GIVEN //BookMain.java //This is the client code of Book class public class BookMain{ public static void main(String[] arguments){ // Small test of the Book class Book example = new Book("Angels and Demons"); System.out.println("Title (should be Angels and Demons): " + example.getTitle()); System.out.println("Rented? (should be false): " + example.isBorrowed()); example.rented(); //marks the book as rented System.out.println("Rented? (should be true): " + example.isBorrowed()); example.returned();//marks the book as returned System.out.println("Rented? (should be false): " + example.isBorrowed()); } GIVEN //Book.java //complete the missing implementation to this class public class Book{ private String title; private boolean borrowed; // Creates a new Book (constructor) public Book(String bookTitle){ // Implement this method } // Marks the book as rented public void rented() { // Implement this method } // Marks the book as not rented public void returned() { // Implement this method } // Returns true if the book is rented, false otherwise public boolean isBorrowed() { // Implement this method } // Returns the title of the book public String getTitle() { // Implement this method } } GIVEN //LibraryMain.java // This is the client code of Library class //complete the missing static method(s) in this code public class LibraryMain { public static void main(String[] args){ // Create two libraries Library firstLibrary = new Library("101 Big Street"); Library secondLibrary = new Library("228 Wonderland"); // Add four books to the first library firstLibrary.addBook(new Book("Angels and Demons")); firstLibrary.addBook(new Book("The Maze Runner")); firstLibrary.addBook(new Book("I See You")); firstLibrary.addBook(new Book("Fantastic Beasts and Where to Find Them")); // Print opening hours and the addresses System.out.println("Library hours:"); printOpeningHours(); System.out.println(); System.out.println("Library addresses:"); firstLibrary.printAddress(); secondLibrary.printAddress(); System.out.println(); // Try to borrow The Lords of the Rings from both libraries System.out.println("Borrowing