Hi please fix my code so that the output matches this: Course Information: Course Number: ECE287 Course Title: Digital Systems Design Course Information: Course Number: ECE387 Course Title: Embedded Systems Design Instructor Name: Mark Patterson Location: Wilson Hall 231 Class Time: WF: 2-3:30 pm main.cpp (do not change) #include "OfferedCourse.h" int main() { Course myCourse; OfferedCourse myOfferedCourse; string courseNumber, courseTitle; string oCourseNumber, oCourseTitle, instructorName, location, classTime; getline(cin, courseNumber); getline(cin, courseTitle); getline(cin, oCourseNumber); getline(cin, oCourseTitle); getline(cin, instructorName); getline(cin, location); getline(cin, classTime); myCourse.SetCourseNumber(courseNumber); myCourse.SetCourseTitle(courseTitle); myCourse.PrintInfo(); myOfferedCourse.SetCourseNumber(oCourseNumber); myOfferedCourse.SetCourseTitle(oCourseTitle); myOfferedCourse.SetInstructorName(instructorName); myOfferedCourse.SetLocation(location); myOfferedCourse.SetClassTime(classTime); myOfferedCourse.PrintInfo(); cout << " Instructor Name: " << myOfferedCourse.GetInstructorName() << endl; cout << " Location: " << myOfferedCourse.GetLocation() << endl; cout << " Class Time: " << myOfferedCourse.GetClassTime() << endl; } Course.h: #ifndef COURSEH #define COURSEH #include #include using namespace std; class Course { private: string courseNumber; string courseTitle; public: void SetCourseNumber(const string& number); void SetCourseTitle(const string& title); string GetCourseNumber() const; string GetCourseTitle() const; void PrintInfo() const; }; #endif OfferedCourse.h: #ifndef OFFERED_COURSEH #define OFFERED_COURSEH #include "Course.h" #include class OfferedCourse : public Course { private: string instructorName; string location; string classTime; public: void SetInstructorName (const string& instructor); void SetLocation(const string& l); void SetClassTime (const string& time); string GetInstructorName() const; string GetLocation() const; string GetClassTime() const; void PrintInfo() const; }; #endif Course.cpp: #include "Course.h" void Course::SetCourseNumber(const string& number) { courseNumber = number; } void Course::SetCourseTitle(const string& title) { courseTitle = title; } string Course::GetCourseTitle()const { return courseTitle; } string Course::GetCourseNumber()const { return courseNumber; } void Course::PrintInfo() const { cout << "Course Information:"<
Hi please fix my code so that the output matches this:
Course Information:
Course Number: ECE287
Course Title: Digital
Course Information:
Course Number: ECE387
Course Title: Embedded Systems Design Instructor
Name: Mark Patterson
Location: Wilson Hall 231
Class Time: WF: 2-3:30 pm
main.cpp (do not change)
#include "OfferedCourse.h"
int main() {
Course myCourse;
OfferedCourse myOfferedCourse;
string courseNumber, courseTitle;
string oCourseNumber, oCourseTitle, instructorName, location, classTime;
getline(cin, courseNumber);
getline(cin, courseTitle);
getline(cin, oCourseNumber);
getline(cin, oCourseTitle);
getline(cin, instructorName);
getline(cin, location);
getline(cin, classTime);
myCourse.SetCourseNumber(courseNumber);
myCourse.SetCourseTitle(courseTitle);
myCourse.PrintInfo();
myOfferedCourse.SetCourseNumber(oCourseNumber);
myOfferedCourse.SetCourseTitle(oCourseTitle);
myOfferedCourse.SetInstructorName(instructorName);
myOfferedCourse.SetLocation(location);
myOfferedCourse.SetClassTime(classTime);
myOfferedCourse.PrintInfo();
cout << " Instructor Name: " << myOfferedCourse.GetInstructorName() << endl;
cout << " Location: " << myOfferedCourse.GetLocation() << endl;
cout << " Class Time: " << myOfferedCourse.GetClassTime() << endl;
}
Course.h:
#ifndef COURSEH
#define COURSEH
#include <iostream>
#include <string>
using namespace std;
class Course {
private:
string courseNumber;
string courseTitle;
public:
void SetCourseNumber(const string& number);
void SetCourseTitle(const string& title);
string GetCourseNumber() const;
string GetCourseTitle() const;
void PrintInfo() const;
};
#endif
OfferedCourse.h:
#ifndef OFFERED_COURSEH
#define OFFERED_COURSEH
#include "Course.h"
#include <string>
class OfferedCourse : public Course {
private:
string instructorName;
string location;
string classTime;
public:
void SetInstructorName (const string& instructor);
void SetLocation(const string& l);
void SetClassTime (const string& time);
string GetInstructorName() const;
string GetLocation() const;
string GetClassTime() const;
void PrintInfo() const;
};
#endif
Course.cpp:
#include "Course.h"
void Course::SetCourseNumber(const string& number) {
courseNumber = number;
}
void Course::SetCourseTitle(const string& title) {
courseTitle = title;
}
string Course::GetCourseTitle()const {
return courseTitle;
}
string Course::GetCourseNumber()const {
return courseNumber;
}
void Course::PrintInfo() const {
cout << "Course Information:"<<endl;
cout<< "Course Number:"<< GetCourseNumber()<<endl;
cout<<"Course Title:"<<GetCourseTitle()<<endl;
}
OfferedCourse.cpp
#include "OfferedCourse.h"
void OfferedCourse::SetInstructorName(const string& instructor) {
instructorName = instructor;
}
void OfferedCourse::SetLocation(const string& l) {
location = l;
}
void OfferedCourse::SetClassTime(const string& time) {
classTime = time;
}
string OfferedCourse::GetInstructorName() const {
return instructorName;
}
string OfferedCourse::GetLocation() const {
return location;
}
string OfferedCourse::GetClassTime() const {
return classTime;
}
void OfferedCourse::PrintInfo() const {
cout << "Instructor Name: " << GetInstructorName() << endl;
cout << "Location: " << GetLocation() << endl;
cout << "Class Time: " << GetClassTime() << endl;
}
My output comes out to this and I am unsure of how to fix it
Course Information:
Course Number:ECE287
Course Title: Digital Systems Design
Instructor Name: Mark Patterson
Location: Wilson Hall 231
Class Time: WF: 2-3:30 pm
Instructor Name: Mark Patterson
Location: Wilson Hall 231
Class Time: WF: 2-3:30 pm

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

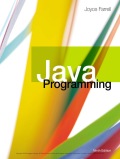
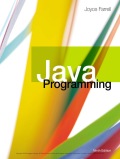