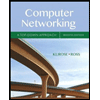
Instruction: To test the Linked List class, create a new Java class with the main method, generate Linked List using Integer and check whether all methods do what they’re supposed to do. A sample Java class with main method is provided below including output generated. If you encounter errors, note them and try to correct the codes. Post the changes in your code, if any.
Additional Instruction: Linked List is a part of the Collection framework present in java.util package, however, to be able to check the complexity of Linked List operations, we can recode the data structure based on Java Documentation https://docs.oracle.com/javase/8/docs/api/java/util/LinkedList.html
package com.linkedlist;
public class linkedListTester {
public static void main(String[] args) {
ListI<Integer> list = new LinkedList<Integer>();
int n=10;
for(int i=0;i<n;i++) {
list.addFirst(i);
}
for(int i=0;i<n;i++) {
list.addLast(i);
}
list.removeFirst();
list.removeLast();
list.remove(8);
System.out.println(list.contains(8));
System.out.println(list.peekFirst());
System.out.println(list.peekLast());
System.out.println(list.size());
}
}
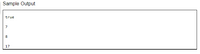

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- True or False? Our LBList class inherits from the ABList class. Please explain.arrow_forwardGiven main.py and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insert_in_descending_order() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 the output is: 9 8 7 6 5 4 3 2 1 main.py(can not edit) from IntNode import IntNodefrom IntList import IntList if __name__ == "__main__": int_list = IntList() input_line = input() input_strings = input_line.split(' ') for num_string in input_strings: # Convert from string to integer num = int(num_string) # Insert into linked list in descending order new_node = IntNode(num) int_list.insert_in_descending_order(new_node) int_list.print_int_list() IntNode.py(can not edit) class IntNode: def __init__(self, initial_data, next = None, prev = None): self.data = initial_data self.next = next self.prev = prev IntList.py(need to edit)arrow_forwardLooking at all four list implementations, which actions/methods tend to be less efficient in the Linked List implementation compared to the Array Implementation? Explain why for each action/method you specify.arrow_forward
- maxLength Language/Type: Related Links: Java Set collections Set Write a method maxLength that accepts as a parameter a Set of strings, and that returns the length of the longest string in the set. If your method is passed an empty set, it should return 0. 1 9 10 Method: Write a Java method as described, not a complete program or class. N345678 2arrow_forwardJAVA please Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Code provided in the assignment ItemNode.java:arrow_forwardI don't get the "//To be implemented" comments, what am I supposed to do?Also, are the header files and header files implementation different? Like is the linkedList.h different than the linkedList.h (implementation)?arrow_forward
- Java Programming language Help please.arrow_forwardGiven the source code of linked List, answer the below questions(image): A. Fill out the method printList that print all the values of the linkedList: Draw the linked list. public void printList() { } // End of print method B. Write the lines to insert 10 at the end of the linked list. You must draw the final linked List. Notice that you can’t use second or third nodes. Feel free to define a new node. Assume you have only a head node C. Write the lines to delete node 2. You must draw the final linked list. Notice that you can’t use second or third node. Feel free to define a new node. Assume you have only a head nodearrow_forwardLinked List, create your own code. (Do not use the build in function or classes of Java or from the textbook). Create a LinkedList class: Call the class MyLinkedList, (hint) Create a second class called Node.java and use it, remember in the class I put the Node class inside the LinkedList Class, but you should do it outside. This class should haveo Variables you may need for a Node,o (optional) Constructor Your linked list is of an int type. (you may do it as General type as <E>) For this Linked List you need to have the following methods: add, addAfter, remove, size, contain, toString, compare, addInOrder. This is just a suggestion, if you use Generic type, you must modify this Write a main function or Main class to test all the methods,o Create a 2 linked list and test all your methods. (Including the compare)arrow_forward
- Suppose you have created a new class: SortedLinkedList. This class is derived from LinkedList (single links). You are asked to overload the method Insert. The method Insert will no longer take in a parameter position, this is because now Insert will place the element in the correct position such that the list is always sorted. Write the C++ code for the implementation of that Insert method.arrow_forwardPlease fill in all the code gaps if possible: (java) public class LinkedList { privateLinkedListNode head; **Creates a new instance of LinkedList** public LinkedList () { } public LinkedListNode getHead() public void setHead (LinkedListNode head) **Add item at the front of the linked list** public void insertFront (Object item) **Remove item at the front of linked list and return the object variable** public Object removeFront() }arrow_forwardSimple linkedlist implementation please help (looks like a lot but actually isnt, everything is already set up for you below to make it EVEN easier) help in any part you can be clear Given an interface for LinkedList- Without using the java collections interface (ie do not import java.util.List,LinkedList, Stack, Queue...)- Create an implementation of LinkedList interface provided- For the implementation create a tester to verify the implementation of thatdata structure performs as expected Build Bus Route – Linked List- You’ll be provided a java package containing an interface, data structure,implementation shell, test harness shell below.- Your task is to:o Implement the LinkedList interface (fill out the implementation shell)o Put your implementation through its paces by exercising each of themethods in the test harnesso Create a client (a class with a main) ‘BusClient’ which builds a busroute by performing the following operations on your linked list:o§ Create (insert) 4 stations§…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
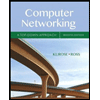
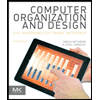
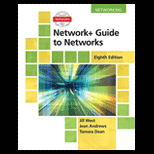
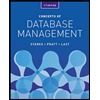
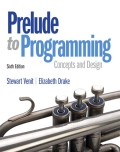
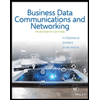