The Maze Runner:"); firstLibrary.borrowBook("The Maze Runner"); firstLibrary.borrowBook("The Maze Runner"); secondLibrary.borrowBook("The Maze Runner"); System.out.println(); // Print the titles of all available books from both libraries System.out.println("Books available in the first library:"); firstLibrary.printAvailableBooks(); System.out.println(); System.out.println("Books available in the second library:"); secondLibrary.printAvailableBooks(); System.out.println(); // Return The Maze Runner to the first library System.out.println("Returning The Maze Runner:"); firstLibrary.returnBook("The Maze Runner"); System.out.println(); // Print the titles of available from the first library System.out.println("Books available in the first library:"); firstLibrary.printAvailableBooks(); }//main }
GIVEN
//BookMain.java
//This is the client code of Book class
public class BookMain{
public static void main(String[] arguments){
// Small test of the Book class
Book example = new Book("Angels and Demons");
System.out.println("Title (should be Angels and Demons): " + example.getTitle());
System.out.println("Rented? (should be false): " + example.isBorrowed());
example.rented(); //marks the book as rented
System.out.println("Rented? (should be true): " + example.isBorrowed());
example.returned();//marks the book as returned
System.out.println("Rented? (should be false): " + example.isBorrowed());
}
GIVEN
//Book.java
//complete the missing implementation to this class
public class Book{
private String title;
private boolean borrowed;
// Creates a new Book (constructor)
public Book(String bookTitle){
// Implement this method
}
// Marks the book as rented
public void rented() {
// Implement this method
}
// Marks the book as not rented
public void returned() {
// Implement this method
}
// Returns true if the book is rented, false otherwise
public boolean isBorrowed() {
// Implement this method
}
// Returns the title of the book
public String getTitle() {
// Implement this method
}
}
GIVEN
//LibraryMain.java
// This is the client code of Library class
//complete the missing static method(s) in this code
