(Java) 7.2 Code: Look at the following Person.java class code: class Person{ public String name; public int age; public String gender; public void greeting() { System.out.println( "Hi, my name is " + name + "!"); } public void greeting(String otherName) { System.out.println("Hi, " + otherName + "!"); } public void printPerson () { System.out.println("Name: "+name); System.out.println("Age: "+age); System.out.println("Gender: "+gender); System.out.println(); } } Remove the printPerson method from this class. Instead, write a toString() method that can be used to display the Student Object in the following manner: Name: Age: Gender: Note the use of the <> above. Do not add these <> to your String. Instead, these <> are commonly used to mean a value will be filled in later. Don't forget the @Override tag in front of the toString() method image source Using the below starter code and the toString method, we will now display the Person object both to the console and to a File. Copy and paste the new test file below into PersonTest.java (you can erase the old contents of the file). Then, update the code to call the toString method to display the Person object both to the console and to a file. Notice the versatility of this method! Finally, refresh your project on Canvas (right-click on the project and select the refresh option). You should see the person.text file appear in the package exploreer. Open this file to verify that you get the correct output in person.txt. /** * PersonTest.java, Activity 7.2 * @author * CIS 36B */ import java.util.Scanner; import java.io.*; public class PersonTest { public static void main(String[] args) throws IOException{ Scanner input = new Scanner(System.in); File file = new File("person.txt"); PrintWriter out = new PrintWriter(file); String name, gender; int age; Person person = new Person(); System.out.print("Welcome!\n\nEnter your name: "); name = input.nextLine(); person.setName(name); System.out.println("You entered: " + person.getName() + "\n"); System.out.print("Enter your age: "); age = input.nextInt(); person.setAge(age); System.out.println("You entered: " + person.getAge() + "\n"); System.out.print("Enter your gender: "); input.nextLine(); gender = input.nextLine(); person.setGender(gender); System.out.println("You entered: " + person.getGender() + "\n"); System.out.println("Your Summary:\n" + //call toString here); out.println("Your Summary: "); //add one line of code to write person to the file - Hint: call toString! out.close(); } } When you get the correct output (as shown below), upload Person.java and PersonTest.java to Canvas Sample Output (Note that user input may vary): Welcome! Enter your name: Marta Gomez You entered: Marta Gomez Enter your age: 25 You entered: 25 Enter your gender: Non binary You entered: Non binary Your Summary: Name: Marta Gomez Age: 25 Gender: Non binary Sample Output in person.txt: Your Summary: Name: Marta Gomez Age: 25 Gender: Non binary
(Java) 7.2 Code: Look at the following Person.java class code: class Person{ public String name; public int age; public String gender; public void greeting() { System.out.println( "Hi, my name is " + name + "!"); } public void greeting(String otherName) { System.out.println("Hi, " + otherName + "!"); } public void printPerson () { System.out.println("Name: "+name); System.out.println("Age: "+age); System.out.println("Gender: "+gender); System.out.println(); } } Remove the printPerson method from this class. Instead, write a toString() method that can be used to display the Student Object in the following manner: Name: Age: Gender: Note the use of the <> above. Do not add these <> to your String. Instead, these <> are commonly used to mean a value will be filled in later. Don't forget the @Override tag in front of the toString() method image source Using the below starter code and the toString method, we will now display the Person object both to the console and to a File. Copy and paste the new test file below into PersonTest.java (you can erase the old contents of the file). Then, update the code to call the toString method to display the Person object both to the console and to a file. Notice the versatility of this method! Finally, refresh your project on Canvas (right-click on the project and select the refresh option). You should see the person.text file appear in the package exploreer. Open this file to verify that you get the correct output in person.txt. /** * PersonTest.java, Activity 7.2 * @author * CIS 36B */ import java.util.Scanner; import java.io.*; public class PersonTest { public static void main(String[] args) throws IOException{ Scanner input = new Scanner(System.in); File file = new File("person.txt"); PrintWriter out = new PrintWriter(file); String name, gender; int age; Person person = new Person(); System.out.print("Welcome!\n\nEnter your name: "); name = input.nextLine(); person.setName(name); System.out.println("You entered: " + person.getName() + "\n"); System.out.print("Enter your age: "); age = input.nextInt(); person.setAge(age); System.out.println("You entered: " + person.getAge() + "\n"); System.out.print("Enter your gender: "); input.nextLine(); gender = input.nextLine(); person.setGender(gender); System.out.println("You entered: " + person.getGender() + "\n"); System.out.println("Your Summary:\n" + //call toString here); out.println("Your Summary: "); //add one line of code to write person to the file - Hint: call toString! out.close(); } } When you get the correct output (as shown below), upload Person.java and PersonTest.java to Canvas Sample Output (Note that user input may vary): Welcome! Enter your name: Marta Gomez You entered: Marta Gomez Enter your age: 25 You entered: 25 Enter your gender: Non binary You entered: Non binary Your Summary: Name: Marta Gomez Age: 25 Gender: Non binary Sample Output in person.txt: Your Summary: Name: Marta Gomez Age: 25 Gender: Non binary
Chapter7: Characters, Strings, And The Stringbuilder
Section: Chapter Questions
Problem 18RQ
Related questions
Question
(Java)
7.2
Code:
- Look at the following Person.java class code:
class Person{
public String name;
public int age;
public String gender;
public void greeting() {
System.out.println( "Hi, my name is " + name + "!");
}
public void greeting(String otherName)
{
System.out.println("Hi, " + otherName + "!");
}
public void printPerson () {
System.out.println("Name: "+name);
System.out.println("Age: "+age);
System.out.println("Gender: "+gender);
System.out.println();
}
}
- Remove the printPerson method from this class.
