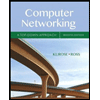
Given a pointer to the head of a linked list and a specific position, determine the data value at that position. Count backwards from the tail node. The tail is at position 0, its parent is at 1 and so on.
Example
head refers to 3 -> 2 -> 1 -> 0 -> NULL
positionFromTail = 2
Each of the data values matches its distance from the tail. The value 2 is at the desired position.
Function Description
Complete the getNode function in the editor below.
getNode has the following parameters:
· SinglyLinkedListNode pointer head: refers to the head of the list
· int positionFromTail: the item to retrieve
Returns
· int: the value at the desired position
Input Format
The first line contains an integer t, the number of test cases.
Each test case has the following format:
The first line contains an integer n, the number of elements in the linked list.
The next n lines contains an integer, the data value for an element of the linked list.
The last line contains an integer positionFromTail, the position from the tail to retrieve the
value of.
Constraints
· 1 ≤ t ≤ 10
· 1 ≤ n, m ≤ 1000
· 1 ≤ list1[i] ≤ 1000, where list[i] is the ith element of the linked list.
· 0 ≤ positionFromTail < n
Sample Input
2
1
1
0
3
3
2
1
2
Sample Output
1
3
Explanation
In the first case, there is one element in linked list with a value of 1. The last (only) element contains 1.
In the second case, the list is 3 -> 2 -> 1 -> NULL. The element with position of 2 from tail contains 3.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- struct insert_at_back_of_sll { // Function takes a constant Book as a parameter, inserts that book at the // back of a singly linked list, and returns nothing. void operator()(const Book& book) { /// TO-DO (3) /// // Write the lines of code to insert "book" at the back of "my_sll". Since // the SLL has no size() function and no tail pointer, you must walk the // list looking for the last node. // // HINT: Do not attempt to insert after "my_sll.end()". // ///// END-T0-DO (3) ||||// } std::forward_list& my_sll; };arrow_forwardTrue/False Select true or false for the statements below. Explain your answers if you like to receive partial credit. 3) Which of the following is true about the insertBeforeCurrent function for a CircularLinked List (CLL) like you did in programming exercise 1?a. If the CLL is empty, you need to create the new node, set it to current, andhave its next pointer refer to itselfb. The worst case performance of the function is O(n)c. If you insert a new element with the same data value as the current node, theperformance improves to O(log n)arrow_forwardCreate a user-defined function called duplicates. This function will check to see if a singly linked list contains nodes with the same data stored. If any duplicates are found, return the value 1. Otherwise return 0. You may use the following typedef structure. typedef struct node_s{ int data; struct node_s * nextptr; }node_t;arrow_forward
- Write a struct ‘Student’ that has member variables: (string) first name, (int) age and (double) fee. Create a sorted (in ascending order according to the age) linked list of three instances of the struct Student. The age of the students must be 21, 24 and 27. Write a function to insert the new instances (elements of the linked list), and insert three instance whose member variable have age (i) 20, (ii) 23 and (iii) 29. Write a function to remove the instances from the linked list, and remove the instances whose member variables have age (i) 21 and (ii) 29. Write a function to count the elements of the linked list. Write a function to search the elements from the linked list, and implement the function to search an element whose age is 23. Write a function to print the linked list on the console. Consider the Student struct as defined above. Create a stack of 5objects of the class. Implement the following (i) push an element to the stack (ii) pop an element from the stack (iii) get the…arrow_forwardJava/Data Structures: The Java Class Library implementation of the interface list return null when an index is out of range. True or Falsearrow_forwardProject 2: Singly-Linked List The purpose of this assignment is to assess your ability to: ▪Implement sequential search algorithms for linked list structures ▪Implement sequential abstract data types using linked data ▪Analyze and compare algorithms for efficiency using Big-O notation For this assignment, you will implement a singly-linked node class. Use your singly-linked node to implement a singly-linked list class that maintains its elements in ascending order. The SinglyLinkedList class is defined by the following data: ▪A node pointer to the front and the tail of the list Implement the following methods in your class: ▪A default constructor list<T> myList ▪A copy constructor list<T> myList(aList) ▪Access to first elementmyList.front() ▪Access to last elementmyList.back() ▪Insert value myList.insert(val) ▪Remove value at frontmyList.pop_front() ▪Remove value at tailmyList.pop_back() ▪Determine if emptymyList.empty() ▪Return # of elementsmyList.size() ▪Reverse order of…arrow_forward
- hi I really need help with this assignment problem flip_matrix(mat:list)->list You will be given a single parameter a 2D list (A list with lists within it) this will look like a 2D matrix when printed out, see examples below. Your job is to flip the matrix on its horizontal axis. In other words, flip the matrix horizontally so that the bottom is at top and the top is at the bottom. Return the flipped matrix. To print the matrix to the console: print('\n'.join([''.join(['{:4}'.format(item) for item in row]) for row in mat])) Example: Matrix:W R I T XH D R L GL K F M VG I S T CW N M N FExpected:W N M N FG I S T CL K F M VH D R L GW R I T X Matrix:L CS PExpected:S PL C Matrix:A D JA Q HJ C IExpected:J C IA Q HA D Jarrow_forward3 4 5 5 7 3 9 3 1 2 B 4 5 7 9 0 2 3 4 5 8 0 1 def calculate_trip_time( iata_src: str, iata_dst: str, flight_walk: List[str], flights: FlightDir ) -> float: Return a float corresponding to the amount of time required to travel from the source airport to the destination airport to the destination airport, as outlined by the flight_walk. PERBE The start time of the trip should be considered zero. In other words, assuming we start the trip at 12:00am, this function should return the time it takes for the trip to finish, including all the waiting times before, and between the flights. If there is no path available, return -1.0 >>> calculate_trip_time("AA1", 2.0 >>> calculate_trip_time("AA1", >>> calculate_trip_time("AA1", >>> calculate_trip_time("AA1", 0.0 >>> calculate_trip_time("AA4", 14.0 >>> calculate_trip_time("AA1", 7.5 >>> calculate_trip_time("AA1", 2.0 *** "AA2", ["AA1", "AA2"], TEST_FLIGHTS_DIR_FOUR_CITIES) "AA7", ["AA7"] "AA1"], TEST_FLIGHTS_DIR_FOUR_CITIES) "AA7", ["AA1",…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
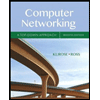
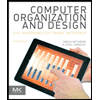
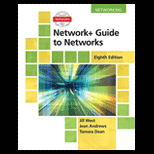
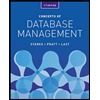
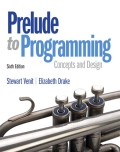
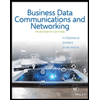