Write a for loop to initialize the objects in the ArrayList created in question number 2 above to -1. import java.util.*; public class test { public static void main(String[] args) { int n = 25; ArrayList list = new ArrayList(n); for(int i = 0;i
Q: Write a Java program that goes through Collection of Linked List elements of String data type and…
A: Code is working and screenshot of working code is also attached
Q: Would a LinkedList or an ArrayList perform better when run on the following code? Why? public static…
A: In Linked List, each node can be present at different memory location but is linked to each other…
Q: Write a method called removeDuplicates() that takes as a parameter a sorted ArrayList of Strings and…
A: Required: Write a method called removeDuplicates() that takes as a parameter a sorted ArrayList of…
Q: Trying this again since part of my question keeps disappearing Given main() in the ShoppingList…
A: I have Provided this answer with full description in step-2.
Q: PLEASE SOLVE IN JAVA. I can only fit the entire problem by having the driver code as an image but…
A: Program: public class DoublyLinkedList<E> { // define ListNode elements specific for this…
Q: Implement a List using array: a. Implement ArrayList class of ListADT interface which will define…
A: The answer given as below:
Q: Suppose myGoods is an ArrayList of Product objects. Write the code using an iterator and a while…
A: // Java code to illustrate iterator() import java.util.*; public class Main { public static void…
Q: Add a method, printEvenNodes, to the SinglyLinkedList class that prints all integers stored in the…
A: Note: Answering the question in python as no language is mentioned. Task : Given the singly linked…
Q: Write a program in Java using ArrayList to remove duplicates in the array {0,1,4,5,8,0,9,4}.
A:
Q: Consider the List interface's add (i, x) and remove (i) methods. When i is big (say n-2 or n-1),…
A: Ans:- option 3
Q: Write the implementation of a static method, called sumOdds, that belongs IntNode. The method takes…
A: Task :- Write a Java program to find the sum of all odd numbers in the list. Java program :-…
Q: Write a method which takes as input a singly linked list and print the first duplicate number.…
A: import java.util.*;public class Duplicate{static class Node{int data;Node next;};static Node…
Q: If an array of size 6 is used to implement a circular queue, and the current values of rear and…
A:
Q: In java, given: // Returns the element at the specified position in this list. public E get(int…
A: We need to write a method to replace the value at given index in the list.
Q: Apply the methods in the ListIterator interface to write a Java program in NetBeans that creates a…
A: The program should then print out the elements initially in the original order, and then afterwards…
Q: Remember, the well-balanced response you have to the question you respond must be well-spoken and…
A: Please find the answer to the above question below:
Q: Implement the following two methods in O(n) time. // Reverse the list and return it in O(n) time…
A: I have implemented both the methods for you with proper code and comments and Main method , class…
Q: Write a static method named negListFromQueue that takes two parameters: an array-based unbounded…
A: Given that, Write a static method named negListFromQueue that takes two parameters: an array-based…
Q: Provide a static method that reverses the elements of a generic array list, without modifying the…
A: import java.util.*;class ArrayMethods{ private int[] values; //constructor to initialize values…
Q: Given the following methods from the List interface draw the final state of an initial empty…
A: Initial list is []*AS.add(s) will add the String at the end of the list*AS.add(i,s) will insert…
Q: Make the following class generic so that it can deal with an arbitrary class rather than only Dog…
A: Given: Make the following class generic so that it can deal with an arbitrary class rather than…
Q: In Java: Modify the attached program code below According to the question a, b and c a. Replace the…
A: import java.util.Scanner;class DoublyLinkedList { private Node head;private Node tail;private int…
Q: Assume we have a linked list of integer elements. Write a recursive method recNumEven (LLNode list)…
A: PROGRAM STRUCTURE: Write the definition of the function to find the number of even values in the…
Q: f) Write Java code and illustrates the elements of myList after removing element 38 at index 2.…
A: The question has been answered in step2
Q: Consider the following Java code: count = 0; for (int i = 0; i < list.size(); i++) {…
