Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce. Carrots Peanuts. where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts.
In Java
import java.util.Scanner;
public class ShoppingList {
public static void main (String[] args) {
Scanner scnr = new Scanner(System.in);
ItemNode headNode; // Create intNode objects
ItemNode currNode;
ItemNode lastNode;
String item;
int i;
// Front of nodes list
headNode = new ItemNode();
lastNode = headNode;
int input = scnr.nextInt();
for(i = 0; i < input; i++ ){
item = scnr.next();
currNode = new ItemNode(item);
lastNode.insertAtEnd(headNode, currNode);
lastNode = currNode;
}
// Print linked list
currNode = headNode.getNext();
while (currNode != null) {
currNode.printNodeData();
currNode = currNode.getNext();
}
}
}
-----------------------------------------
public class ItemNode {
private String item;
private ItemNode nextNodeRef; // Reference to the next node
public ItemNode() {
item = "";
nextNodeRef = null;
}
// Constructor
public ItemNode(String itemInit) {
this.item = itemInit;
this.nextNodeRef = null;
}
// Constructor
public ItemNode(String itemInit, ItemNode nextLoc) {
this.item = itemInit;
this.nextNodeRef = nextLoc;
}
// Insert node after this node.
public void insertAfter(ItemNode nodeLoc) {
ItemNode tmpNext;
tmpNext = this.nextNodeRef;
this.nextNodeRef = nodeLoc;
nodeLoc.nextNodeRef = tmpNext;
}
public void insertAtEnd(String value){
ItemNode aux = this.nextNodeRef;
if (aux == null){
aux = new ItemNode(value);
return;
}
while (aux.getNext() != null){
aux = aux.getNext();
}
setNext(aux, value);
}// TODO: Define insertAtEnd() method that inserts a node
// to the end of the linked list
public void setNext(ItemNode node, String value) {
node.nextNodeRef = new ItemNode(value);
}
// Get location pointed by nextNodeRef
public ItemNode getNext() {
return this.nextNodeRef;
}
public void printNodeData() {
System.out.println(this.item);
}
}
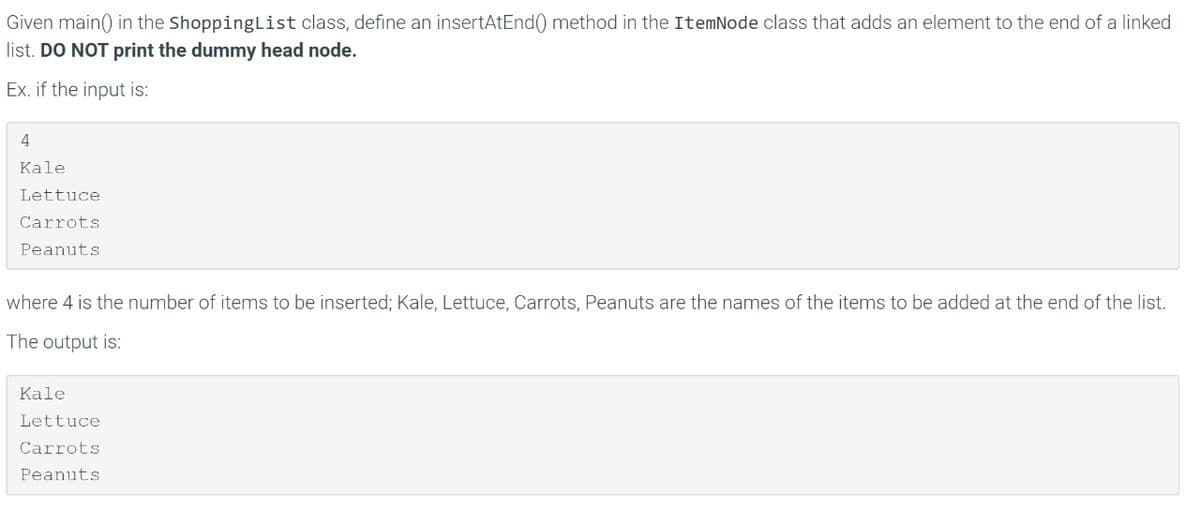

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 6 images

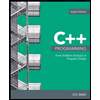
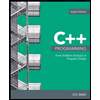