- Instead, write a toString() method that can be used to display the Student Object in the following manner:
Name: <The Name>
Age: <The Age>
Gender: <The Gender>
- Note the use of the <> above. Do not add these <> to your String. Instead, these <> are commonly used to mean a value will be filled in later.
- Don't forget the @Override tag in front of the toString() method
image source
- Using the below starter code and the toString method, we will now display the Person object both to the console and to a File.
- Copy and paste the new test file below into PersonTest.java (you can erase the old contents of the file).
- Then, update the code to call the toString method to display the Person object both to the console and to a file.
- Notice the versatility of this method!
- Finally, refresh your project on Canvas (right-click on the project and select the refresh option). You should see the person.text file appear in the package exploreer. Open this file to verify that you get the correct output in person.txt.
* PersonTest.java, Activity 7.2
* @author
* CIS 36B
*/
import java.util.Scanner;
import java.io.*;
public class PersonTest {
public static void main(String[] args) throws IOException{
Scanner input = new Scanner(System.in);
File file = new File("person.txt");
PrintWriter out = new PrintWriter(file);
String name, gender;
int age;
Person person = new Person();
System.out.print("Welcome!\n\nEnter your name: ");
name = input.nextLine();
person.setName(name);
System.out.println("You entered: " + person.getName() + "\n");
System.out.print("Enter your age: ");
age = input.nextInt();
person.setAge(age);
System.out.println("You entered: " + person.getAge() + "\n");
System.out.print("Enter your gender: ");
input.nextLine();
gender = input.nextLine();
person.setGender(gender);
System.out.println("You entered: " + person.getGender() + "\n");
System.out.println("Your Summary:\n" + //call toString here);
out.println("Your Summary: ");
//add one line of code to write person to the file - Hint: call toString!
out.close();
}
}
- When you get the correct output (as shown below), upload Person.java and PersonTest.java to Canvas
Sample Output (Note that user input may vary):
Welcome!
Enter your name: Marta Gomez
You entered: Marta Gomez
Enter your age: 25
You entered: 25
Enter your gender: Non binary
You entered: Non binary
Your Summary:
Name: Marta Gomez
Age: 25
Gender: Non binary
Enter your name: Marta Gomez
You entered: Marta Gomez
Enter your age: 25
You entered: 25
Enter your gender: Non binary
You entered: Non binary
Your Summary:
Name: Marta Gomez
Age: 25
Gender: Non binary
Sample Output in person.txt:
Your Summary:
Name: Marta Gomez
Age: 25
Gender: Non binary
![Class: Person
NameſfirstName, lastName]
Age
Gender
Instantiation
Interests
Biot "(Name] is (Age] years old. They like [interests]" }
Greeting "Hil 'm [Namej". }
Object: person1
Name[Bob, Smith]
Age: 32
Gender: Male
Interests: Music, Skiing
Biof "Bob Smith is 32 years old. He likes Music and Skiling.")
Greeting "Hil I'm Bob." }
Object: person2
Name[Diana, Cope)
Age: 28
Gender: Female
Interests: Kickboxing, Brewing
Biof "Diana Cope is 28 years old. She likes Kickboxing and Brewing."}
Greeting "H I'm Diana.")](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2abc661b-c0c9-4b79-9347-0fc323cf483e%2F2e46d18d-c90e-4838-8ce8-da023b3a3559%2Fs8yzz1k_processed.png&w=3840&q=75)
Transcribed Image Text:Class: Person
NameſfirstName, lastName]
Age
Gender
Instantiation
Interests
Biot "(Name] is (Age] years old. They like [interests]" }
Greeting "Hil 'm [Namej". }
Object: person1
Name[Bob, Smith]
Age: 32
Gender: Male
Interests: Music, Skiing
Biof "Bob Smith is 32 years old. He likes Music and Skiling.")
Greeting "Hil I'm Bob." }
Object: person2
Name[Diana, Cope)
Age: 28
Gender: Female
Interests: Kickboxing, Brewing
Biof "Diana Cope is 28 years old. She likes Kickboxing and Brewing."}
Greeting "H I'm Diana.")
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
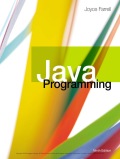
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
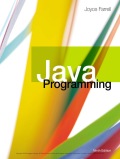
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT