Hello, I am required to solve the simulation case by using DYNAMIC QUEUE (linked-list) but the solution by using static queue (array)
Hello, I am required to solve the simulation case by using DYNAMIC QUEUE (linked-list) but the solution by using static queue (array) are given as follows:
(Note that I use Dev-C++)
//Header file: queueADT.h
#ifndef H_queueADT
#define H_queueADT
template <class Type>
class queueADT
{
public:
virtual bool isEmptyQueue() const = 0;
virtual bool isFullQueue() const = 0;
virtual void initializeQueue() = 0;
virtual Type front() const = 0;
virtual Type back() const = 0;
virtual void addQueue(const Type& queueElement) = 0;
virtual void deleteQueue() = 0;
};
#endif
//Header file: QueueasArray.h
#ifndef H_QueueAsArray
#define H_QueueAsArray
#include <iostream>
#include <cassert>
#include "queueADT.h"
using namespace std;
template <class Type>
class queueType: public queueADT <Type>
{
public:
const queueType <Type>& operator = (const queueType <Type>&);
//Overload the assignment operator
bool isEmptyQueue() const;
bool isFullQueue() const;
void initializeQueue();
Type front() const;
Type back() const;
void addQueue(const Type& queueElement);
void deleteQueue();
queueType(int queueSize = 100);
//Constructor
queueType(const queueType<Type>& otherQueue);
//Copy constructor
~queueType();
//Destructor
private:
int maxQueueSize;
int count;
int queueFront;
int queueRear;
Type *list;
};
template <class Type>
bool queueType<Type>::isEmptyQueue() const
{
return (count == 0);
}//end isEmptyQueue
template <class Type>
bool queueType<Type>::isFullQueue() const
{
return (count == maxQueueSize);
}//end isFullQueue
template <class Type>
void queueType<Type>::initializeQueue()
{
queueFront = 0;
queueRear = maxQueueSize - 1;
count = 0;
}// end initializeQueue
template <class Type>
Type queueType<Type>::front() const
{
assert (!isEmptyQueue());
return list [queueFront];
}// end front
template <class Type>
Type queueType<Type>::back() const
{
assert(!isEmptyQueue());
return list[queueRear];
}// end back
template <class Type>
void queueType<Type>::addQueue(const Type& newElement)
{
if (!isFullQueue())
{
queueRear = (queueRear + 1) % maxQueueSize; //use mod
//operator to advance queueRear
//because the array is circular
count++;
list[queueRear] = newElement;
}
else
cout << "Cannot add to a full queue." << endl;
} //end addQueue
template <class Type>
void queueType<Type>::deleteQueue()
{
if (!isEmptyQueue())
{
count--;
queueFront = (queueFront + 1) % maxQueueSize; //use the
//mod operator to advance queueFront
//because the array is circular
}
else
cout << "Cannot remove from an empty queue." << endl;
} //end deleteQueue
//Constructor
template <class Type>
queueType<Type>::queueType(int queueSize)
{
if (queueSize <= 0)
{
cout << "Size of the array to hold the queue must "
<< "be positive." << endl;
cout << "Creating an array of size 100." << endl;
maxQueueSize = 100;
}
else
maxQueueSize = queueSize; //set maxQueueSize to
//queueSize
queueFront = 0; //initialize queueFront
queueRear = maxQueueSize - 1; //initialize queueRear
count = 0;
list = new Type[maxQueueSize]; //create the array to
//hold the queue elements
} //end constructor
//Destructor
template <class Type>
queueType<Type>::~queueType()
{
delete [] list;
} //end destructor
template <class Type>
const queueType<Type>& queueType<Type>::operator=
(const queueType<Type>& otherQueue)
{
cout << "Write the definition of the function "
<< "to overload the assignment operator." << endl;
} //end assignment operator
template <class Type>
queueType<Type>::queueType(const queueType<Type>& otherQueue)
{
cout << "Write the definition of the copy constructor."
<< endl;
} //end copy constructor
#endif



Step by step
Solved in 4 steps with 5 images

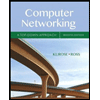
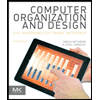
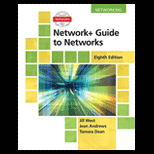
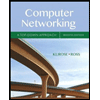
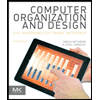
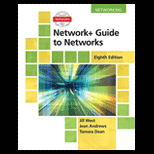
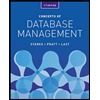
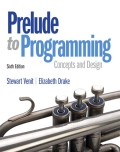
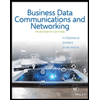