A: We are going to find out the worst case time complexity for the given java code. In worst case, how…
Q: Write a method to sort an array of elements at index in a single linked list and then display this…
A: public class Main{ node head; node sorted; class node { int val; node next; public node(int…
Q: Apply the methods in the ListIterator interface to write a Java program in NetBeans that creates a…
A: Push the inputs in the linked list. Then print the list. And then reverse the list. And again print…
Q: Write a static method named SmallestEvenStackQueueToList that takes two parameters: an array-based…
A: Given:
Q: Given a stack of positive integers, write a Java method called movePrimes that rearranges elements…
A: Algorithm: Start Define 2 stacks stack, stack1 Store the given data in the 1st stack i.e stack Now…
Q: Write a method manyStrings that takes an ArrayList of Strings and an integer n as parameters and…
A: public static void manyStrings(ArrayList<String> list, int n) { if(n > 1) { for(int i =…
Q: using arrays or Arraylist in Java language Write the method filterBySize(). * * Given an ArrayList…
A: CODE: //Driver code public class Main { public static void main(String[] args) { String []arr= {"a",…
Q: · Write a method to insert an element at index in a Doubly Linked List data structure and test it.…
A: Solution: Doubly Linked List:
Q: We define the stretch of a value in a list to be the number of elements between that two leftmost…
A: Here is the approcach to solve the question :- Take the arraylist and then apply the for loop on…
Q: Exercise 3: Provide a static method that checks whether a generic array list is a palindrome; that…
A: import java.util.*;import java.lang.*;import java.io.*; class Codechef{ public static boolean…
Q: Give me the numbered steps of the method Putltem() for an Array implementation of the Sorted List.…
A: Find the required answer in sentences given as below :
Q: FOR JAVA Consider rewriting the method max() given below such that it gives the correct answer even…
A: Given: FOR JAVA Consider rewriting the method max() given below such that it gives the correct…
Q: Suppose that you want an operation for the ADT list that adds an array of items to the end of the…
A: Given: Suppose that you want an operation for the ADT list that adds an array of items to the end of…
Q: Using classes , write down a program that implements creation of a head pointer of a linked list ,…
A: A CLass is a user defined data type that can be used in our C++ program.
Q: Based on your understanding of how lists are implemented in Java, which of the following Queue…
A: Hey there, I am writing the required solution based on the above given question. Please do find the…
Q: Implement a fill method in AList class to fill all the elements in the list with a given value it.…
A: The program is written in c++ #include <iostream> using namespace std;template <typename…
Q: Implement a lastIndex method for the LinkedIntList class you worked on for HW4. This method takes…
A: Solution: There is no //TODO comment in the code mentioned in comment Hence, you can insert…
Q: Write a method markLength4 that takes an ArrayList of Strings as a parameter and that places a…
A: import java.util.*;class Test113 { public static void main(String args[]) { ArrayList<String>…
Q: Write code in java for bookstore where is object sequentially aggregated, list of book name, year of…
A: Code: import java.util.ArrayList;import java.util.Iterator; class BookStore { String book_name,…
Q: JAVA plese Implement the indexOf method in the LinkedIntegerList class public int indexOf(int…
A: the correct solution is attached below:-
Q: Write a method evensum that returns the sum of the values in even indices in a list of integers.…
A: front is storing the first node address and then through this we can iterate till last node by…
Q: Write a method and test it to insert an array of elements at index in a single linked list and then…
A: Start Take the elements Elements can be positioned at indexes. At particular positions they placed.…
Q: Write a method to insert an element at index in a Doubly Linked List data structure and test it. The…
A: #include <iostream> using namespace std; //structure of the linked list struct node { char…
Q: Given a standard linked list of int, L, write a method to print L in order: 1. using a loop 2. using…
A: PART 1:- CODE:- // Method to print the list using simple looppublic void…
Write a for loop to initialize the objects in the ArrayList created in question number 2 above to -1.

Step by step
Solved in 3 steps with 1 images

- Fix the following method printEvenIndex so that it will print out the Integers at even indices of the passed-in ArrayList list. import java.util.*; public class Test1 { public static void printEvenIndex(ArrayList<Integer> list) { for (int i) { if (i % 2 == 1) { System.out.print(list.get(i) + ", "); } } } public static void main(String[] args) { //instantiate ArrayList and fill with Integers ArrayList<Integer> values = new ArrayList<Integer>(); int[] nums = {1, 5, 7, 9, -2, 3, 2}; for (int i = 0; i < nums.length; i ++) { values.add(nums[i]); } System.out.println("Expected Result:\t 1, 7, -2, 2,"); System.out.print("Your Result:\t\t "); printEvenIndex(values); } }using arrays or Arraylist in Java language Write the method filterBySize().* * Given an ArrayList of String, return a new list where only * strings of the given length are retained.* * Examples:* filterBySize(["a", "bb", "b", "ccc"], 1) returns ["a", "b"]* filterBySize(["a", "bb", "b", "ccc"], 3) returns ["ccc"]* filterBySize(["a", "bb", "b", "ccc"], 4) returns []* * @param list the list of Strings to process.* @param n the size of words to retain.* @return a new list of words with the indicated size retained.In java, Implement a method that removes all duplicates. Keep the first instance of the element but remove all subsequent duplicate elements.public void removeDuplicates() see the picture for the right output. Given files: Demo6.java public class Demo6 { public static void main(String[] args) { MyLinkedList<String> list = new MyLinkedList<>(); TestBench.addToList(list); TestBench.addToList(list); TestBench.addToList(list); TestBench.addToList(list); for (int x = 0; x < list.size(); x += 1 + x/3) { System.out.println("Removing element at index: " + x); list.remove(x); } System.out.println("Result"); System.out.println(list); System.out.println("Shuffling"); list.shuffle(7777); System.out.println(list); System.out.println("Removing duplicates (keeping first instance)"); list.removeDuplicates(); System.out.println(list); }} TestBench.java import java.util.AbstractList; public class TestBench { public static…
- Using JAVA, write a method that modifies an ArrayList<String>, moving all strings starting with an uppercase letter to the front, without otherwise changing the order of the elements. Write a test program to demonstrate the correct operation of the method.Add a void instance method replaceAll(E oldE, E newE) in the MyLinkedList class to replace the occurrence of all oldE value with the specified newE value. Implement this method in O(n) time. import java.util.*; public class PExamCh24 { public static void main(String[] args) { new PExamCh24();}public PExamCh24() { // Create a list for strings MyLinkedList<String> list = new MyLinkedList<>(); // Add elements to the list list.add("America"); // Add America to the list System.out.println("(1) " + list); list.add(0, "Japan"); // Add Canada to the beginning of the list System.out.println("(2) " + list); list.add("Russia"); // Add Russia to the end of the list System.out.println("(3) " + list); list.addLast("Japan"); // Add France to the end of the list System.out.println("(4) " + list); list.add(2, "Japan"); // Add Germany to the list at index 2 System.out.println("(5) " + list); list.add(5, "Norway"); // Add Norway to the list…Using the picture, use Java to design and implement the class PascalTriangle that will generate a Pascal Triangle from a given number of rows. Represent each row in a triangle as a list and the entire triangle as a list of these lists, implement the class ArrayList for these lists.
- Write a program in Java using ArrayList to remove duplicates in the array {0,1,4,5,8,0,9,4}.Write a java while loop that loops through an array of type int with a size of 6, and adds up every other element in the array and prints the sum.Java 1. Implement ArrayUnorderedList<T> class which will extend ArrayList<T> by defining the following additional methods(i) public void addToFront(T element); //Adds the specified element to the front of the list.(ii) public void addToRear(T element); //Adds the specified element to the rear of the list.(iii) public void addAfter(T element, T target); //Adds the specified element after the specified target element.a. Create an object of ArrayUnorderedList<T> class and perform some add, remove, and search operations and finally print the entire Stack.
- a Java method that takes an array of integers as its only parameter and returns the "centered" average of that list as a double. If the smallest or largest value occurs more than once, only keep one copy.import java.util.ArrayList;import java.util.Arrays;public class PS{/*** Write the method named mesh.** Start with two ArrayLists of String, A and B, each with* its elements in alphabetical order and without any duplicates.* Return a new list containing the first N elements from the two* lists. The result list should be in alphabetical order and without* duplicates. A and B will both have a size which is N or more.* Your solution should make a single pass over A and B, taking* advantage of the fact that they are in alphabetical order,* copying elements directly to the new list.** Remember, to see if one String is "greater than" or "less than"* another, you need to use the compareTo() method, not the < or >* operators.** Examples:* mesh(["a","c","z"], ["b","f","z"], 3) returns ["a","b","c"]* mesh(["a","c","z"], ["c","f","z"], 3) returns ["a","c","f"]* mesh(["f","g","z"], ["c","f","g"], 3) returns ["c","f","g"]** @param a an ArrayList of String in alphabetical order.* @param b an…java In this assignment you will swap a position in an array list with another. swap() gets 3 arguments, an Arraylist, a position, and another position to swap with. Example swap(["one","two","three"],0,2) returns:["three","two","one"] public static ArrayList<String> swap(ArrayList<String> list,int pos1,int pos2) public static void main(String[] args) { Scanner in = new Scanner(System.in); int size = in.nextInt(); int pos1 = in.nextInt(); int pos2 = in.nextInt(); ArrayList<String> list = new ArrayList<>(); for(int i=0; i < size; i++) { list.add(in.next()); } System.out.println(swap(list, pos1, pos2)); } }
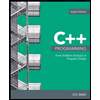
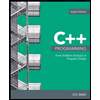