Given a certain temperature in Fahrenheit and a maximum wind velocity (speed) in Miles Per Hour, calculate and display a series of wind chill factors from a wind velocity of 1 MPH to the maximum wind velocity in a table. The user is allowed to specify a maximum wind velocity of up to 50 MPH. Create a project and class in BlueJ named Project2. In main(), your program should prompt the user to enter the current temperature and use a Scanner object to store it in a double variable. Then the user should be prompted to enter the maximum wind velocity and use the Scanner object to store it in an integer variable. If the maximum wind velocity is greater than 1 AND less than or equal to 50, do the following: • Display the first line of the table with column headers for the current temperature, the wind velocity (speed), the calculated wind chill factor, and what the temperature actually feels like. You will need to use the \t escape sequence to put spacing between the columns. • Within a for loop, call a method named wcfactor that has a double parameter and an integer parameter. The method will return a double value for the wind chill factor, based on the following formula (T is the temperature, V is the wind velocity): o ?? = 35.74 + 0.6215 ∗ ?? − 35.75 ∗ ??0.16 + 0.4275 ∗ ?? ∗ ??0.16 • Display each line of the table using a System.out.printf statement. The data should be in this order: current temp, wind speed, calculated wind chill factor, and what the temperature actually feels like. Each line of data must do the following: o The current temp must be converted to an integer using casting so that no decimal places are displayed. o The calculated wind chill factor must be rounded to 2 decimal places. o The Math.round() method must be used to display what the temperature actually feel like with no decimal places. o You must use field widths for each of the four data items to make each data item appear near the middle of each column. You are not allowed to use any escape characters for any of the four data items. Refer to the sample output document in the Files area of Canvas for an example. Otherwise (maximum wind speed is less than or equal to 1 OR greater than 50), display an error message saying “Invalid max wind
Given a certain temperature in Fahrenheit and a maximum wind velocity
(speed) in Miles Per Hour, calculate and display a series of wind chill
factors from a wind velocity of 1 MPH to the maximum wind velocity in a
table. The user is allowed to specify a maximum wind velocity of up to 50
MPH.
Create a project and class in BlueJ named Project2.
In main(), your program should prompt the user to enter the current
temperature and use a Scanner object to store it in a double variable. Then
the user should be prompted to enter the maximum wind velocity and use
the Scanner object to store it in an integer variable.
If the maximum wind velocity is greater than 1 AND less than or equal to
50, do the following:
• Display the first line of the table with column headers for the current
temperature, the wind velocity (speed), the calculated wind chill
factor, and what the temperature actually feels like. You will need to
use the \t escape sequence to put spacing between the columns.
• Within a for loop, call a method named wcfactor that has a double
parameter and an integer parameter. The method will return a double
value for the wind chill factor, based on the following formula (T is the
temperature, V is the wind velocity):
o ?? = 35.74 + 0.6215 ∗ ?? − 35.75 ∗ ??0.16 + 0.4275 ∗ ?? ∗ ??0.16
• Display each line of the table using a System.out.printf statement.
The data should be in this order: current temp, wind speed,
calculated wind chill factor, and what the temperature actually feels
like. Each line of data must do the following:
o The current temp must be converted to an integer using casting
so that no decimal places are displayed.
o The calculated wind chill factor must be rounded to 2 decimal
places.
o The Math.round() method must be used to display what the
temperature actually feel like with no decimal places.
o You must use field widths for each of the four data items to
make each data item appear near the middle of each column.
You are not allowed to use any escape characters for any
of the four data items. Refer to the sample output document in
the Files area of Canvas for an example.
Otherwise (maximum wind speed is less than or equal to 1 OR greater than
50), display an error message saying “Invalid max wind speed”.
You must have the code header contained in the
CS21CommentHeader.docx file at the beginning of your code. Please fill
out the header with the proper information and remove all text in
parentheses.
