Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 main.cpp #include "MileageTrackerNode.h" #include #include using namespace std; int main () { // References for MileageTrackerNode objects MileageTrackerNode* headNode; MileageTrackerNode* currNode; MileageTrackerNode* lastNode; double miles; string date; int i; // Front of nodes list headNode = new MileageTrackerNode(); lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read // in data and insert into the linked list // TODO: Call the PrintNodeData() method // to print the entire linked list // MileageTrackerNode Destructor deletes all // following nodes delete headNode; } MileageTrackerNode.h #ifndef MILEAGETRACKERNODEH #define MILEAGETRACKERNODEH #include using namespace std; class MileageTrackerNode { public: // Constructor MileageTrackerNode(); // Destructor ~MileageTrackerNode(); // Constructor MileageTrackerNode(double milesInit, string dateInit); // Constructor MileageTrackerNode(double milesInit, string dateInit, MileageTrackerNode* nextLoc); /* Insert node after this node. Before: this -- next After: this -- node -- next */ void InsertAfter(MileageTrackerNode* nodeLoc); // Get location pointed by nextNodeRef MileageTrackerNode* GetNext(); void PrintNodeData(); private: double miles; // Node data string date; // Node data MileageTrackerNode* nextNodeRef; // Reference to the next node }; #endif MileageTrackerNode.cpp #include "MileageTrackerNode.h" #include // Constructor MileageTrackerNode::MileageTrackerNode() { miles = 0.0; date = ""; nextNodeRef = nullptr; } // Destructor MileageTrackerNode::~MileageTrackerNode() { if(nextNodeRef != nullptr) { delete nextNodeRef; } } // Constructor MileageTrackerNode::MileageTrackerNode(double milesInit, string dateInit) { miles = milesInit; date = dateInit; nextNodeRef = nullptr; } // Constructor MileageTrackerNode::MileageTrackerNode(double milesInit, string dateInit, MileageTrackerNode* nextLoc) { miles = milesInit; date = dateInit; nextNodeRef = nextLoc; } /* Insert node after this node. Before: this -- next After: this -- node -- next */ void MileageTrackerNode::InsertAfter(MileageTrackerNode* nodeLoc) { MileageTrackerNode* tmpNext; tmpNext = nextNodeRef; nextNodeRef = nodeLoc; nodeLoc->nextNodeRef = tmpNext; } // Get location pointed by nextNodeRef MileageTrackerNode* MileageTrackerNode::GetNext() { return nextNodeRef; } void MileageTrackerNode::PrintNodeData(){ cout << miles << ", " << date << endl; }
Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node.
Ex. If the input is:
3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18
the output is:
2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18
main.cpp
#include "MileageTrackerNode.h"
#include <string>
#include <iostream>
using namespace std;
int main () {
// References for MileageTrackerNode objects
MileageTrackerNode* headNode;
MileageTrackerNode* currNode;
MileageTrackerNode* lastNode;
double miles;
string date;
int i;
// Front of nodes list
headNode = new MileageTrackerNode();
lastNode = headNode;
// TODO: Read in the number of nodes
// TODO: For the read in number of nodes, read
// in data and insert into the linked list
// TODO: Call the PrintNodeData() method
// to print the entire linked list
// MileageTrackerNode Destructor deletes all
// following nodes
delete headNode;
}
MileageTrackerNode.h
#ifndef MILEAGETRACKERNODEH
#define MILEAGETRACKERNODEH
#include <string>
using namespace std;
class MileageTrackerNode {
public:
// Constructor
MileageTrackerNode();
// Destructor
~MileageTrackerNode();
// Constructor
MileageTrackerNode(double milesInit, string dateInit);
// Constructor
MileageTrackerNode(double milesInit, string dateInit, MileageTrackerNode* nextLoc);
/* Insert node after this node.
Before: this -- next
After: this -- node -- next
*/
void InsertAfter(MileageTrackerNode* nodeLoc);
// Get location pointed by nextNodeRef
MileageTrackerNode* GetNext();
void PrintNodeData();
private:
double miles; // Node data
string date; // Node data
MileageTrackerNode* nextNodeRef; // Reference to the next node
};
#endif
MileageTrackerNode.cpp
#include "MileageTrackerNode.h"
#include <iostream>
// Constructor
MileageTrackerNode::MileageTrackerNode() {
miles = 0.0;
date = "";
nextNodeRef = nullptr;
}
// Destructor
MileageTrackerNode::~MileageTrackerNode() {
if(nextNodeRef != nullptr) {
delete nextNodeRef;
}
}
// Constructor
MileageTrackerNode::MileageTrackerNode(double milesInit, string dateInit) {
miles = milesInit;
date = dateInit;
nextNodeRef = nullptr;
}
// Constructor
MileageTrackerNode::MileageTrackerNode(double milesInit, string dateInit, MileageTrackerNode* nextLoc) {
miles = milesInit;
date = dateInit;
nextNodeRef = nextLoc;
}
/* Insert node after this node.
Before: this -- next
After: this -- node -- next
*/
void MileageTrackerNode::InsertAfter(MileageTrackerNode* nodeLoc) {
MileageTrackerNode* tmpNext;
tmpNext = nextNodeRef;
nextNodeRef = nodeLoc;
nodeLoc->nextNodeRef = tmpNext;
}
// Get location pointed by nextNodeRef
MileageTrackerNode* MileageTrackerNode::GetNext() {
return nextNodeRef;
}
void MileageTrackerNode::PrintNodeData(){
cout << miles << ", " << date << endl;
}
Help me in TODO c++

Step by step
Solved in 3 steps with 1 images

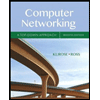
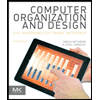
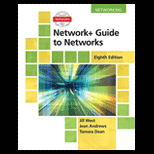
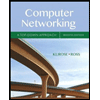
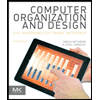
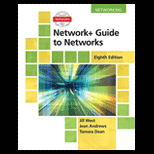
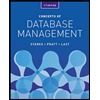
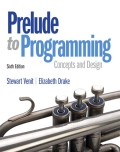
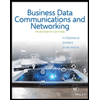