Hello. I'm working on decompressing text in run length encoding in C++. I'm almost finished with the code but I'm confused about how to fix the whitespace. Program requirements: Do not forget to close the file after you are done with it. You must use a while loop to handle the loop reading information from the file. You must use a for loop to handle the count/char output. Use the sample runs to ensure that all output is spelled/spaced correctly. Hint: You will need to make use of .get(ch) to "throw out" the irrelavent spaces. With stdin you would have used std::cin.get(ch), however with file objects remember you will need to use file_obj.get(ch) .
Hello. I'm working on decompressing text in run length encoding in C++. I'm almost finished with the code but I'm confused about how to fix the whitespace.
Program requirements:
- Do not forget to close the file after you are done with it.
- You must use a while loop to handle the loop reading information from the file.
- You must use a for loop to handle the count/char output.
- Use the sample runs to ensure that all output is spelled/spaced correctly.
Hint:
You will need to make use of .get(ch) to "throw out" the irrelavent spaces. With stdin you would have used std::cin.get(ch), however with file objects remember you will need to use file_obj.get(ch) .



Introduction
Decompress:
To decompress is to carry out the opposite of compression. By encoding data in a way that uses fewer bits than the original data, compression is the act of lowering the size of a file or other set of data. The process of undoing this encoding and obtaining the original data from the compressed form is known as decompression.
The size of files is frequently reduced through compression in order to facilitate storage or network transmission, and decompression is required in order to recover the original contents. File types that are frequently compressed include zip archives, JPEG pictures, and MP3 music files.
Step by step
Solved in 3 steps

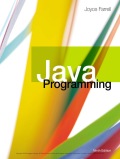
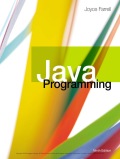