he weather generator methods you will be writing for this assignment will: predict future precipitation pattern for one month: oneMonthGenerator find the number of wet or dry days in a given month’s forecast: numberOfWetDryDays find the longest wet or dry spell in a given month’s forecast: lengthOfLongestWetDrySpell
he weather generator methods you will be writing for this assignment will: predict future precipitation pattern for one month: oneMonthGenerator find the number of wet or dry days in a given month’s forecast: numberOfWetDryDays find the longest wet or dry spell in a given month’s forecast: lengthOfLongestWetDrySpell
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
The weather generator methods you will be writing for this assignment will:
- predict future precipitation pattern for one month: oneMonthGenerator
- find the number of wet or dry days in a given month’s forecast: numberOfWetDryDays
- find the longest wet or dry spell in a given month’s forecast: lengthOfLongestWetDrySpell
![Future transition probability table as a 2D array
The oneMonthGenerator method receives as arguments the transition probability tables (dry to wet, and wet to wet) as 2D arrays.
Each table row corresponds to a location (longitude, latitude) in the USA and contains the transition probabilities
for each month of the year.
Longitude Latitude January February March April May June July August September October November December
-97.58
26.02
0.76
0.75
0.77 0.74 0.80 0.86 0.94 0.97
0.89
0.77
0.74
0.77
Following are the methods to be completed in WeatherGenerator.java:
public class WeatherGenerator {
/* Given a location (longitude, latitude) in the USA and a month of the year, the method
* returns the forecast for the month based on the drywet and wetwet transition
* probabilities tables.
*
* month will be a value between 2 and 13: 2 corresponds to January, 3 corresponds to February
* and so on. These are the column indexes of each month in the transition probabilities tables.
* The first day of the month has a 50% chance to be a wet day, 0-0.49 (wet), 0.50-0.99 (dry)
* Use StdRandom.uniform() to generate a real number uniformly in [0,1)
* /
int[] oneMonthGenerator (double longitute, double latitude, int month, double0] drywet, double[][] wetwet)
// Returns the longest number of consecutive mode (WET or DRY) days in forecast.
int numberofWetDryDays (int[] forecast, int mode)
/ *
* Analyzes the forecast array and returns the longest number of
* consecutive mode (which can be WET or DRY) days in forecast.
* /
int lengthofLongestWetDrySpell (int[] forecast, int mode)
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F801e7fe1-493b-4a36-a14c-4533a3782dfd%2F2137a2bb-8452-4f98-9c2b-bc79243e6796%2Fptxg4v_processed.png&w=3840&q=75)
Transcribed Image Text:Future transition probability table as a 2D array
The oneMonthGenerator method receives as arguments the transition probability tables (dry to wet, and wet to wet) as 2D arrays.
Each table row corresponds to a location (longitude, latitude) in the USA and contains the transition probabilities
for each month of the year.
Longitude Latitude January February March April May June July August September October November December
-97.58
26.02
0.76
0.75
0.77 0.74 0.80 0.86 0.94 0.97
0.89
0.77
0.74
0.77
Following are the methods to be completed in WeatherGenerator.java:
public class WeatherGenerator {
/* Given a location (longitude, latitude) in the USA and a month of the year, the method
* returns the forecast for the month based on the drywet and wetwet transition
* probabilities tables.
*
* month will be a value between 2 and 13: 2 corresponds to January, 3 corresponds to February
* and so on. These are the column indexes of each month in the transition probabilities tables.
* The first day of the month has a 50% chance to be a wet day, 0-0.49 (wet), 0.50-0.99 (dry)
* Use StdRandom.uniform() to generate a real number uniformly in [0,1)
* /
int[] oneMonthGenerator (double longitute, double latitude, int month, double0] drywet, double[][] wetwet)
// Returns the longest number of consecutive mode (WET or DRY) days in forecast.
int numberofWetDryDays (int[] forecast, int mode)
/ *
* Analyzes the forecast array and returns the longest number of
* consecutive mode (which can be WET or DRY) days in forecast.