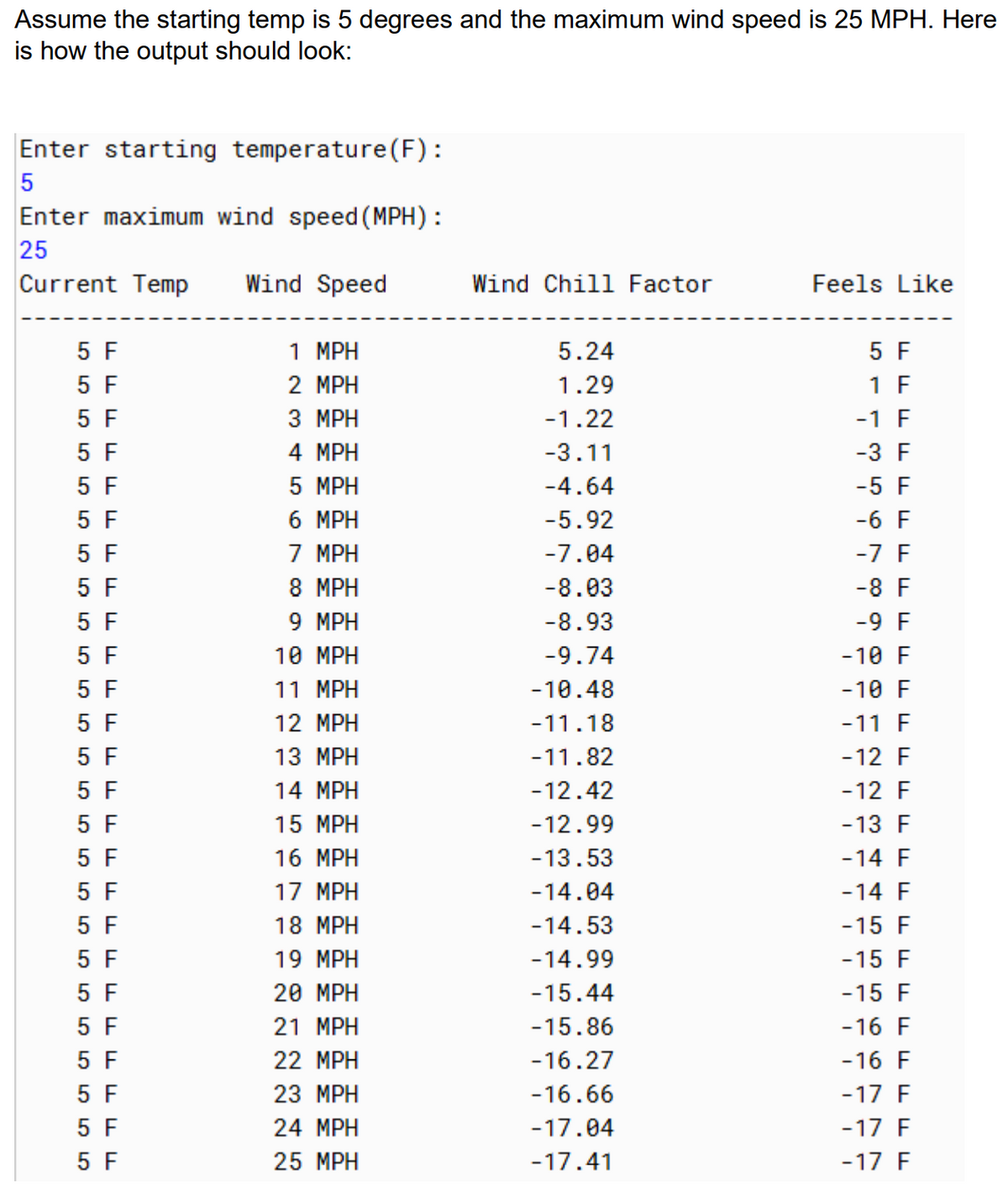

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images
