Hello. Please answer the attached C programming question correctly. The attached code has to be changed so that it can print a decoded version of the binary number list. Please do not use very advanced syntax to solve the problem. Also, please create a complete file header based on the file header style picture. *If you change the attached code correctly, do not use very advanced syntax, and create the file header, I will give you a thumbs up. Thanks. —----------------------------------------------------------------------------------------------------------------- Code That Needs to Be Changed: #include int bintodec(char *str) { int x = 0; for(int i = 0; str[i] != '\0'; i++) { x = (str[i] - '0') + x * 2; } return x; } void dectobin(int x, char *bin) { for(int i = 0; i < 7; i++) { bin[i] = '0'; } bin[7] = '\0'; int i = 6; while(x != 0) { bin[i] = (x % 2) + '0'; x /= 2; i--; } } int main() { int num=12; char binaryNum[]="00101100"; char binaryRep[8]; int decRep; int i, j; dectobin(num, binaryRep); decRep = bintodec(binaryNum); printf(" Binary representation of %d is %s.\n",num, binaryRep); printf(" Decimal representation of %s is %d.\n",binaryNum, decRep); return 0; } —------------------------------------------------------------------------------------------------------------------ Question: Assume you received a text message in binary, and you need to decode the message. char *binaryMsg = "0101100101101111011101010010000001100110011011110111010101101110011001000010000001110100011010000110010100100000011100110110010101100011011100100110010101110100001000000110110101100101011100110111001101100001011001110110010100101110"; The message is the ASCII code of some characters. Your task is to convert every eight bits of the message to a decimal number. The decimal number is the ASCII code of the character that you need to print. When you print all characters, you will see the message. *Further instructions are in the pictures.
Hello. Please answer the attached C
*If you change the attached code correctly, do not use very advanced syntax, and create the file header, I will give you a thumbs up. Thanks.
—-----------------------------------------------------------------------------------------------------------------
Code That Needs to Be Changed:
#include <stdio.h>
int bintodec(char *str) {
int x = 0;
for(int i = 0; str[i] != '\0'; i++) {
x = (str[i] - '0') + x * 2;
}
return x;
}
void dectobin(int x, char *bin) {
for(int i = 0; i < 7; i++) {
bin[i] = '0';
}
bin[7] = '\0';
int i = 6;
while(x != 0) {
bin[i] = (x % 2) + '0';
x /= 2;
i--;
}
}
int main()
{
int num=12;
char binaryNum[]="00101100";
char binaryRep[8];
int decRep;
int i, j;
dectobin(num, binaryRep);
decRep = bintodec(binaryNum);
printf(" Binary representation of %d is %s.\n",num, binaryRep);
printf(" Decimal representation of %s is %d.\n",binaryNum, decRep);
return 0;
}
—------------------------------------------------------------------------------------------------------------------
Question:
Assume you received a text message in binary, and you need to decode the message.
char *binaryMsg = "0101100101101111011101010010000001100110011011110111010101101110011001000010000001110100011010000110010100100000011100110110010101100011011100100110010101110100001000000110110101100101011100110111001101100001011001110110010100101110";
The message is the ASCII code of some characters. Your task is to convert every eight bits of the message to a decimal number. The decimal number is the ASCII code of the character that you need to print. When you print all characters, you will see the message.
*Further instructions are in the pictures.
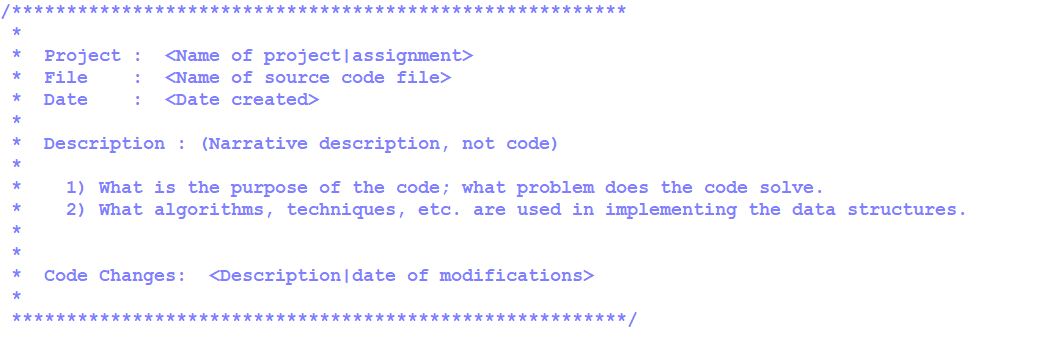
![1. You need to copy the first eight bits of binaryMsg to another string. Then, send that string
for bintodec function and save it in an integer named decRep. Finally, print decRep as a
character.
2. Then continue step 2 for the next eight bits of binaryMsg.
3. Repeat step 2 and 3 till you get to '\0' at the end of binaryMsg.
Hint:
You need a string to send for bintodec function. You can use strncpy to copy a part of
binaryMsg to a temporary string and send it for bintodec function.
char binaryRep [8];
int decRep;
strncpy(binaryRep, binaryMsg + (0*8), 8); //copies the first byte of binaryMSG to binaryRep
decRep = bintodec (binaryRep); // You can print the character value of decRep for the first character o
of the secret message
strncpy(binaryRep, binaryMsg + (1*8), 8); //copies the second byte of binaryMSG to binaryRep
decRep = bintodec (binaryRep); // You can print the character value of decRep for the second character
of the secret message
strncpy(binaryRep, binaryMsg + (2*8), 8); //copies the third byte of binaryMSG to binaryRep
decRep = bintodec (binaryRep); // You can print the character value of decRep for the third character o
of the secret message
you need to repeat this code up to reach '\0' at the end of binary Msg.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1223c4c6-4ebb-4911-bc0d-edb37c26385f%2F4be21743-e771-4f82-97b6-802fa788d6ae%2F3ph155_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

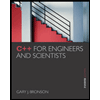
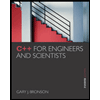