How do you use smart pointers when trying to call a library function that was written before smart pointers were developed? Instead of passing smart pointers to functions, normally, you'll retrieve the smart-pointer's raw pointer using get(), and call the function with that. Complete the code below to double Frank's salary and to print his information. You may use a shared_ptr or a unique_ptr.
How do you use smart pointers when trying to call a library function that was written before smart pointers were developed? Instead of passing smart pointers to functions, normally, you'll retrieve the smart-pointer's raw pointer using get(), and call the function with that. Complete the code below to double Frank's salary and to print his information. You may use a shared_ptr or a unique_ptr.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter10: Pointers
Section: Chapter Questions
Problem 3PP
Related questions
Question
C++
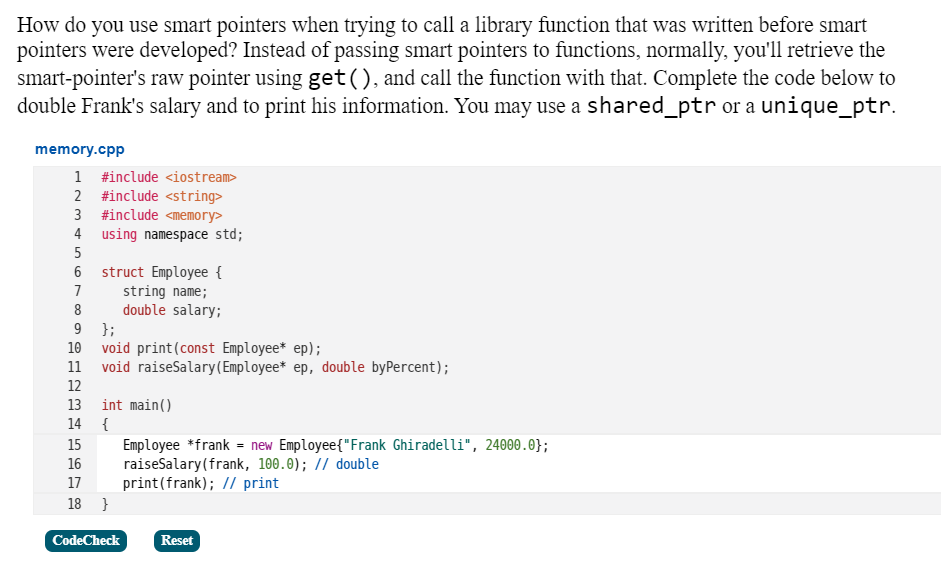
Transcribed Image Text:How do you use smart pointers when trying to call a library function that was written before smart
pointers were developed? Instead of passing smart pointers to functions, normally, you'll retrieve the
smart-pointer's raw pointer using get(), and call the function with that. Complete the code below to
double Frank's salary and to print his information. You may use a shared_ptr or a unique_ptr.
memory.cpp
1 #include <iostream>
2 #include <string>
3 #include <memory>
4 using namespace std;
5
6 struct Employee {
string name;
double salary;
9 };
10 void print(const Employee* ep);
11 void raiseSalary(Employee* ep, double byPercent);
7
8
12
13 int main()
14 {
15
Employee *frank = new Employee{"Frank Ghiradelli", 24000.0};
raiseSalary(frank, 100.0); // double
print(frank); // print
16
17
18 }
CodeCheck
Reset
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
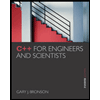
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
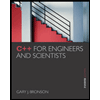
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr