How can I modify this C script so that instead of a single character d or w, it takes the specific string "Delta" or "Wye" as user input to choose either option in the script? I believe the only header that should need to be added is string.h, please don't add anything else I won't be able to use it. My script: #include #include int main(){ //4. Prompt the user for repetition of the process. char ch = 'y'; char network='a'; float za,zb,zc,zac,zbc,zcc,z1,z2,z3,z1c,z2c,z3c; float zaxb,zbxc,zaxc,zd,zdc,z1z3,z1z2,z2z3; float zaxbc,zbxcc,zaxcc,z1z3c,z1z2c,z2z3c,zt,ztc; while(ch=='y' || ch=='Y') { //1. ask the user whether it is a Delta or Wye network. printf("\nWhat kind of network? Enter D for delta or W for Wye network:\n\n"); scanf("%s",&network); //2. ask the user to type in values (for impedances) //3. make the conversion (Delta to Wye or Wye to Delta) //Delta Network ==> Wye if(network=='d'||network=='D'){ printf("\nWhat are the real values of the impedances Za,Zb,and Zc?\n\n"); printf("Za="); scanf("%f",&za); printf("Zb="); scanf("%f",&zb); printf("Zc="); scanf("%f",&zc); printf("\nWhat are the complex values of the impedances Za,Zb,and Zc?\n\n"); printf("Zac="); scanf("%f",&zac); printf("Zbc="); scanf("%f",&zbc); printf("Zcc="); scanf("%f",&zcc); zd=za+zb+zc; zdc=zac+zbc+zcc; zaxc=(za*zc)-(zac*zcc); zaxcc=(za*zcc)+(zc*zac); zaxb=(za*zb)-(zac*zbc); zaxbc=(za*zbc)+(zb*zac); zbxc=(zb*zc)-(zbc*zcc); zbxcc=(zb*zcc)+(zc*zbc); //zaxc/zd z1=((zaxc*zd)+(zaxcc*zdc))/((pow(zd,2)+(pow(zdc,2)))); //zaxb/zd z2=((zaxb*zd)+(zaxbc*zdc))/((pow(zd,2)+(pow(zdc,2)))); //zbxc/zd z3=((zbxc*zd)+(zbxcc*zdc))/((pow(zd,2)+(pow(zdc,2)))); //zaxcc/zdc z1c=((zaxcc*zd)-(zaxc*zdc))/((pow(zd,2)+pow(zdc,2))); //zaxbc/zdc z2c=((zaxbc*zd)-(zaxb*zdc))/((pow(zd,2)+pow(zdc,2))); //zbxcc/zdc z3c=((zaxcc*zd)-(zaxc*zdc))/((pow(zd,2)+pow(zdc,2))); printf("\nDelta values \nZa=%.2f+%.2fj, Zb=%.2f+%.2fj, Zc=%.2f+%.2fj\n\n",za,zac,zb,zbc,zc,zcc); printf("Correspond to Wye values \nZ1=%.2f+%.2fj, Z2=%.2f+%.2fj, Z3=%.2f+%.2fj\n\n",z1,z1c,z2,z2c,z3,z3c); } //Wye Network ==> Delta if(network=='w'||network=='W'){ printf("\nWhat are the real values of the impedances z1,z2,and z3?\n\n"); printf("Z1="); scanf("%f",&z1); printf("Z2="); scanf("%f",&z2); printf("Z3="); scanf("%f",&z3); printf("\nWhat are the complex values of the impedances z1,z2,and z3?\n\n"); printf("Z1c="); scanf("%f",&z1c); printf("Z2c="); scanf("%f",&z2c); printf("Z3c="); scanf("%f",&z3c); z1z2=((z1*z2)-(z1c*z2c)); z1z3=((z1*z3)-(z1c*z3c)); z2z3=((z2*z3)-(z2c*z3c)); z1z2c=((z1*z2c)+(z2*z1c)); z1z3c=((z1*z3c)+(z3*z1c)); z2z3c=((z2*z3c)+(z3*z2c)); zt=(z1z2+z1z3+z2z3); ztc=((z1z2c)+(z1z3c)+(z2z3c)); //za=zt/z3 //zb=zt/z1 //z3=zt/z2 za=(((zt*z3)+(ztc*z3c))/((pow(z3,2)))+(pow(z3c,2))); zb=(((zt*z1)+(ztc*z1c))/((pow(z1,2)))+(pow(z1c,2))); zc=(((zt*z2)+(ztc*z2c))/((pow(z2,2)))+(pow(z2c,2))); //zac=ztc/z3c //zbc=ztc/z1c //z3c=ztc/z2c zac=(((ztc*z3c)-(ztc*z3c))/((pow(z3,2)))+(pow(z3c,2))); zbc=(((ztc*z1c)-(ztc*z1c))/((pow(z1,2)))+(pow(z1c,2))); zcc=(((ztc*z2c)-(ztc*z2c))/((pow(z2,2)))+(pow(z2c,2))); printf("\nWye values \nZ1=%.2f+%.2fj, Z2=%.2f+%.2fj, Z3=%.2f+%.2fj\n\n",z1,z1c,z2,z2c,z3,z3c); printf("Correspond to Delta values \nZa=%.2f+%.2fj, Zb=%.2f+%.2fj, Zc=%.2f+%.2fj\n\n",za,zac,zb,zbc,zc,zcc); } printf("Enter Y to continue, enter any other character to stop the program.\n"); scanf(" %c",&ch); } return 0; }
How can I modify this C script so that instead of a single character d or w, it takes the specific string "Delta" or "Wye" as user input to choose either option in the script?
