/** * Generate all combinations of the characters in the string s with length * k. * * @param * a string. * @param k * the length of the combinations * @return a list of all of the combinations of the strings in s. */ public static ArrayList combinations (String s, int k) { return combinations ("", s, k); }| /** * Recursive Problem Transformation: * * Generate all combinations of length k of the characters in rest prefixed * with the characters in prefix. This is very similar to the subset method! *Note k is the total length of each string in the returned ArrayList. * * * For example: * combinations ("", "ABC", 2) -> "" before {AB, AC, BC} * -> {AB, AC, BC} * * * * * * combinations ("A", "BC", 2) -> A before {BC, B, C, ""} -> {AB, AC} combinations ("", "BC", 2) -> "" before {BC, B, C, ""} -> {BC}
/** * Generate all combinations of the characters in the string s with length * k. * * @param * a string. * @param k * the length of the combinations * @return a list of all of the combinations of the strings in s. */ public static ArrayList combinations (String s, int k) { return combinations ("", s, k); }| /** * Recursive Problem Transformation: * * Generate all combinations of length k of the characters in rest prefixed * with the characters in prefix. This is very similar to the subset method! *Note k is the total length of each string in the returned ArrayList. * * * For example: * combinations ("", "ABC", 2) -> "" before {AB, AC, BC} * -> {AB, AC, BC} * * * * * * combinations ("A", "BC", 2) -> A before {BC, B, C, ""} -> {AB, AC} combinations ("", "BC", 2) -> "" before {BC, B, C, ""} -> {BC}
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
Complete the below function (using recursion)
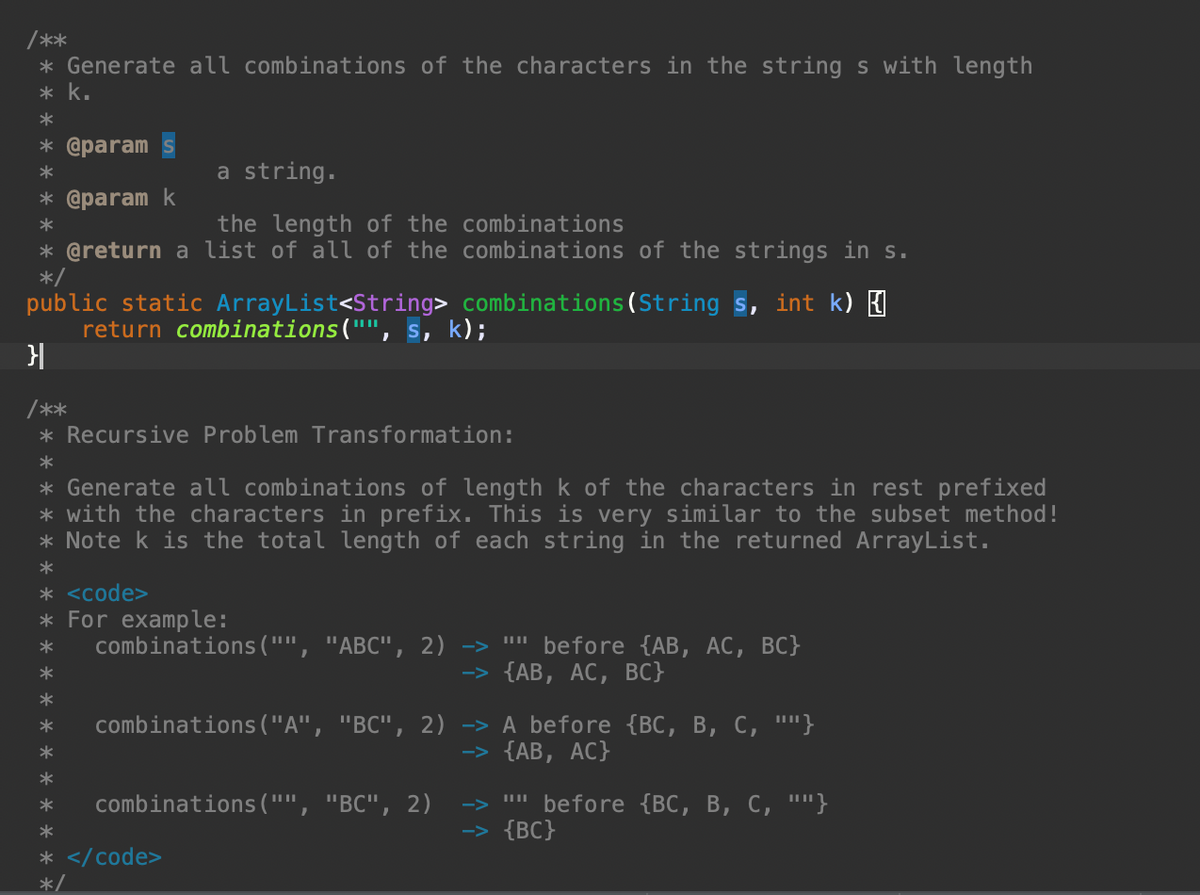
Transcribed Image Text:/**
* Generate all combinations of the characters in the string s with length
* k.
*
* @param
*
a string.
the length of the combinations
* @return a list of all of the combinations of the strings in s.
*/
public static ArrayList<String> combinations (String s, int k) {
return combinations("", s, k);
}|
/**
* @param k
*
Recursive Problem Transformation:
*
* Generate all combinations of length k of the characters in rest prefixed
* with the characters in prefix. This is very similar to the subset method!
*Note k is the total length of each string in the returned ArrayList.
*
* <code>
* For example:
*
combinations ("", "ABC", 2) -> "" before {AB, AC, BC}
*
-> {AB, AC, BC}
*
*
combinations ("A", "BC", 2)
combinations ("", "BC", 2)
*
*
* </code>
*/
-> A before {BC, B, C, ""}
-> {AB, AC}
before {BC, B, C, ""}
-> {BC}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
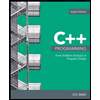
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
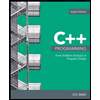
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning