Haunted Mansion.java Haunted Mansion represents the whole of your mansion. It includes all the necessary information and handles most of the functionality for exploring a mansion. A Haunted Mansion should include: • A name. A Player. A 2D array of rooms, representing the layout of the mansion. You should ensure that the room in the first column and first row does not contain a Monster. The goal room the Player is trying to reach. You should ensure that the goal room does not contain a Monster. The goal room should also already be present somewhere in the rooms array. A Scanner, for processing user input. The Haunted Mansion will have only a few behaviors: win • lose move enter This should take no parameters and return nothing. Use this to print out a victory message. This is the same as win, but should instead print out a defeat message. Include whatever you like here! This should prompt the user for a direction to move in. The valid options are "1", "", "u", "d", for left, right, up, and down. The user can also input "map". After any of the three following cases, you should prompt the user for input again: . If input was not one of the valid options, print out a message saying as much. If the chosen direction would be out of bounds (of the rooms array) print out a message saying: There is only a wall in that direction! If the Room in the chosen direction is locked, print out a message saying: The room in that direction is locked off! If the input was "map", print out the current map of the rooms which have been explored (you can use HauntedHelper for this, but do not use createFullMansionMap). Otherwise, you should update the Player's location indices to reflect moving to the Room in the specified direction. You should also update the Player's previous location indices before changing their current location indices. This will take no parameters and return nothing. Print out a short introduction message. Something like: (player name) enters (mansion name). The halls are dimly lit and sounds echo from deeper within... (player name) looks behind them only to see the entrance is gone! They'll have to find a way out... This method will contain the main program loop, where you will flow through a sequence of events until either the Player runs out of courage (a losing scenario) or reaches the goal room (a winning scenario). The events are as follows: ■ Print out: • (player name} looks around the room... Explore the current Room. This is the Room in the rooms array at the Player's current position. • If the Room is the goal room, begin the win sequence and stop the loop The Player should look for Scooby Snacks in the room. If the Room has a Monster, it should frighten and then haunt the Player. Then, let the user choose the next move (you have a method for this). If the Player ever runs out of courage, you should begin the lose sequence and stop the loop.
Haunted Mansion.java Haunted Mansion represents the whole of your mansion. It includes all the necessary information and handles most of the functionality for exploring a mansion. A Haunted Mansion should include: • A name. A Player. A 2D array of rooms, representing the layout of the mansion. You should ensure that the room in the first column and first row does not contain a Monster. The goal room the Player is trying to reach. You should ensure that the goal room does not contain a Monster. The goal room should also already be present somewhere in the rooms array. A Scanner, for processing user input. The Haunted Mansion will have only a few behaviors: win • lose move enter This should take no parameters and return nothing. Use this to print out a victory message. This is the same as win, but should instead print out a defeat message. Include whatever you like here! This should prompt the user for a direction to move in. The valid options are "1", "", "u", "d", for left, right, up, and down. The user can also input "map". After any of the three following cases, you should prompt the user for input again: . If input was not one of the valid options, print out a message saying as much. If the chosen direction would be out of bounds (of the rooms array) print out a message saying: There is only a wall in that direction! If the Room in the chosen direction is locked, print out a message saying: The room in that direction is locked off! If the input was "map", print out the current map of the rooms which have been explored (you can use HauntedHelper for this, but do not use createFullMansionMap). Otherwise, you should update the Player's location indices to reflect moving to the Room in the specified direction. You should also update the Player's previous location indices before changing their current location indices. This will take no parameters and return nothing. Print out a short introduction message. Something like: (player name) enters (mansion name). The halls are dimly lit and sounds echo from deeper within... (player name) looks behind them only to see the entrance is gone! They'll have to find a way out... This method will contain the main program loop, where you will flow through a sequence of events until either the Player runs out of courage (a losing scenario) or reaches the goal room (a winning scenario). The events are as follows: ■ Print out: • (player name} looks around the room... Explore the current Room. This is the Room in the rooms array at the Player's current position. • If the Room is the goal room, begin the win sequence and stop the loop The Player should look for Scooby Snacks in the room. If the Room has a Monster, it should frighten and then haunt the Player. Then, let the user choose the next move (you have a method for this). If the Player ever runs out of courage, you should begin the lose sequence and stop the loop.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:Haunted Mansion.java
Haunted Mansion represents the whole of your mansion. It includes all the necessary information and
handles most of the functionality for exploring a mansion. A Haunted Mansion should include:
•
A name.
A Player.
A 2D array of rooms, representing the layout of the mansion. You should ensure that the room
in the first column and first row does not contain a Monster.
The goal room the Player is trying to reach. You should ensure that the goal room does not
contain a Monster. The goal room should also already be present somewhere in the rooms
array.
