JAVA PROGRAM Chapter 4. Homework Assignment (read instructions carefully) Write a program that asks the user for the name of a file. The program should read all the numbers from the given file and display the total and average of all numbers in the following format (three decimal digits): Total: nnnnn.nnn Average: nnnnn.nnn Class name: FileTotalAndAverage double_input1.txt double_input2.txt PLEASE MODIFY THIS CODE, SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES ALL THE TEST CASES, BECAUSE WHEN I UPLOAD IT TO HYPERGRADE IT DOES NOT PASS THE TEST CASES. IT HAS TO PASS ALL THE TEST CASES. I PROVIDED THE CORRECT OUTPUT AS A SCREENSHOT AS A REFERRENCE. import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; import java.util.InputMismatchException; import java.util.Scanner; public class FileTotalAndAverage { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); String fileName; do { System.out.print("Please enter the file name: "); fileName = scanner.nextLine(); try { double[] numbers = readNumbersFromFile(fileName); if (numbers != null) { double total = calculateTotal(numbers); double average = calculateAverage(numbers); System.out.printf("Total: %.3f\n", total); System.out.printf("Average: %.3f\n", average); break; // Exit the loop if successful } } catch (IOException e) { System.out.println("File '" + fileName + "' does not exist."); } catch (InputMismatchException e) { System.out.println("Invalid data in the file. Please make sure the file contains only numeric values."); } } while (true); scanner.close(); } private static double[] readNumbersFromFile(String fileName) throws IOException { BufferedReader reader = new BufferedReader(new FileReader(fileName)); String line; double[] numbers = null; try { String[] tokens = reader.readLine().split("\\s+"); numbers = new double[tokens.length]; for (int i = 0; i < tokens.length; i++) { numbers[i] = Double.parseDouble(tokens[i]); } } finally { reader.close(); } return numbers; } private static double calculateTotal(double[] numbers) { double total = 0; for (double num : numbers) { total += num; } return total; } private static double calculateAverage(double[] numbers) { double total = calculateTotal(numbers); return total / numbers.length; } } Test Case 1 Please enter the file name: \n double_input1.txtENTER Total: -5,748.583\n Average: -57.486\n Test Case 2 Please enter the file name: \n double_input2.txtENTER Total: 112,546.485\n Average: 56.273\n Test Case 3 Please enter the file name: \n double_input3.txtENTER File 'double_input3.txt' does not exist.\n Please enter the file name again: \n double_input1.txtENTER Total: -5,748.583\n Average: -57.486\n
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.InputMismatchException;
import java.util.Scanner;
public class FileTotalAndAverage {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String fileName;
do {
System.out.print("Please enter the file name: ");
fileName = scanner.nextLine();
try {
double[] numbers = readNumbersFromFile(fileName);
if (numbers != null) {
double total = calculateTotal(numbers);
double average = calculateAverage(numbers);
System.out.printf("Total: %.3f\n", total);
System.out.printf("Average: %.3f\n", average);
break; // Exit the loop if successful
}
} catch (IOException e) {
System.out.println("File '" + fileName + "' does not exist.");
} catch (InputMismatchException e) {
System.out.println("Invalid data in the file. Please make sure the file contains only numeric values.");
}
} while (true);
scanner.close();
}
private static double[] readNumbersFromFile(String fileName) throws IOException {
BufferedReader reader = new BufferedReader(new FileReader(fileName));
String line;
double[] numbers = null;
try {
String[] tokens = reader.readLine().split("\\s+");
numbers = new double[tokens.length];
for (int i = 0; i < tokens.length; i++) {
numbers[i] = Double.parseDouble(tokens[i]);
}
} finally {
reader.close();
}
return numbers;
}
private static double calculateTotal(double[] numbers) {
double total = 0;
for (double num : numbers) {
total += num;
}
return total;
}
private static double calculateAverage(double[] numbers) {
double total = calculateTotal(numbers);
return total / numbers.length;
}
}
Test Case 1
double_input1.txtENTER
Total: -5,748.583\n
Average: -57.486\n
Test Case 2
double_input2.txtENTER
Total: 112,546.485\n
Average: 56.273\n
Test Case 3
double_input3.txtENTER
File 'double_input3.txt' does not exist.\n
Please enter the file name again: \n
double_input1.txtENTER
Total: -5,748.583\n
Average: -57.486\n
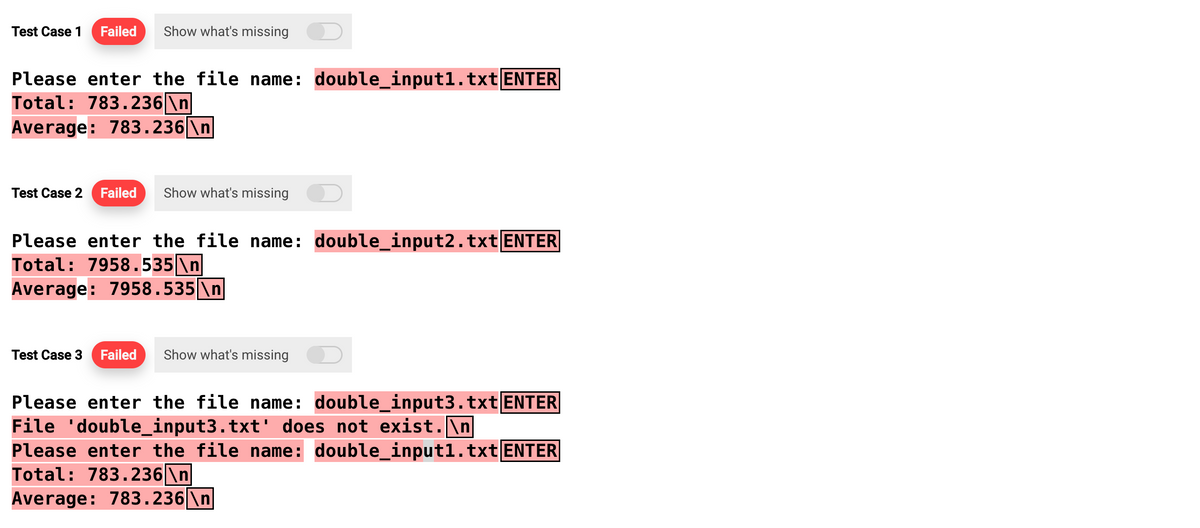

Step by step
Solved in 3 steps with 2 images

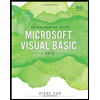
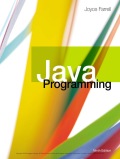
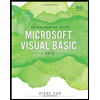
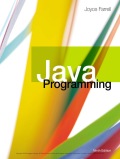