* /
int lengthofLongestWetDrySpell (int[] forecast, int mode)
}
![Use the main method as a driver to test your methods. To generate the weather for location at longitude -98.76 and latitude 26.70 for the month of
February do:
java WeatherGenerator111 -98.76 26.7O 3
public static void main (String[] args) {
int number0fRows
= 4001; // Total number of locations
// Total number of 14 columns in file
// File format: longitude, latitude, 12 months of transition probabilities
int number0fColumns =
14;
// Allocate and populate arrays that hold the transition probabilities
double[][] drywet
double[][] wetwet = new double[numberofRows ] [ numberOfColumns];
populateTransitionProbabilitiesArrays (drywet, wetwet, numberofRows);
new double[number0fRows ] [numberOfColumns];
%D
/*** WRITE YOUR CODE BELLOW THIS LINE. DO NOT erase any of the lines above. ***/
// Read command line inputs
double longitute
Double.parseDouble(args[0]);
Double.parseDouble(args[1]);
= Integer.parseInt(args[2]);
double latitude
%3D
int
month
int[] forecast = oneMonthGenerator(longitute, latitude, month, drywet, wetwet);
int drySpell
int wetSpell
lengthofLongestSpell(forecast, DRY);
lengthofLongestSpell(forecast, WET);
%3D
%3D
+ month);
Stdout.println("There are
StdOut.println(drySpell +
+ forecast.length +
days of dry spell.");
days in the forecast for month
for ( int i =
0; i < forecast.length; i++ ) {
// This is the ternary operator. (conditional) ? executed if true : executed if false
String weather =
StdOut.println("Day
WET) ? "Wet"
is forecasted to be
: "Dry";
(forecast[i]
+ (i+1) +
+ weather);
}
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F801e7fe1-493b-4a36-a14c-4533a3782dfd%2F2137a2bb-8452-4f98-9c2b-bc79243e6796%2F6ke3hft_processed.png&w=3840&q=75)
Transcribed Image Text:Use the main method as a driver to test your methods. To generate the weather for location at longitude -98.76 and latitude 26.70 for the month of
February do:
java WeatherGenerator111 -98.76 26.7O 3
public static void main (String[] args) {
int number0fRows
= 4001; // Total number of locations
// Total number of 14 columns in file
// File format: longitude, latitude, 12 months of transition probabilities
int number0fColumns =
14;
// Allocate and populate arrays that hold the transition probabilities
double[][] drywet
double[][] wetwet = new double[numberofRows ] [ numberOfColumns];
populateTransitionProbabilitiesArrays (drywet, wetwet, numberofRows);
new double[number0fRows ] [numberOfColumns];
%D
/*** WRITE YOUR CODE BELLOW THIS LINE. DO NOT erase any of the lines above. ***/
// Read command line inputs
double longitute
Double.parseDouble(args[0]);
Double.parseDouble(args[1]);
= Integer.parseInt(args[2]);
double latitude
%3D
int
month
int[] forecast = oneMonthGenerator(longitute, latitude, month, drywet, wetwet);
int drySpell
int wetSpell
lengthofLongestSpell(forecast, DRY);
lengthofLongestSpell(forecast, WET);
%3D
%3D
+ month);
Stdout.println("There are
StdOut.println(drySpell +
+ forecast.length +
days of dry spell.");
days in the forecast for month
for ( int i =
0; i < forecast.length; i++ ) {
// This is the ternary operator. (conditional) ? executed if true : executed if false
String weather =
StdOut.println("Day
WET) ? "Wet"
is forecasted to be
: "Dry";
(forecast[i]
+ (i+1) +
+ weather);
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
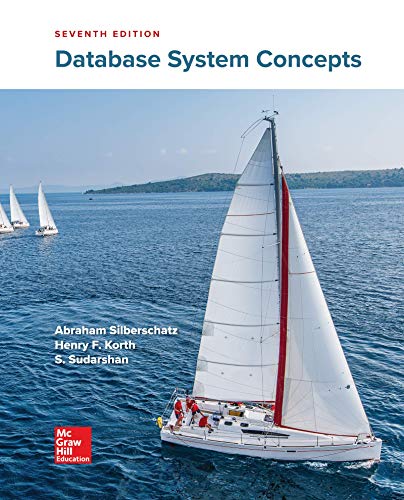
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
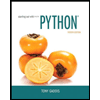
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
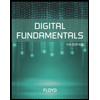
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
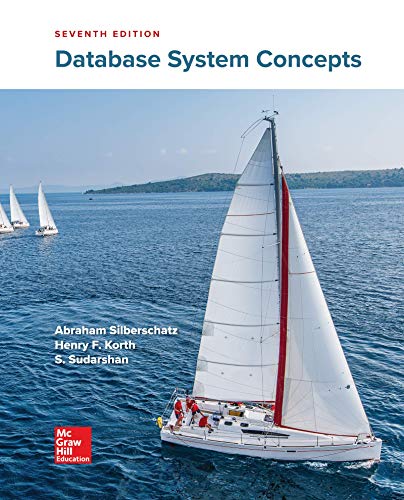
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
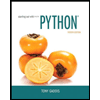
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
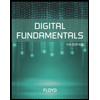
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
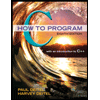
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
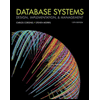
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
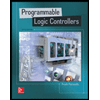
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education