typeder typedef Sequence(); value_type; size_type; // postcondition: The Sequence has been initialized to an empty Sequence. void start(); // postcondition: The first item in the Sequence becomes the current item (but if the // Sequence is empty, then there is no current item). void advance(); // precondition: is_item() returns true // Postcondition: If the current item was already the last item in the Sequence, then there // is no longer any current item. Otherwise, the new current item is the item immediately after // the original current item. void insert (const value_type& entry); // Postcondition: A new copy of entry has been inserted in the Sequence before the // current item. If there was no current item, then the new entry has been inserted at the // front. In either case, the new item is now the current item of the Sequence. void attach (const value_type& entry); // Not required this week. // Postcondition: A new copy of entry has been inserted in the Sequence after the current // item. If there was no current item, then the new entry has been attached to the end of // the Sequence. In either case, the new item is now the current item of the Sequence. void remove_current(); // Not required this week // Precondition: is_item returns true. // Postcondition: The current item has been removed from the Sequence. If the item removed // was the last item in the Sequence, then there is now no current item. Otherwise, the item // that was after the removed item is now the current item. size_type size () const; // Postcondition: Returns the number of items in the Sequence. bool is item() const; // Postcondition: A true return value indicates that there is a valid "current" item that // may be retrieved by the current member function (listed below). A false return value // indicates that there is no valid current item. value_type current() const; // Precondition: is_item() returns true // Postcondition: The current item in the Sequence is returned.
This is the information after the two pictures.
Also, your class should be placed in a namespace "cs_sequence".
Here is an example of a simple client program, to give you an idea about how sequences might be used. You can also use this to test your class, but it's far from exhaustive. Keep in mind as you write your class that you should test each member function after you write it.
int main() { Sequence s; for (int i = 0; i < 6; i++) { s.insert(i); } for (s.start(); s.is_item(); s.advance()) { cout << s.current() << " "; } cout << endl; }
This client program will print the numbers 5 4 3 2 1 0. (They are backward because insert() inserts each new item at the front of the list.)
Implementation
We will be using the following implementation of type Sequence, which includes 5 data members.
- numItems. Stores the number of items in the Sequence.
- headPtr and tailPtr. The head and tail pointers of the linked list. If the Sequence has no items, then these pointers are both nullptr. The reason for the tail pointer is the attach function. Normally this function adds a new item immediately after the current node. But if there is no current node, then attach places its new item at the tail of the list, so it makes sense to keep a tail pointer around.
- cursor. Points to the node with the current item (or nullptr if there is no current item).
- precursor. Points to the node before current item, or nullptr if there is no current item or if the current item is the first node. Note that precursor points to nullptr if there is no current item, or, to put it another way, points to nullptr whenever cursor points to nullptr. Can you figure out why we propose a precursor? The answer is the insert function, which normally adds a new item immediately before the current node. But the linked-list functions have no way of inserting a new node before a speci- fied node. We can only add new nodes after a specified node. Therefore, the insert function will work by adding the new item after the precursor node -- which is also just before the cursor node.
You must use this implementation. That means that you will have 5 data members, you won't have a header node, and precursor must be nullptr if cursor is nullptr. (What is a header node? If you don't know what it is, you probably aren't using it and shouldn't worry about it. It's not the same thing as a headPtr data member, which you WILL be using in this assignment.)
You should implement nodes using the same technique shown in the lecture videos and in the examples in the written lesson. In other words, there should not be a node class; rather, a node struct should be defined inside the Sequence class.
Many students make the mistake of thinking that this assignment will very closely track with the examples done in lecture. In this assignment perhaps more than any other so far I am expecting you to use the concepts taught in the lesson and text to implement a class that is very different from the ones done in the lessons and the text (instead of implementing a class that is pretty similar to the ones done in the lesson and text).
I am providing my solution to the insert() function. This should make it easier to get started: you can use this insert() function and do all of this week's functions (default constructor, size(), start(), advance(), current(), and is_item()) and then write a client program to test what you have so far.
void Sequence::insert(const value_type& entry) { node* new_node = new node; new_node->data = entry; numItems++; if (cursor == headPtr || cursor == nullptr) { // insert at front (or into empty list). new_node->next = headPtr; // precursor remains nullptr. headPtr = new_node; if (numItems == 1) { tailPtr = new_node; } } else { // inserting anywhere else new_node->next = cursor; // tailPtr, headPtr and precursor don't change. precursor->next = new_node; } cursor = new_node; }
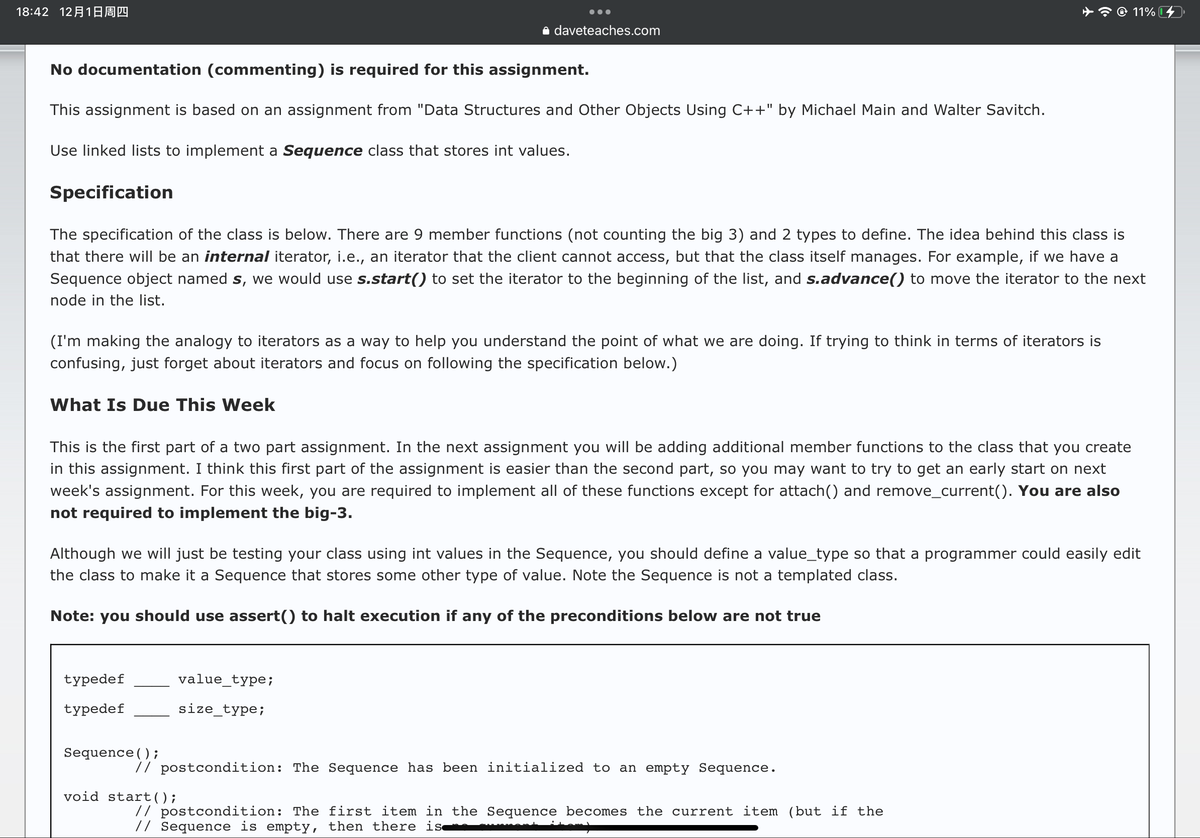
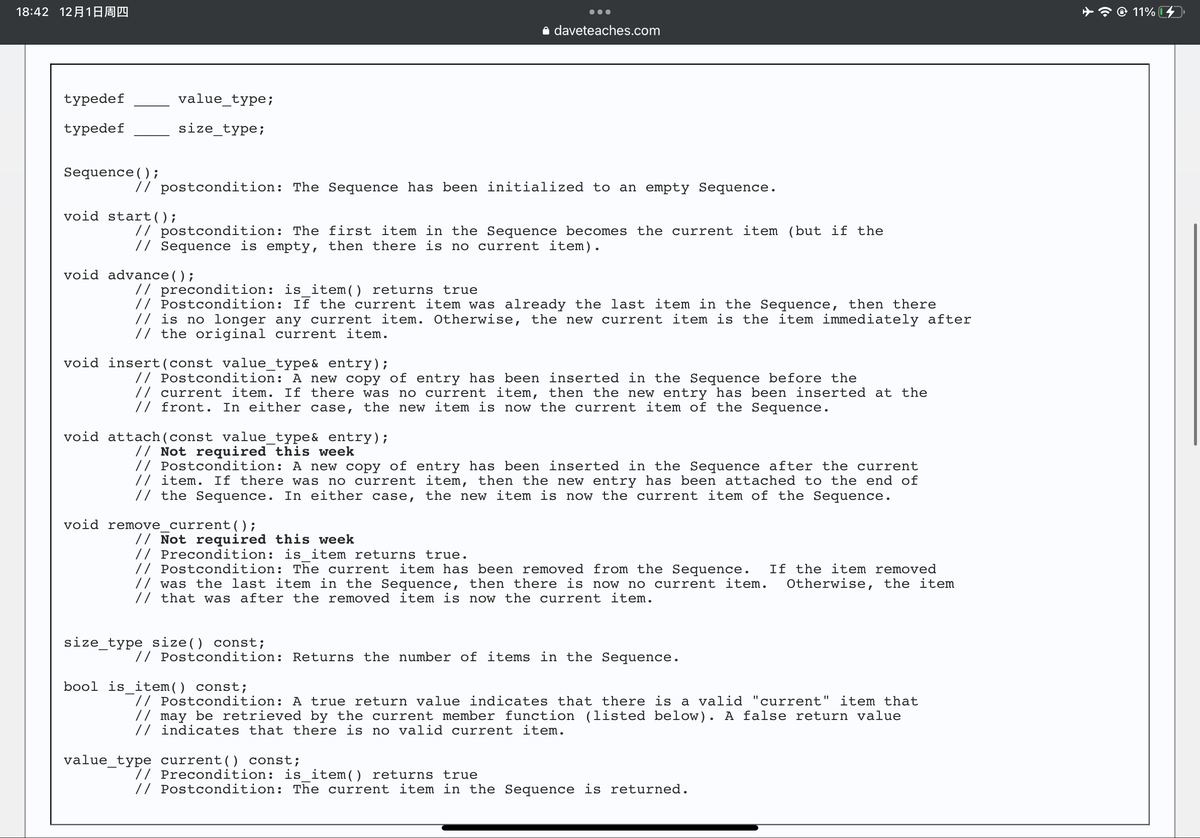

Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 1 images

But this week only need main.cpp, sequence.cpp and sequence.h. Why there are 5 files?
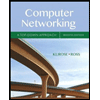
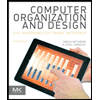
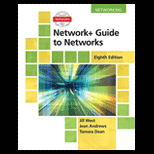
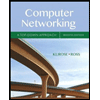
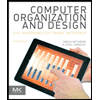
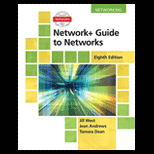
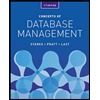
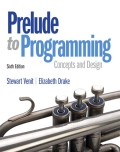
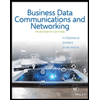