char*)); /*use count_tokes() to determine the length*/ int invalidToken = 0; // set false int startIndex = 0; int arrayIndex = 0; for(int i = 0; i <= strlen(str); i++) { if( i == strlen(str) || str[i] == 32){ // if past end of string, or if space if(invalidToken) { invalidToken = 0; // set false startIndex = i + 1; continue; } else { int lengthOfTokenFound = i - startIndex; char *tokenFound; tokenFound = (char*) malloc((lengthOfTokenFound+1)*sizeof(char)); /*+1 for '\0' character */ for(int i = 0; i < lengthOfTokenFound; i++) { tokenFound[i] = str[startIndex+i]; } tokenArr[arrayIndex] = tokenFound; arrayIndex++; startIndex = i + 1; continue; } } if( !(str[i] >= 97 && str[i] <= 122) ) { // if not lowercase letter invalidToken = 1; // set true } } return tokenArr; } void printArrayOfStrings(char ** arr, int length) { printf("["); for(int i = 0; i < length; i++) { printf("'%s'", arr[i]); if(i < length-1) { printf(", "); } } printf("]\n\n"); } void main() { char myStr1[] = "abc EFaG hi"; int count1 = count_tokens(myStr1); printf("String '%s' has %d tokens.\n",myStr1, count1); char** tokArr1 = split_tokens(myStr1); printArrayOfStrings(tokArr1, count1); char myStr2[] = "ab 12 ef hi"; int count2 = count_tokens(myStr2); printf("String '%s' has %d tokens.\n",myStr2, count2); char** tokArr2 = split_tokens(myStr2); printArrayOfStrings(tokArr2, count2); char myStr3[] = "ab12ef+"; int count3 = count_tokens(myStr3); printf("String '%s' has %d tokens.\n",myStr3, count3); char** tokArr3 = split_tokens(myStr3); printArrayOfStrings(tokArr3, count3); }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Hi in the below code I would like to remove the blank tokens and how to achieve it.
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int count_tokens(const char* str) {
int count = 0;
int invalidToken = 0; // set false
for(int i = 0; i <= strlen(str); i++) {
if( i == strlen(str) || str[i] == 32){ // if past end of string, or if space
if(invalidToken) {
invalidToken = 0; // set false
continue;
}
else {
count++; // increment count only if token was not invalid
continue;
}
}
if( !(str[i] >= 97 && str[i] <= 122) ) { // if not lowercase letter
invalidToken = 1; // set true
}
}
return count;
}
char** split_tokens(const char* str) {
char **tokenArr;
tokenArr = (char **) malloc(count_tokens(str) * sizeof(char*)); /*use count_tokes() to determine the length*/
int invalidToken = 0; // set false
int startIndex = 0;
int arrayIndex = 0;
for(int i = 0; i <= strlen(str); i++) {
if( i == strlen(str) || str[i] == 32){ // if past end of string, or if space
if(invalidToken) {
invalidToken = 0; // set false
startIndex = i + 1;
continue;
}
else {
int lengthOfTokenFound = i - startIndex;
char *tokenFound;
tokenFound = (char*) malloc((lengthOfTokenFound+1)*sizeof(char)); /*+1 for '\0' character */
for(int i = 0; i < lengthOfTokenFound; i++) {
tokenFound[i] = str[startIndex+i];
}
tokenArr[arrayIndex] = tokenFound;
arrayIndex++;
startIndex = i + 1;
continue;
}
}
if( !(str[i] >= 97 && str[i] <= 122) ) { // if not lowercase letter
invalidToken = 1; // set true
}
}
return tokenArr;
}
void printArrayOfStrings(char ** arr, int length) {
printf("[");
for(int i = 0; i < length; i++) {
printf("'%s'", arr[i]);
if(i < length-1) {
printf(", ");
}
}
printf("]\n\n");
}
void main() {
char myStr1[] = "abc EFaG hi";
int count1 = count_tokens(myStr1);
printf("String '%s' has %d tokens.\n",myStr1, count1);
char** tokArr1 = split_tokens(myStr1);
printArrayOfStrings(tokArr1, count1);
char myStr2[] = "ab 12 ef hi";
int count2 = count_tokens(myStr2);
printf("String '%s' has %d tokens.\n",myStr2, count2);
char** tokArr2 = split_tokens(myStr2);
printArrayOfStrings(tokArr2, count2);
char myStr3[] = "ab12ef+";
int count3 = count_tokens(myStr3);
printf("String '%s' has %d tokens.\n",myStr3, count3);
char** tokArr3 = split_tokens(myStr3);
printArrayOfStrings(tokArr3, count3);
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 6 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
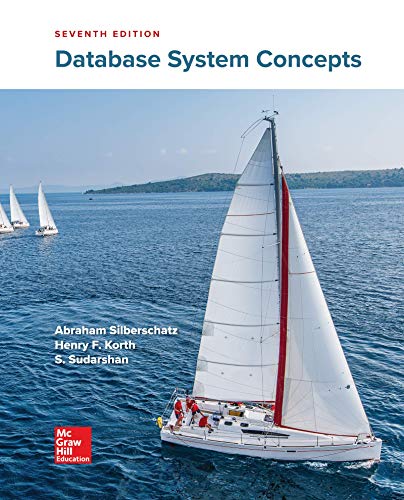
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
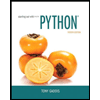
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
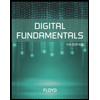
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
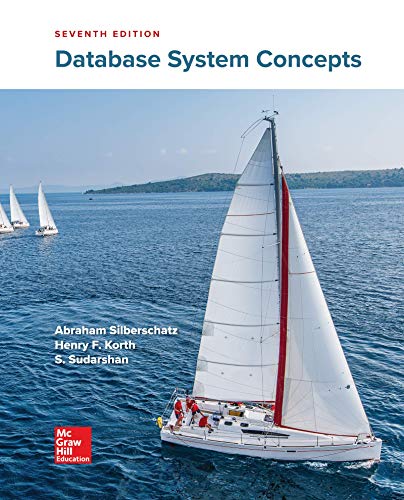
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
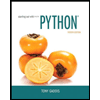
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
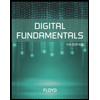
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
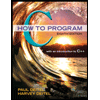
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
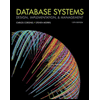
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
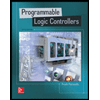
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education