I believe the only header that should need to be added is string.h, please don't add anything else I won't be able to use it.
My script:
#include<stdio.h>
#include<math.h>
int main(){
//4. Prompt the user for repetition of the process.
char ch = 'y';
char network='a';
float za,zb,zc,zac,zbc,zcc,z1,z2,z3,z1c,z2c,z3c;
float zaxb,zbxc,zaxc,zd,zdc,z1z3,z1z2,z2z3;
float zaxbc,zbxcc,zaxcc,z1z3c,z1z2c,z2z3c,zt,ztc;
while(ch=='y' || ch=='Y')
{
//1. ask the user whether it is a Delta or Wye network.
printf("\nWhat kind of network? Enter D for delta or W for Wye network:\n\n");
scanf("%s",&network);
//2. ask the user to type in values (for impedances)
//3. make the conversion (Delta to Wye or Wye to Delta)
//Delta Network ==> Wye
if(network=='d'||network=='D'){
printf("\nWhat are the real values of the impedances Za,Zb,and Zc?\n\n");
printf("Za=");
scanf("%f",&za);
printf("Zb=");
scanf("%f",&zb);
printf("Zc=");
scanf("%f",&zc);
printf("\nWhat are the complex values of the impedances Za,Zb,and Zc?\n\n");
printf("Zac=");
scanf("%f",&zac);
printf("Zbc=");
scanf("%f",&zbc);
printf("Zcc=");
scanf("%f",&zcc);
zd=za+zb+zc;
zdc=zac+zbc+zcc;
zaxc=(za*zc)-(zac*zcc);
zaxcc=(za*zcc)+(zc*zac);
zaxb=(za*zb)-(zac*zbc);
zaxbc=(za*zbc)+(zb*zac);
zbxc=(zb*zc)-(zbc*zcc);
zbxcc=(zb*zcc)+(zc*zbc);
//zaxc/zd
z1=((zaxc*zd)+(zaxcc*zdc))/((pow(zd,2)+(pow(zdc,2))));
//zaxb/zd
z2=((zaxb*zd)+(zaxbc*zdc))/((pow(zd,2)+(pow(zdc,2))));
//zbxc/zd
z3=((zbxc*zd)+(zbxcc*zdc))/((pow(zd,2)+(pow(zdc,2))));
//zaxcc/zdc
z1c=((zaxcc*zd)-(zaxc*zdc))/((pow(zd,2)+pow(zdc,2)));
//zaxbc/zdc
z2c=((zaxbc*zd)-(zaxb*zdc))/((pow(zd,2)+pow(zdc,2)));
//zbxcc/zdc
z3c=((zaxcc*zd)-(zaxc*zdc))/((pow(zd,2)+pow(zdc,2)));
printf("\nDelta values \nZa=%.2f+%.2fj, Zb=%.2f+%.2fj, Zc=%.2f+%.2fj\n\n",za,zac,zb,zbc,zc,zcc);
printf("Correspond to Wye values \nZ1=%.2f+%.2fj, Z2=%.2f+%.2fj, Z3=%.2f+%.2fj\n\n",z1,z1c,z2,z2c,z3,z3c);
}
//Wye Network ==> Delta
if(network=='w'||network=='W'){
printf("\nWhat are the real values of the impedances z1,z2,and z3?\n\n");
printf("Z1=");
scanf("%f",&z1);
printf("Z2=");
scanf("%f",&z2);
printf("Z3=");
scanf("%f",&z3);
printf("\nWhat are the complex values of the impedances z1,z2,and z3?\n\n");
printf("Z1c=");
scanf("%f",&z1c);
printf("Z2c=");
scanf("%f",&z2c);
printf("Z3c=");
scanf("%f",&z3c);
z1z2=((z1*z2)-(z1c*z2c));
z1z3=((z1*z3)-(z1c*z3c));
z2z3=((z2*z3)-(z2c*z3c));
z1z2c=((z1*z2c)+(z2*z1c));
z1z3c=((z1*z3c)+(z3*z1c));
z2z3c=((z2*z3c)+(z3*z2c));
zt=(z1z2+z1z3+z2z3);
ztc=((z1z2c)+(z1z3c)+(z2z3c));
//za=zt/z3
//zb=zt/z1
//z3=zt/z2
za=(((zt*z3)+(ztc*z3c))/((pow(z3,2)))+(pow(z3c,2)));
zb=(((zt*z1)+(ztc*z1c))/((pow(z1,2)))+(pow(z1c,2)));
zc=(((zt*z2)+(ztc*z2c))/((pow(z2,2)))+(pow(z2c,2)));
//zac=ztc/z3c
//zbc=ztc/z1c
//z3c=ztc/z2c
zac=(((ztc*z3c)-(ztc*z3c))/((pow(z3,2)))+(pow(z3c,2)));
zbc=(((ztc*z1c)-(ztc*z1c))/((pow(z1,2)))+(pow(z1c,2)));
zcc=(((ztc*z2c)-(ztc*z2c))/((pow(z2,2)))+(pow(z2c,2)));
printf("\nWye values \nZ1=%.2f+%.2fj, Z2=%.2f+%.2fj, Z3=%.2f+%.2fj\n\n",z1,z1c,z2,z2c,z3,z3c);
printf("Correspond to Delta values \nZa=%.2f+%.2fj, Zb=%.2f+%.2fj, Zc=%.2f+%.2fj\n\n",za,zac,zb,zbc,zc,zcc);
}
printf("Enter Y to continue, enter any other character to stop the program.\n");
scanf(" %c",&ch);
}
return 0;
}
Thanks!

Step by step
Solved in 3 steps

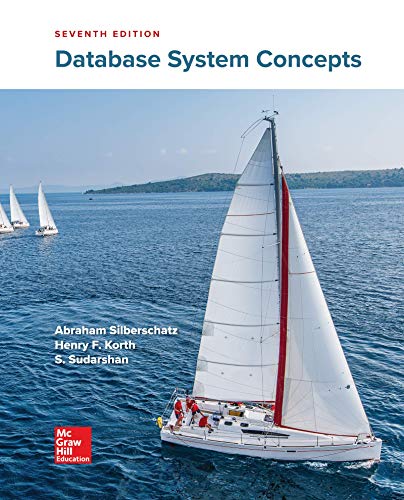
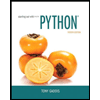
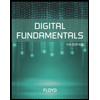
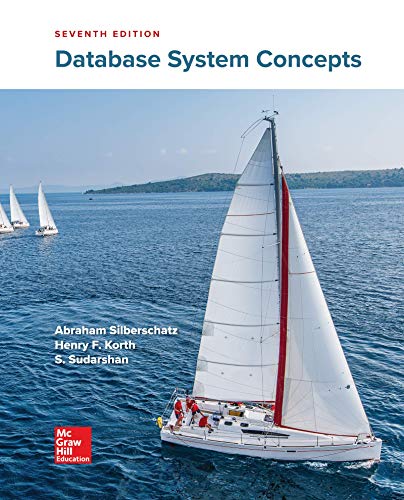
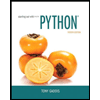
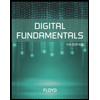
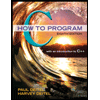
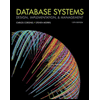
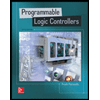