A Scanner, for processing user input.
The Haunted Mansion will have only a few behaviors:
•
win
lose
move
This should take no parameters and return nothing. Use this to print out a victory
message.
This is the same as win, but should instead print out a defeat message. Include whatever
you like here!
• This should prompt the user for a direction to move in. The valid options are "1",
"", "u", "d", for left, right, up, and down. The user can also input "map".
。 After any of the three following cases, you should prompt the user for input again:
enter
•
•
If input was not one of the valid options, print out a message saying as much.
If the chosen direction would be out of bounds (of the rooms array) print out a
message saying:
There is only a wall in that direction!
If the Room in the chosen direction is locked, print out a message saying:
The room in that direction is locked off!
If the input was "map", print out the current map of the rooms which have
been explored (you can use HauntedHelper for this, but do not use
createFullMansionMap).
Otherwise, you should update the Player's location indices to reflect moving to the
Room in the specified direction. You should also update the Player's previous location
indices before changing their current location indices.
This will take no parameters and return nothing.
Print out a short introduction message. Something like:
(player name} enters (mansion name}. The halls are
dimly lit and sounds echo from deeper within...
(player name} looks behind them only to see the
entrance is gone! They'll have to find a way out...
• This method will contain the main program loop, where you will flow through a
sequence of events until either the Player runs out of courage (a losing scenario) or
reaches the goal room (a winning scenario). The events are as follows:
■ Print out:
• {player name} looks around the room...
Explore the current Room. This is the Room in the rooms array at the Player's
current position.
• If the Room is the goal room, begin the win sequence and stop the loop.
■ The Player should look for Scooby Snacks in the room.
■
■
If the Room has a Monster, it should frighten and then haunt the Player.
Then, let the user choose the next move (you have a method for this).
If the Player ever runs out of courage, you should begin the lose sequence and
stop the loop.

Transcribed Image Text:be putting them
in one! You will set the stage for their newest adventure, a lexd-based command line adventure
game, in which the
gang must escape a Haunted Mansion.
You will define several classes and hierarchies that take advantage of object-oriented concepts to
bandle the different events and scenarios in your mansion. This will include a set of Rooms,
Monsters, and a Player
the arrangement, or Loorplan, of rooms. A Player always begins in the middlemost Room of the
mansion. An exit toom will be specia. ly designated. "The layer can move left, right, up, or down
one slep (Room) during each round of the game. The Player begins the game with a love
immediately lose the game.
Player enters a Room, they may encounter a Monster. Á Monster encounter will take a toll on a
layer's courage. Monsters come in different varieties, and their respective effects on players
ou will have a base Monster class and some subclasses to create different types of
Monsters.
While the scary monsters are there to thwart you, fortunately there will be Scooby Snacks in a
Player's courage.
The data members for your classes should be only accessible via appropriate setier and getter
soccïfied. All explicitly described methods should be public unless otherwise stated, and
nd you
may create additional private helper methods wherever necessary. Similarly, all instance data
should be private unless otherwise stated.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
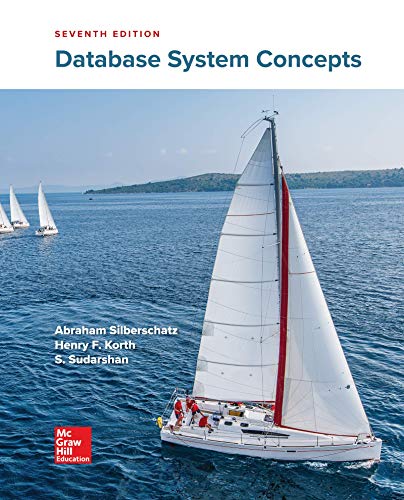
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
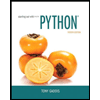
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
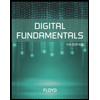
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
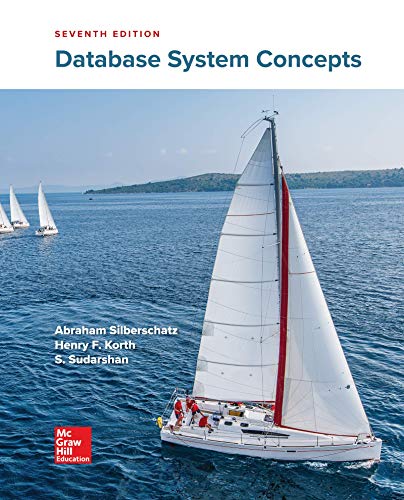
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
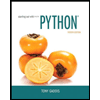
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
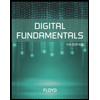
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
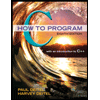
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
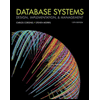
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
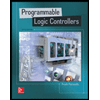
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education