public class LibraryMain {
public static void main(String[] args){
// Create two libraries
Library firstLibrary = new Library("101 Big Street");
Library secondLibrary = new Library("228 Wonderland");
// Add four books to the first library
firstLibrary.addBook(new Book("Angels and Demons"));
firstLibrary.addBook(new Book("The Maze Runner"));
firstLibrary.addBook(new Book("I See You"));
firstLibrary.addBook(new Book("Fantastic Beasts and Where to Find Them"));
// Print opening hours and the addresses
System.out.println("Library hours:");
printOpeningHours();
System.out.println();
System.out.println("Library addresses:");
firstLibrary.printAddress();
secondLibrary.printAddress();
System.out.println();
// Try to borrow The Lords of the Rings from both libraries
System.out.println("Borrowing The Maze Runner:");
firstLibrary.borrowBook("The Maze Runner");
firstLibrary.borrowBook("The Maze Runner");
secondLibrary.borrowBook("The Maze Runner");
System.out.println();
// Print the titles of all available books from both libraries
System.out.println("Books available in the first library:");
firstLibrary.printAvailableBooks();
System.out.println();
System.out.println("Books available in the second library:");
secondLibrary.printAvailableBooks();
System.out.println();
// Return The Maze Runner to the first library
System.out.println("Returning The Maze Runner:");
firstLibrary.returnBook("The Maze Runner");
System.out.println();
// Print the titles of available from the first library
System.out.println("Books available in the first library:");
firstLibrary.printAvailableBooks();
}//main
}
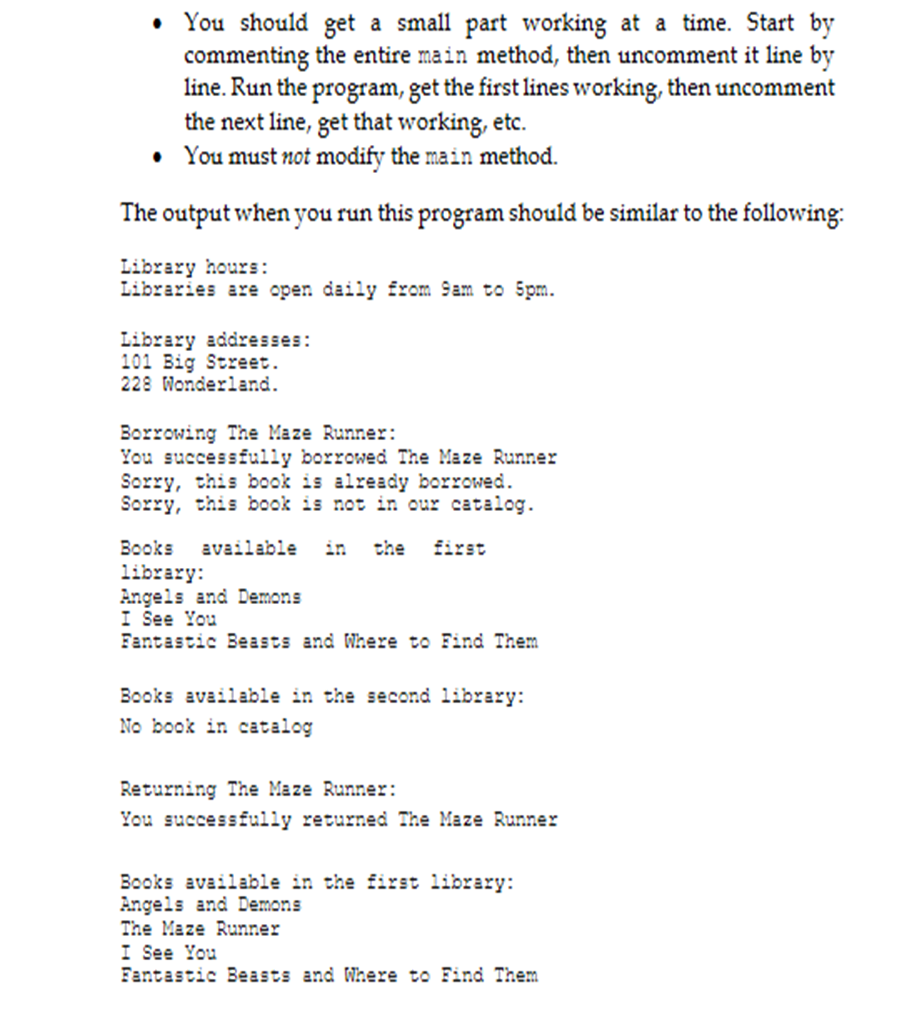
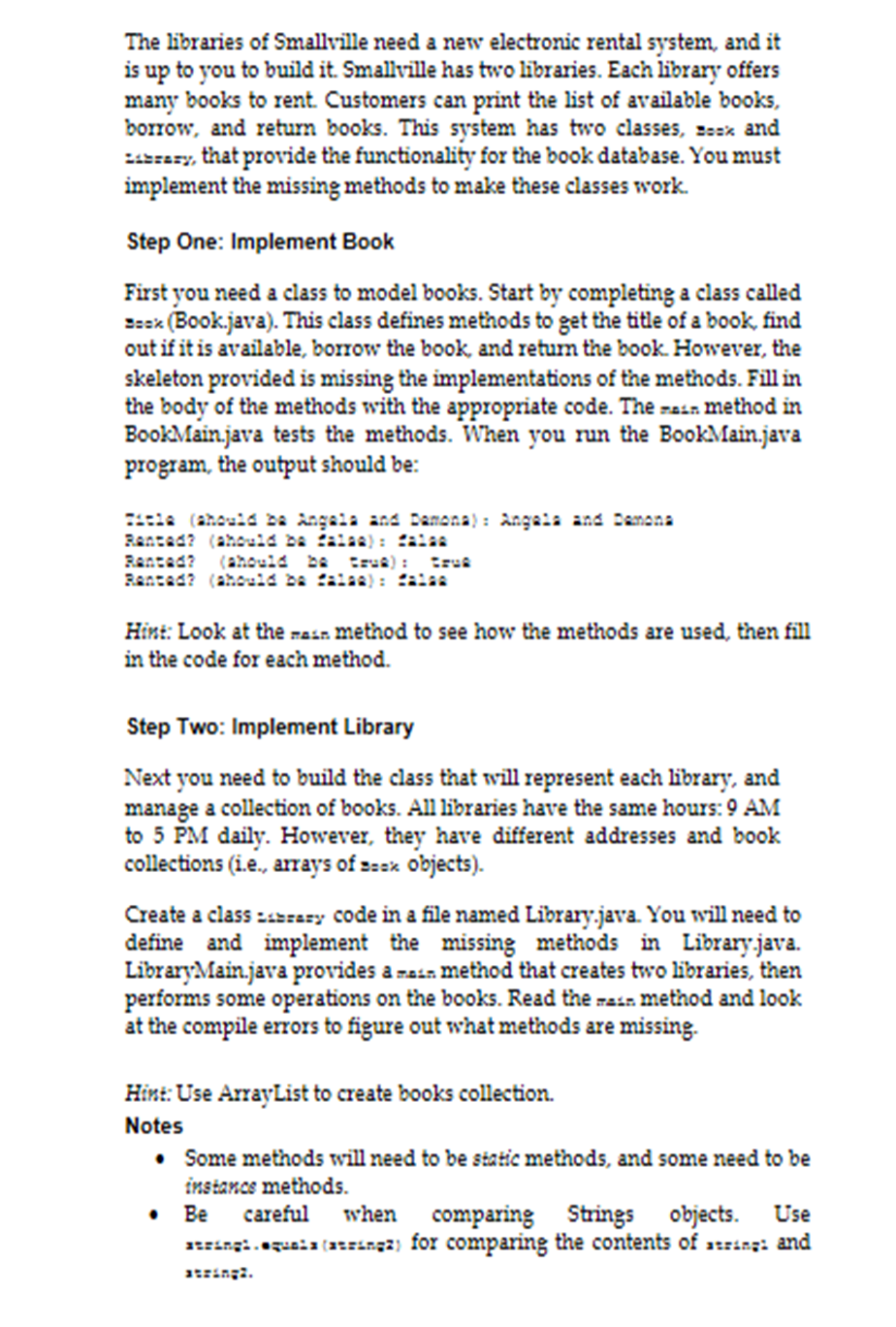

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

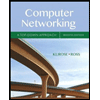
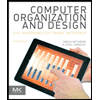
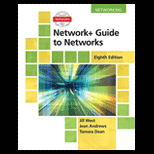
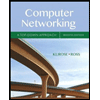
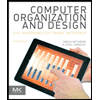
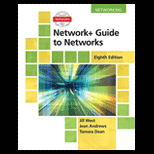
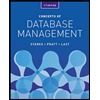
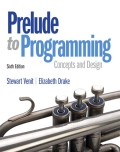
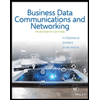