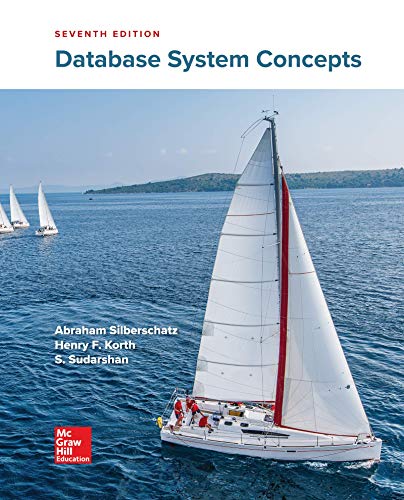
(Class Average: Reading Student Records from a CSV File) Use Python
Use the csv module to read the grades.csv file from the previous exercise (exercise 9.3).
Display the data in tabular format, including an additional column showing each student’s average to the right of that student’s three exam grades and an additional row showing the class average on each exam below that exam’s column.
This is exercise 9.3
# Importing csv module
import csv
# empty list to store data
data = []
columns = ["firstname", "lastname", "grade1", "grade2", "grade3"]
filename = "grades.csv"
for i in range(3):
firstname = input("Enter First Name : ")
lastname = input("Enter Last Name : ")
grade1 = float(input("Enter Grade 1 : "))
grade2 = float(input("Enter Grade 2 : "))
grade3 = float(input("Enter Grade 3 : "))
data.append([firstname, lastname, grade1, grade2, grade3])
print()
# write data and columns as csv file
with open(filename, 'w') as csvfile:
# creating a csv writer object
csvwriter = csv.writer(csvfile)
# writing the columns
csvwriter.writerow(columns)
# writing the each row to the data
csvwriter.writerows(data)
print("CSV file {} has been created successfully!!".format(filename))
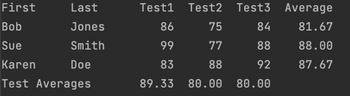

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Create a string variable containing your full name (with spaces) 2) print the string as shown below 3) Create a blank 5x10 character array 4) Create a function printArray() that will print every row and column of the array 5) Create a function getNextCharacter() that gets passed the name string and returns the next non-blank character. If you have reached the end of the string then continue at the beginning. You may need to use a global variable 6) Create a function fillArray() that will fill the 2 dimensional array with the letters of your name skipping blanks. Use the above getNextCharacter() function to fill the array with only the characters in your last name. 7) Print the entire array. 8) Request a row number to print and use a sentinal loop to make sure the row number is valid. If not then request a row number again 9) Create a function printIter() that prints only the passed row using iteration 10) Create a function printRecur() that prints only the passed row using recursion…arrow_forwardC++ Coding: Arrays Implement a two-dimensional character array. Use a nested loop to store a 12x6 flag. 5 x 2 stars as * Alternate = and – to represent stripe colors Use a nested loop to output the character array to the console.arrow_forwardGame of Hunt in C++ language Create the 'Game of Hunt'. The computer ‘hides’ the treasure at a random location in a 10x10 matrix. The user guesses the location by entering a row and column values. The game ends when the user locates the treasure or the treasure value is less than or equal to zero. Guesses in the wrong location will provide clues such as a compass direction or number of squares horizontally or vertically to the treasure. Using the random number generator, display one of the following in the board where the player made their guess: U# Treasure is up ‘#’ on the vertical axis (where # represents an integer number). D# Treasure is down ‘#’ on the vertical axis (where # represents an integer number) || Treasure is in this row, not up or down from the guess location. -> Treasure is to the right. <- Treasure is to the left. -- Treasure is in the same column, not left or right. +$ Adds $50 to treasure and no $50 turn loss. -$ Subtracts…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
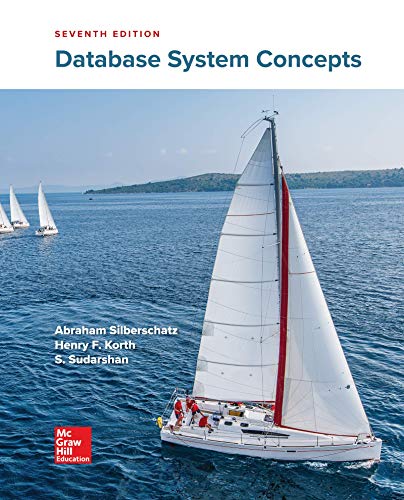
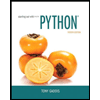
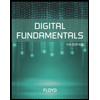
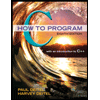
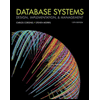
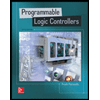