Given main() complete the Stack class by writing the methods push() and pop(). The stack uses an array of size 5 to store elements. The command Push followed by a positive number pushes the number onto the stack. The command Pop pops the top element from the stack. Entering -1 exits the program. Ex. If the input is Push 1 Push 2 Push 3 Push 4 Push 5 Pop -1 the output is Stack contents (top to bottom): 1 Stack contents (top to bottom): 2 1 Stack contents (top to bottom): 3 2 1 Stack contents (top to bottom): 4 3 2 1 Stack contents (top to bottom): 5 4 3 2 1 Stack contents (top to bottom): 4 3 2 1 import java.util.Scanner; public class PushPopStack { public static void main (String[] args) { Scanner scnr = new Scanner(System.in); Stack stack = new Stack(5); String action; // Push or Pop int numInput; // Integer value to push action = scnr.next(); while (!action.equals("-1")) { if (action.equals("Push")) { numInput = scnr.nextInt(); stack.push(numInput); System.out.println("Stack contents (top to bottom):"); stack.printStack(); } else if (action.equals("Pop")) { stack.pop(); System.out.println("Stack contents (top to bottom):"); stack.printStack(); } action = scnr.next(); } } }
Given main() complete the Stack class by writing the methods push() and pop(). The stack uses an array of size 5 to store elements. The command Push followed by a positive number pushes the number onto the stack. The command Pop pops the top element from the stack. Entering -1 exits the program.
Ex. If the input is
Push 1 Push 2 Push 3 Push 4 Push 5 Pop -1
the output is
Stack contents (top to bottom): 1 Stack contents (top to bottom): 2 1 Stack contents (top to bottom): 3 2 1 Stack contents (top to bottom): 4 3 2 1 Stack contents (top to bottom): 5 4 3 2 1 Stack contents (top to bottom): 4 3 2 1
import java.util.Scanner;
public class PushPopStack {
public static void main (String[] args) {
Scanner scnr = new Scanner(System.in);
Stack stack = new Stack(5);
String action; // Push or Pop
int numInput; // Integer value to push
action = scnr.next();
while (!action.equals("-1")) {
if (action.equals("Push")) {
numInput = scnr.nextInt();
stack.push(numInput);
System.out.println("Stack contents (top to bottom):");
stack.printStack();
}
else if (action.equals("Pop")) {
stack.pop();
System.out.println("Stack contents (top to bottom):");
stack.printStack();
}
action = scnr.next();
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

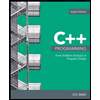
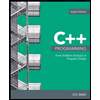