Hello, I have written my random bear generator but for some reason the code isn't running in my command terminal. Please have a look at it and let me know what I am missing. I followed my teachers instructors and lectures, but it doesn't run on another compiler either. class Bear { constructor(type, color, weight, favoriteFood) { this.type = type; this.color = color; this.weight = weight; this.favoriteFood = favoriteFood; if (this.weight === 700 || this.type === "Little Bear" || this.type === "Baby Bear") { this.isAggressive = false; } else if (this.weight === 1200 || this.type === "Papa Bear" || this.type === "Black Bear") { this.isAggressive = true; } else { this.isAggressive = Math.random() < 0.5; } if (this.type === "Winnie the Pooh Bear" && this.favoriteFood !== "Honey") { throw new Error("Winnie the Pooh Bear must have Honey as his favorite food."); } } } class BearGenerator { get types() { return [ "Winnie the Pooh Bear", "Yogi Bear", "Boo-boo Bear", "Paddington Bear", "Mama Bear", "Papa Bear", "Baby Bear", "Little Bear", "Teddy Bear", "Smokey Bear", ]; } get colors() { return [ "Red", "Blue", "White", "Indigo", "Green", "Teal", "Fuchsia", "Brown", "Violet", "Black", ]; } get foods() { return [ "Honey", "Pizza", "Burger", "Burrito", "Wings", "Spaghetti", "Steak", ]; } createRandomBear() { const type = this.types[Math.floor(Math.random() * this.types.length)]; const color = this.colors[Math.floor(Math.random() * this.colors.length)]; const weight = Math.floor(Math.random() * 1250) + 250; const favoriteFood = this.foods[Math.floor(Math.random() * this.foods.length)]; return new Bear(type, color, weight, favoriteFood); } } class DataProcessor { constructor(data) { this.data = data; } getStatsFor(valuesArray, property) { const stats = {}; for (const value of valuesArray) { stats[value] = 0; } for (const bear of this.data) { stats[bear[property]] += 1; } for (const value of valuesArray) { stats[value] = (stats[value] / this.data.length) * 100; } return stats; } } function randomBears(numberOfBears) { const bearGenerator = new BearGenerator(); const bears = []; for (let i = 0; i < numberOfBears; i++) { bears.push(bearGenerator.createRandomBear()); } const dataProcessor = new DataProcessor(bears); console.log("Favorite Food Stats:"); console.log(dataProcessor.getStatsFor(bearGenerator.foods, "favoriteFood")); console.log("Type Stats:"); console.log(dataProcessor.getStatsFor(bearGenerator.types, "type")); console.log("Color Stats:"); console.log(dataProcessor.getStatsFor(bearGenerator.colors, "color")); console.log("Aggression Stats:"); console.log(dataProcessor.getStatsFor([true, false], "isAggressive")); return bears; }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Hello, I have written my random bear generator but for some reason the code isn't running in my command terminal. Please have a look at it and let me know what I am missing. I followed my teachers instructors and lectures, but it doesn't run on another compiler either.
class Bear {
constructor(type, color, weight, favoriteFood) {
this.type = type;
this.color = color;
this.weight = weight;
this.favoriteFood = favoriteFood;
if (this.weight === 700 || this.type === "Little Bear" || this.type === "Baby Bear") {
this.isAggressive = false;
} else if (this.weight === 1200 || this.type === "Papa Bear" || this.type === "Black Bear") {
this.isAggressive = true;
} else {
this.isAggressive = Math.random() < 0.5;
}
if (this.type === "Winnie the Pooh Bear" && this.favoriteFood !== "Honey") {
throw new Error("Winnie the Pooh Bear must have Honey as his favorite food.");
}
}
}
class BearGenerator {
get types() {
return [
"Winnie the Pooh Bear",
"Yogi Bear",
"Boo-boo Bear",
"Paddington Bear",
"Mama Bear",
"Papa Bear",
"Baby Bear",
"Little Bear",
"Teddy Bear",
"Smokey Bear",
];
}
get colors() {
return [
"Red",
"Blue",
"White",
"Indigo",
"Green",
"Teal",
"Fuchsia",
"Brown",
"Violet",
"Black",
];
}
get foods() {
return [
"Honey",
"Pizza",
"Burger",
"Burrito",
"Wings",
"Spaghetti",
"Steak",
];
}
createRandomBear() {
const type = this.types[Math.floor(Math.random() * this.types.length)];
const color = this.colors[Math.floor(Math.random() * this.colors.length)];
const weight = Math.floor(Math.random() * 1250) + 250;
const favoriteFood = this.foods[Math.floor(Math.random() * this.foods.length)];
return new Bear(type, color, weight, favoriteFood);
}
}
class DataProcessor {
constructor(data) {
this.data = data;
}
getStatsFor(valuesArray, property) {
const stats = {};
for (const value of valuesArray) {
stats[value] = 0;
}
for (const bear of this.data) {
stats[bear[property]] += 1;
}
for (const value of valuesArray) {
stats[value] = (stats[value] / this.data.length) * 100;
}
return stats;
}
}
function randomBears(numberOfBears) {
const bearGenerator = new BearGenerator();
const bears = [];
for (let i = 0; i < numberOfBears; i++) {
bears.push(bearGenerator.createRandomBear());
}
const dataProcessor = new DataProcessor(bears);
console.log("Favorite Food Stats:");
console.log(dataProcessor.getStatsFor(bearGenerator.foods, "favoriteFood"));
console.log("Type Stats:");
console.log(dataProcessor.getStatsFor(bearGenerator.types, "type"));
console.log("Color Stats:");
console.log(dataProcessor.getStatsFor(bearGenerator.colors, "color"));
console.log("Aggression Stats:");
console.log(dataProcessor.getStatsFor([true, false], "isAggressive"));
return bears;
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 7 images

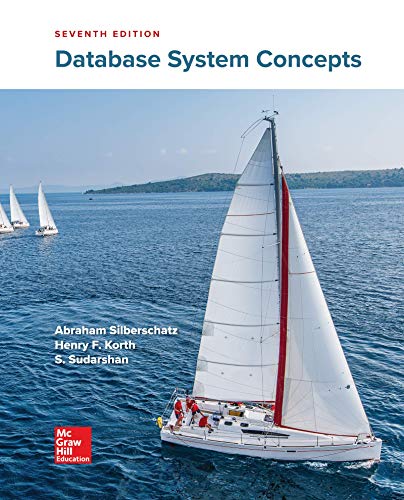
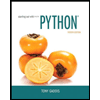
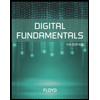
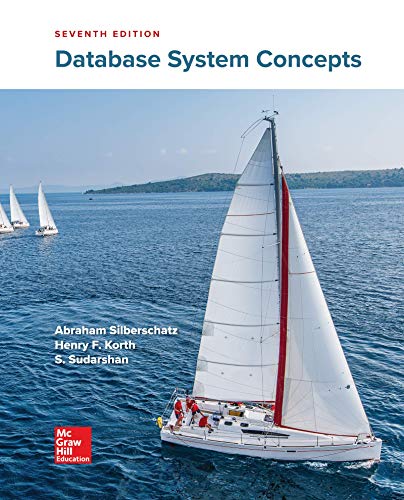
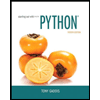
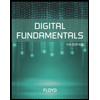
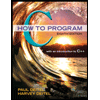
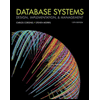
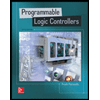